
Rtos API example
fhss_api.h File Reference
Go to the source code of this file.
Data Structures | |
struct | fhss_api |
Struct fhss_api defines interface between software MAC and FHSS. More... | |
struct | fhss_callback |
Struct fhss_callback defines functions that software MAC needs to implement. More... | |
Typedefs | |
typedef bool | fhss_is_broadcast_channel (const fhss_api_t *api) |
FHSS is broadcast channel. | |
typedef bool | fhss_use_broadcast_queue (const fhss_api_t *api, bool is_broadcast_addr, int frame_type) |
FHSS queue check. | |
typedef int | fhss_tx_handle (const fhss_api_t *api, bool is_broadcast_addr, uint8_t *destination_address, int frame_type, uint8_t *synch_info, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length) |
FHSS TX handle. | |
typedef bool | fhss_check_tx_conditions (const fhss_api_t *api, bool is_broadcast_addr, uint8_t handle, int frame_type, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length) |
Check TX permission. | |
typedef void | fhss_receive_frame (const fhss_api_t *api, uint16_t pan_id, uint8_t *source_address, uint32_t timestamp, uint8_t *synch_info, int frame_type) |
Notification of received FHSS synch or synch request frame. | |
typedef void | fhss_data_tx_done (const fhss_api_t *api, bool waiting_ack, bool tx_completed, uint8_t handle) |
Data TX done callback. | |
typedef bool | fhss_data_tx_fail (const fhss_api_t *api, uint8_t handle) |
Data TX or CCA failed callback. | |
typedef void | fhss_synch_state_set (const fhss_api_t *api, fhss_states fhss_state, uint16_t pan_id) |
Change synchronization state. | |
typedef uint32_t | fhss_read_timestamp (const fhss_api_t *api) |
Read timestamp. | |
typedef uint16_t | fhss_get_retry_period (const fhss_api_t *api, uint8_t *destination_address, uint16_t phy_mtu) |
Get retransmission period. | |
typedef int | fhss_init_callbacks (const fhss_api_t *api, fhss_callback_t *callbacks) |
Initialize MAC functions. | |
typedef uint16_t | mac_read_tx_queue_size (const fhss_api_t *fhss_api, bool broadcast_queue) |
Read MAC TX queue size. | |
typedef int | mac_read_mac_address (const fhss_api_t *fhss_api, uint8_t *mac_address) |
Read MAC address. | |
typedef uint32_t | mac_read_datarate (const fhss_api_t *fhss_api) |
Read PHY datarate. | |
typedef int | mac_change_channel (const fhss_api_t *fhss_api, uint8_t channel_number) |
Change channel. | |
typedef int | mac_send_fhss_frame (const fhss_api_t *fhss_api, int frame_type) |
Send FHSS frame. | |
typedef int | mac_synch_lost_notification (const fhss_api_t *fhss_api) |
Send notification when FHSS synchronization is lost. | |
typedef int | mac_tx_poll (const fhss_api_t *fhss_api) |
Poll TX queue. | |
typedef int | mac_broadcast_notify (const fhss_api_t *fhss_api, uint32_t broadcast_time) |
Broadcast channel notification from FHSS. | |
typedef int | mac_read_coordinator_mac_address (const fhss_api_t *fhss_api, uint8_t *mac_address) |
Read coordinator MAC address. | |
Enumerations | |
enum | fhss_states |
FHSS states. More... |
Detailed Description
Definition in file fhss_api.h.
Typedef Documentation
typedef bool fhss_check_tx_conditions(const fhss_api_t *api, bool is_broadcast_addr, uint8_t handle, int frame_type, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length) |
Check TX permission.
- Parameters:
-
api FHSS instance. is_broadcast_addr Destination address type of packet (true if broadcast address). handle Handle of the data request. frame_type Frame type of packet (Frames types are defined by FHSS api). frame_length MSDU length of the frame. phy_header_length PHY header length. phy_tail_length PHY tail length.
- Returns:
- false if transmission is denied, true if transmission is allowed.
Definition at line 97 of file fhss_api.h.
typedef void fhss_data_tx_done(const fhss_api_t *api, bool waiting_ack, bool tx_completed, uint8_t handle) |
Data TX done callback.
- Parameters:
-
api FHSS instance. waiting_ack MAC is waiting Acknowledgement for this frame. tx_completed TX completed (Ack received or no more retries left (if unicast frame)). handle Handle of the data request.
Definition at line 117 of file fhss_api.h.
typedef bool fhss_data_tx_fail(const fhss_api_t *api, uint8_t handle) |
Data TX or CCA failed callback.
- Parameters:
-
api FHSS instance. handle Handle of the data request.
- Returns:
- true if frame has to be queued for retransmission, false otherwise.
Definition at line 125 of file fhss_api.h.
typedef uint16_t fhss_get_retry_period(const fhss_api_t *api, uint8_t *destination_address, uint16_t phy_mtu) |
Get retransmission period.
FHSS uses different retry periods for different destinations.
- Parameters:
-
api FHSS instance. destination_address Destination MAC address. phy_mtu PHY MTU size.
- Returns:
- Retransmission period.
Definition at line 149 of file fhss_api.h.
typedef int fhss_init_callbacks(const fhss_api_t *api, fhss_callback_t *callbacks) |
Initialize MAC functions.
- Parameters:
-
api FHSS instance. callbacks MAC functions to be called from FHSS.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 158 of file fhss_api.h.
typedef bool fhss_is_broadcast_channel(const fhss_api_t *api) |
FHSS is broadcast channel.
Checks if current channel is broadcast channel.
- Parameters:
-
api FHSS instance.
- Returns:
- false if unicast channel, true if broadcast channel.
Definition at line 57 of file fhss_api.h.
typedef uint32_t fhss_read_timestamp(const fhss_api_t *api) |
Read timestamp.
- Parameters:
-
api FHSS instance.
- Returns:
- Timestamp to be written in received frame.
Definition at line 140 of file fhss_api.h.
typedef void fhss_receive_frame(const fhss_api_t *api, uint16_t pan_id, uint8_t *source_address, uint32_t timestamp, uint8_t *synch_info, int frame_type) |
Notification of received FHSS synch or synch request frame.
- Parameters:
-
api FHSS instance. pan_id Source PAN id of the received frame (FHSS_SYNCH_FRAME only). source_address Source address of the received frame (FHSS_SYNCH_FRAME only). timestamp Timestamp of reception (FHSS_SYNCH_FRAME only). synch_info Pointer to synchronization info (FHSS_SYNCH_FRAME only). frame_type Frame type of packet (Frames types are defined by FHSS api).
Definition at line 108 of file fhss_api.h.
typedef void fhss_synch_state_set(const fhss_api_t *api, fhss_states fhss_state, uint16_t pan_id) |
Change synchronization state.
- Parameters:
-
api FHSS instance. fhss_state FHSS state (FHSS states are defined by FHSS api). pan_id PAN id of the network FHSS synchronizes with.
Definition at line 133 of file fhss_api.h.
typedef int fhss_tx_handle(const fhss_api_t *api, bool is_broadcast_addr, uint8_t *destination_address, int frame_type, uint8_t *synch_info, uint16_t frame_length, uint8_t phy_header_length, uint8_t phy_tail_length) |
FHSS TX handle.
Set destination channel and write synchronization info.
- Parameters:
-
api FHSS instance. is_broadcast_addr Destination address type of packet (true if broadcast address). destination_address Destination MAC address. frame_type Frame type of packet (Frames types are defined by FHSS api). synch_info Pointer to where FHSS synchronization info is written (if synch frame). frame_length MSDU length of the frame. phy_header_length PHY header length. phy_tail_length PHY tail length.
- Returns:
- 0 Success.
- -1 Transmission of the packet is currently not allowed, try again.
- -2 Invalid api.
- -3 Broadcast packet on Unicast channel (not allowed), push packet back to queue.
- -4 Synchronization info missing.
Definition at line 84 of file fhss_api.h.
typedef bool fhss_use_broadcast_queue(const fhss_api_t *api, bool is_broadcast_addr, int frame_type) |
FHSS queue check.
Checks if broadcast queue must be used instead of unicast queue.
- Parameters:
-
api FHSS instance. is_broadcast_addr Destination address type of packet (true if broadcast address). frame_type Frame type of packet (Frames types are defined by FHSS api).
- Returns:
- false if unicast queue, true if broadcast queue.
Definition at line 66 of file fhss_api.h.
typedef int mac_broadcast_notify(const fhss_api_t *fhss_api, uint32_t broadcast_time) |
Broadcast channel notification from FHSS.
- Parameters:
-
fhss_api FHSS instance. broadcast_time Remaining broadcast time.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 244 of file fhss_api.h.
typedef int mac_change_channel(const fhss_api_t *fhss_api, uint8_t channel_number) |
Change channel.
- Parameters:
-
fhss_api FHSS instance. channel_number Channel number.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 210 of file fhss_api.h.
typedef int mac_read_coordinator_mac_address(const fhss_api_t *fhss_api, uint8_t *mac_address) |
Read coordinator MAC address.
- Parameters:
-
fhss_api FHSS instance. mac_address MAC address pointer.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 253 of file fhss_api.h.
typedef uint32_t mac_read_datarate(const fhss_api_t *fhss_api) |
Read PHY datarate.
- Parameters:
-
fhss_api FHSS instance.
- Returns:
- PHY datarate.
Definition at line 201 of file fhss_api.h.
typedef int mac_read_mac_address(const fhss_api_t *fhss_api, uint8_t *mac_address) |
Read MAC address.
- Parameters:
-
fhss_api FHSS instance. mac_address MAC address pointer.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 194 of file fhss_api.h.
typedef uint16_t mac_read_tx_queue_size(const fhss_api_t *fhss_api, bool broadcast_queue) |
Read MAC TX queue size.
- Parameters:
-
fhss_api FHSS instance. broadcast_queue Queue type to be read (true if broadcast queue).
- Returns:
- Queue size (number of frames queued).
Definition at line 185 of file fhss_api.h.
typedef int mac_send_fhss_frame(const fhss_api_t *fhss_api, int frame_type) |
Send FHSS frame.
- Parameters:
-
fhss_api FHSS instance. frame_type Frame type of packet (Frames types are defined by FHSS api).
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 219 of file fhss_api.h.
typedef int mac_synch_lost_notification(const fhss_api_t *fhss_api) |
Send notification when FHSS synchronization is lost.
- Parameters:
-
fhss_api FHSS instance.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 227 of file fhss_api.h.
typedef int mac_tx_poll(const fhss_api_t *fhss_api) |
Poll TX queue.
- Parameters:
-
fhss_api FHSS instance.
- Returns:
- 0 Success.
- -1 Invalid parameters.
Definition at line 235 of file fhss_api.h.
Enumeration Type Documentation
enum fhss_states |
FHSS states.
Definition at line 46 of file fhss_api.h.
Generated on Sun Jul 17 2022 08:25:34 by
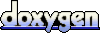