
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
flash_journal_private.h
00001 /* 00002 * Copyright (c) 2006-2016, ARM Limited, All Rights Reserved 00003 * SPDX-License-Identifier: Apache-2.0 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00006 * not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 */ 00017 00018 #ifndef __FLASH_JOURNAL_PRIVATE_H__ 00019 #define __FLASH_JOURNAL_PRIVATE_H__ 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif // __cplusplus 00024 00025 #include "flash-journal/flash_journal.h" 00026 00027 static inline uint32_t roundUp_uint32(uint32_t N, uint32_t BOUNDARY) { 00028 return ((((N) + (BOUNDARY) - 1) / (BOUNDARY)) * (BOUNDARY)); 00029 } 00030 static inline uint32_t roundDown_uint32(uint32_t N, uint32_t BOUNDARY) { 00031 return (((N) / (BOUNDARY)) * (BOUNDARY)); 00032 } 00033 00034 #define LCM_OF_ALL_ERASE_UNITS 4096 /* Assume an LCM of erase_units for now. This will be generalized later. */ 00035 00036 static const uint32_t SEQUENTIAL_FLASH_JOURNAL_INVALD_NEXT_SEQUENCE_NUMBER = 0xFFFFFFFFUL; 00037 static const uint32_t SEQUENTIAL_FLASH_JOURNAL_MAGIC = 0xCE02102AUL; 00038 static const uint32_t SEQUENTIAL_FLASH_JOURNAL_VERSION = 1; 00039 static const uint32_t SEQUENTIAL_FLASH_JOURNAL_HEADER_MAGIC = 0xCEA00AEEUL; 00040 static const uint32_t SEQUENTIAL_FLASH_JOURNAL_HEADER_VERSION = 1; 00041 00042 00043 typedef enum { 00044 SEQUENTIAL_JOURNAL_STATE_NOT_INITIALIZED, 00045 SEQUENTIAL_JOURNAL_STATE_INIT_SCANNING_LOG_HEADERS, 00046 SEQUENTIAL_JOURNAL_STATE_INITIALIZED, 00047 SEQUENTIAL_JOURNAL_STATE_RESETING, 00048 SEQUENTIAL_JOURNAL_STATE_LOGGING_ERASE, 00049 SEQUENTIAL_JOURNAL_STATE_LOGGING_HEAD, 00050 SEQUENTIAL_JOURNAL_STATE_LOGGING_BODY, 00051 SEQUENTIAL_JOURNAL_STATE_LOGGING_TAIL, 00052 SEQUENTIAL_JOURNAL_STATE_READING, 00053 } SequentialFlashJournalState_t; 00054 00055 /** 00056 * Meta-data placed at the head of a Journal. The actual header would be an 00057 * extension of this generic header, and would depend on the implementation 00058 * strategy. Initialization algorithms can expect to find this generic header at 00059 * the start of every Journal. 00060 */ 00061 typedef struct _SequentialFlashJournalHeader { 00062 FlashJournalHeader_t genericHeader; /** Generic meta-data placed at the head of a Journal; common to all journal types. */ 00063 uint32_t magic; /** Sequential journal header specific magic code. */ 00064 uint32_t version; /** Revision number for this sequential journal header. */ 00065 uint32_t numSlots; /** Maximum number of logged blobs; i.e. maximum number of versions of the journaled payload. */ 00066 uint32_t sizeofSlot; /** Slot size. Each slot holds a header, blob-payload, and a tail. */ 00067 } SequentialFlashJournalHeader_t; 00068 00069 /** 00070 * Meta-data placed at the head of a sequential-log entry. 00071 */ 00072 typedef struct _SequentialFlashJournalLogHead { 00073 uint32_t version; 00074 uint32_t magic; 00075 uint32_t sequenceNumber; 00076 uint32_t reserved; 00077 } SequentialFlashJournalLogHead_t; 00078 00079 #define SEQUENTIAL_JOURNAL_VALID_HEAD(PTR) \ 00080 (((PTR)->version == SEQUENTIAL_FLASH_JOURNAL_VERSION) && ((PTR)->magic == SEQUENTIAL_FLASH_JOURNAL_MAGIC)) 00081 00082 /** 00083 * Meta-data placed at the tail of a sequential-log entry. 00084 * 00085 * @note the most crucial items (the ones which play a role in the validation of 00086 * the log-entry) are placed at the end of this structure; this ensures that 00087 * a partially written log-entry-tail won't be accepted as valid. 00088 */ 00089 typedef struct _SequentialFlashJournalLogTail { 00090 uint32_t sizeofBlob; /**< the size of the payload in this blob. */ 00091 uint32_t magic; 00092 uint32_t sequenceNumber; 00093 uint32_t crc32; /**< This field contains the CRC of the header, body (only including logged data), 00094 * and the tail. The 'CRC32' field is assumed to hold 0x0 for the purpose of 00095 * computing the CRC */ 00096 } SequentialFlashJournalLogTail_t; 00097 00098 #define SEQUENTIAL_JOURNAL_VALID_TAIL(TAIL_PTR) ((TAIL_PTR)->magic == SEQUENTIAL_FLASH_JOURNAL_MAGIC) 00099 00100 typedef struct _SequentialFlashJournal_t { 00101 FlashJournal_Ops_t ops; /**< the mandatory OPS table defining the strategy. */ 00102 FlashJournal_Callback_t callback; /**< command completion callback. */ 00103 FlashJournal_Info_t info; /**< the info structure returned from GetInfo(). */ 00104 ARM_DRIVER_STORAGE *mtd; /**< The underlying Memory-Technology-Device. */ 00105 ARM_STORAGE_CAPABILITIES mtdCapabilities; /**< the return from mtd->GetCapabilities(); held for quick reference. */ 00106 uint64_t mtdStartOffset; /**< the start of the address range maintained by the underlying MTD. */ 00107 uint32_t firstSlotOffset; /** Offset from the start of the journal header to the actual logged journal. */ 00108 uint32_t numSlots; /** Maximum number of logged blobs; i.e. maximum number of versions of the journaled payload. */ 00109 uint32_t sizeofSlot; /**< size of the log stride. */ 00110 uint32_t nextSequenceNumber; /**< the next valid sequence number to be used when logging the next blob. */ 00111 uint32_t currentBlobIndex; /**< index of the most recently written blob. */ 00112 SequentialFlashJournalState_t state; /**< state of the journal. SEQUENTIAL_JOURNAL_STATE_INITIALIZED being the default. */ 00113 FlashJournal_OpCode_t prevCommand; /**< the last command issued to the journal. */ 00114 00115 /** 00116 * The following is a union of sub-structures meant to keep state relevant 00117 * to the commands during their execution. 00118 */ 00119 union { 00120 /** state relevant to initialization. */ 00121 struct { 00122 uint64_t currentOffset; 00123 struct { 00124 uint32_t headSequenceNumber; 00125 SequentialFlashJournalLogTail_t tail; 00126 }; 00127 } initScan; 00128 00129 /** state relevant to logging of data. */ 00130 struct { 00131 const uint8_t *blob; /**< the original buffer holding source data. */ 00132 size_t sizeofBlob; 00133 union { 00134 struct { 00135 uint64_t mtdEraseOffset; 00136 }; 00137 struct { 00138 uint64_t mtdOffset; /**< the current Storage offset at which data will be written. */ 00139 uint64_t mtdTailOffset; /**< Storage offset at which the SequentialFlashJournalLogTail_t will be logged for this log-entry. */ 00140 const uint8_t *dataBeingLogged; /**< temporary pointer aimed at the next data to be logged. */ 00141 size_t amountLeftToLog; 00142 union { 00143 SequentialFlashJournalLogHead_t head; 00144 SequentialFlashJournalLogTail_t tail; 00145 }; 00146 }; 00147 }; 00148 } log; 00149 00150 /** state relevant to read-back of data. */ 00151 struct { 00152 const uint8_t *blob; /**< the original buffer holding source data. */ 00153 size_t sizeofBlob; 00154 uint64_t mtdOffset; /**< the current Storage offset from which data is being read. */ 00155 uint8_t *dataBeingRead; /**< temporary pointer aimed at the next data to be read-into. */ 00156 size_t amountLeftToRead; 00157 size_t logicalOffset; /**< the logical offset within the blob at which the next read will occur. */ 00158 } read; 00159 }; 00160 } SequentialFlashJournal_t; 00161 00162 /**< 00163 * A static assert to ensure that the size of SequentialJournal is smaller than 00164 * FlashJournal_t. The caller will only allocate a FlashJournal_t and expect the 00165 * Sequential Strategy to reuse that space for a SequentialFlashJournal_t. 00166 */ 00167 typedef char AssertSequentialJournalSizeLessThanOrEqualToGenericJournal[sizeof(SequentialFlashJournal_t)<=sizeof(FlashJournal_t)?1:-1]; 00168 00169 #define SLOT_ADDRESS(JOURNAL, INDEX) ((JOURNAL)->mtdStartOffset + (JOURNAL)->firstSlotOffset + ((INDEX) * (JOURNAL)->sizeofSlot)) 00170 00171 #ifdef __cplusplus 00172 } 00173 #endif // __cplusplus 00174 00175 #endif /* __FLASH_JOURNAL_PRIVATE_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
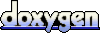