
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
fuzz.c
00001 /* 00002 * Copyright (c) 2001-2003 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Erik Ekman <erik@kryo.se> 00030 * 00031 */ 00032 00033 #include "lwip/init.h" 00034 #include "lwip/netif.h" 00035 #include "netif/etharp.h" 00036 #if LWIP_IPV6 00037 #include "lwip/ethip6.h" 00038 #include "lwip/nd6.h" 00039 #endif 00040 #include <string.h> 00041 #include <stdio.h> 00042 00043 /* no-op send function */ 00044 static err_t lwip_tx_func(struct netif *netif, struct pbuf *p) 00045 { 00046 LWIP_UNUSED_ARG(netif); 00047 LWIP_UNUSED_ARG(p); 00048 return ERR_OK; 00049 } 00050 00051 static err_t testif_init(struct netif *netif) 00052 { 00053 netif->name[0] = 'f'; 00054 netif->name[1] = 'z'; 00055 netif->output = etharp_output; 00056 netif->linkoutput = lwip_tx_func; 00057 netif->mtu = 1500; 00058 netif->hwaddr_len = 6; 00059 netif->flags = NETIF_FLAG_BROADCAST | NETIF_FLAG_ETHARP; 00060 00061 netif->hwaddr[0] = 0x00; 00062 netif->hwaddr[1] = 0x23; 00063 netif->hwaddr[2] = 0xC1; 00064 netif->hwaddr[3] = 0xDE; 00065 netif->hwaddr[4] = 0xD0; 00066 netif->hwaddr[5] = 0x0D; 00067 00068 #if LWIP_IPV6 00069 netif->output_ip6 = ethip6_output; 00070 netif->ip6_autoconfig_enabled = 1; 00071 netif_create_ip6_linklocal_address(netif, 1); 00072 netif->flags |= NETIF_FLAG_MLD6; 00073 #endif 00074 00075 return ERR_OK; 00076 } 00077 00078 static void input_pkt(struct netif *netif, const u8_t *data, size_t len) 00079 { 00080 struct pbuf *p, *q; 00081 err_t err; 00082 00083 LWIP_ASSERT("pkt too big", len <= 0xFFFF); 00084 p = pbuf_alloc(PBUF_RAW, (u16_t)len, PBUF_POOL); 00085 LWIP_ASSERT("alloc failed", p); 00086 for(q = p; q != NULL; q = q->next) { 00087 MEMCPY(q->payload, data, q->len); 00088 data += q->len; 00089 } 00090 err = netif->input(p, netif); 00091 if (err != ERR_OK) { 00092 pbuf_free(p); 00093 } 00094 } 00095 00096 int main(int argc, char** argv) 00097 { 00098 struct netif net_test; 00099 ip4_addr_t addr; 00100 ip4_addr_t netmask; 00101 ip4_addr_t gw; 00102 u8_t pktbuf[2000]; 00103 size_t len; 00104 00105 lwip_init(); 00106 00107 IP4_ADDR(&addr, 172, 30, 115, 84); 00108 IP4_ADDR(&netmask, 255, 255, 255, 0); 00109 IP4_ADDR(&gw, 172, 30, 115, 1); 00110 00111 netif_add(&net_test, &addr, &netmask, &gw, &net_test, testif_init, ethernet_input); 00112 netif_set_up(&net_test); 00113 00114 #if LWIP_IPV6 00115 nd6_tmr(); /* tick nd to join multicast groups */ 00116 #endif 00117 00118 if(argc > 1) { 00119 FILE* f; 00120 const char* filename; 00121 printf("reading input from file... "); 00122 fflush(stdout); 00123 filename = argv[1]; 00124 LWIP_ASSERT("invalid filename", filename != NULL); 00125 f = fopen(filename, "rb"); 00126 LWIP_ASSERT("open failed", f != NULL); 00127 len = fread(pktbuf, 1, sizeof(pktbuf), f); 00128 fclose(f); 00129 printf("testing file: \"%s\"...\r\n", filename); 00130 } else { 00131 len = fread(pktbuf, 1, sizeof(pktbuf), stdin); 00132 } 00133 input_pkt(&net_test, pktbuf, len); 00134 00135 return 0; 00136 }
Generated on Sun Jul 17 2022 08:25:23 by
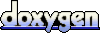