
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
entropy.h
Go to the documentation of this file.
00001 /** 00002 * \file entropy.h 00003 * 00004 * \brief Entropy accumulator implementation 00005 * 00006 * Copyright (C) 2006-2016, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ENTROPY_H 00024 #define MBEDTLS_ENTROPY_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 00034 #if defined(MBEDTLS_SHA512_C) && !defined(MBEDTLS_ENTROPY_FORCE_SHA256) 00035 #include "sha512.h" 00036 #define MBEDTLS_ENTROPY_SHA512_ACCUMULATOR 00037 #else 00038 #if defined(MBEDTLS_SHA256_C) 00039 #define MBEDTLS_ENTROPY_SHA256_ACCUMULATOR 00040 #include "sha256.h" 00041 #endif 00042 #endif 00043 00044 #if defined(MBEDTLS_THREADING_C) 00045 #include "threading.h" 00046 #endif 00047 00048 #if defined(MBEDTLS_HAVEGE_C) 00049 #include "havege.h" 00050 #endif 00051 00052 #define MBEDTLS_ERR_ENTROPY_SOURCE_FAILED -0x003C /**< Critical entropy source failure. */ 00053 #define MBEDTLS_ERR_ENTROPY_MAX_SOURCES -0x003E /**< No more sources can be added. */ 00054 #define MBEDTLS_ERR_ENTROPY_NO_SOURCES_DEFINED -0x0040 /**< No sources have been added to poll. */ 00055 #define MBEDTLS_ERR_ENTROPY_NO_STRONG_SOURCE -0x003D /**< No strong sources have been added to poll. */ 00056 #define MBEDTLS_ERR_ENTROPY_FILE_IO_ERROR -0x003F /**< Read/write error in file. */ 00057 00058 /** 00059 * \name SECTION: Module settings 00060 * 00061 * The configuration options you can set for this module are in this section. 00062 * Either change them in config.h or define them on the compiler command line. 00063 * \{ 00064 */ 00065 00066 #if !defined(MBEDTLS_ENTROPY_MAX_SOURCES) 00067 #define MBEDTLS_ENTROPY_MAX_SOURCES 20 /**< Maximum number of sources supported */ 00068 #endif 00069 00070 #if !defined(MBEDTLS_ENTROPY_MAX_GATHER) 00071 #define MBEDTLS_ENTROPY_MAX_GATHER 128 /**< Maximum amount requested from entropy sources */ 00072 #endif 00073 00074 /* \} name SECTION: Module settings */ 00075 00076 #if defined(MBEDTLS_ENTROPY_SHA512_ACCUMULATOR) 00077 #define MBEDTLS_ENTROPY_BLOCK_SIZE 64 /**< Block size of entropy accumulator (SHA-512) */ 00078 #else 00079 #define MBEDTLS_ENTROPY_BLOCK_SIZE 32 /**< Block size of entropy accumulator (SHA-256) */ 00080 #endif 00081 00082 #define MBEDTLS_ENTROPY_MAX_SEED_SIZE 1024 /**< Maximum size of seed we read from seed file */ 00083 #define MBEDTLS_ENTROPY_SOURCE_MANUAL MBEDTLS_ENTROPY_MAX_SOURCES 00084 00085 #define MBEDTLS_ENTROPY_SOURCE_STRONG 1 /**< Entropy source is strong */ 00086 #define MBEDTLS_ENTROPY_SOURCE_WEAK 0 /**< Entropy source is weak */ 00087 00088 #ifdef __cplusplus 00089 extern "C" { 00090 #endif 00091 00092 /** 00093 * \brief Entropy poll callback pointer 00094 * 00095 * \param data Callback-specific data pointer 00096 * \param output Data to fill 00097 * \param len Maximum size to provide 00098 * \param olen The actual amount of bytes put into the buffer (Can be 0) 00099 * 00100 * \return 0 if no critical failures occurred, 00101 * MBEDTLS_ERR_ENTROPY_SOURCE_FAILED otherwise 00102 */ 00103 typedef int (*mbedtls_entropy_f_source_ptr)(void *data, unsigned char *output, size_t len, 00104 size_t *olen); 00105 00106 /** 00107 * \brief Entropy source state 00108 */ 00109 typedef struct 00110 { 00111 mbedtls_entropy_f_source_ptr f_source; /**< The entropy source callback */ 00112 void * p_source; /**< The callback data pointer */ 00113 size_t size; /**< Amount received in bytes */ 00114 size_t threshold; /**< Minimum bytes required before release */ 00115 int strong; /**< Is the source strong? */ 00116 } 00117 mbedtls_entropy_source_state; 00118 00119 /** 00120 * \brief Entropy context structure 00121 */ 00122 typedef struct 00123 { 00124 #if defined(MBEDTLS_ENTROPY_SHA512_ACCUMULATOR) 00125 mbedtls_sha512_context accumulator; 00126 #else 00127 mbedtls_sha256_context accumulator; 00128 #endif 00129 int source_count; 00130 mbedtls_entropy_source_state source[MBEDTLS_ENTROPY_MAX_SOURCES]; 00131 #if defined(MBEDTLS_HAVEGE_C) 00132 mbedtls_havege_state havege_data; 00133 #endif 00134 #if defined(MBEDTLS_THREADING_C) 00135 mbedtls_threading_mutex_t mutex ; /*!< mutex */ 00136 #endif 00137 #if defined(MBEDTLS_ENTROPY_NV_SEED) 00138 int initial_entropy_run; 00139 #endif 00140 } 00141 mbedtls_entropy_context; 00142 00143 /** 00144 * \brief Initialize the context 00145 * 00146 * \param ctx Entropy context to initialize 00147 */ 00148 void mbedtls_entropy_init( mbedtls_entropy_context *ctx ); 00149 00150 /** 00151 * \brief Free the data in the context 00152 * 00153 * \param ctx Entropy context to free 00154 */ 00155 void mbedtls_entropy_free( mbedtls_entropy_context *ctx ); 00156 00157 /** 00158 * \brief Adds an entropy source to poll 00159 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00160 * 00161 * \param ctx Entropy context 00162 * \param f_source Entropy function 00163 * \param p_source Function data 00164 * \param threshold Minimum required from source before entropy is released 00165 * ( with mbedtls_entropy_func() ) (in bytes) 00166 * \param strong MBEDTLS_ENTROPY_SOURCE_STRONG or 00167 * MBEDTSL_ENTROPY_SOURCE_WEAK. 00168 * At least one strong source needs to be added. 00169 * Weaker sources (such as the cycle counter) can be used as 00170 * a complement. 00171 * 00172 * \return 0 if successful or MBEDTLS_ERR_ENTROPY_MAX_SOURCES 00173 */ 00174 int mbedtls_entropy_add_source( mbedtls_entropy_context *ctx, 00175 mbedtls_entropy_f_source_ptr f_source, void *p_source, 00176 size_t threshold, int strong ); 00177 00178 /** 00179 * \brief Trigger an extra gather poll for the accumulator 00180 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00181 * 00182 * \param ctx Entropy context 00183 * 00184 * \return 0 if successful, or MBEDTLS_ERR_ENTROPY_SOURCE_FAILED 00185 */ 00186 int mbedtls_entropy_gather( mbedtls_entropy_context *ctx ); 00187 00188 /** 00189 * \brief Retrieve entropy from the accumulator 00190 * (Maximum length: MBEDTLS_ENTROPY_BLOCK_SIZE) 00191 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00192 * 00193 * \param data Entropy context 00194 * \param output Buffer to fill 00195 * \param len Number of bytes desired, must be at most MBEDTLS_ENTROPY_BLOCK_SIZE 00196 * 00197 * \return 0 if successful, or MBEDTLS_ERR_ENTROPY_SOURCE_FAILED 00198 */ 00199 int mbedtls_entropy_func( void *data, unsigned char *output, size_t len ); 00200 00201 /** 00202 * \brief Add data to the accumulator manually 00203 * (Thread-safe if MBEDTLS_THREADING_C is enabled) 00204 * 00205 * \param ctx Entropy context 00206 * \param data Data to add 00207 * \param len Length of data 00208 * 00209 * \return 0 if successful 00210 */ 00211 int mbedtls_entropy_update_manual( mbedtls_entropy_context *ctx, 00212 const unsigned char *data, size_t len ); 00213 00214 #if defined(MBEDTLS_ENTROPY_NV_SEED) 00215 /** 00216 * \brief Trigger an update of the seed file in NV by using the 00217 * current entropy pool. 00218 * 00219 * \param ctx Entropy context 00220 * 00221 * \return 0 if successful 00222 */ 00223 int mbedtls_entropy_update_nv_seed( mbedtls_entropy_context *ctx ); 00224 #endif /* MBEDTLS_ENTROPY_NV_SEED */ 00225 00226 #if defined(MBEDTLS_FS_IO) 00227 /** 00228 * \brief Write a seed file 00229 * 00230 * \param ctx Entropy context 00231 * \param path Name of the file 00232 * 00233 * \return 0 if successful, 00234 * MBEDTLS_ERR_ENTROPY_FILE_IO_ERROR on file error, or 00235 * MBEDTLS_ERR_ENTROPY_SOURCE_FAILED 00236 */ 00237 int mbedtls_entropy_write_seed_file( mbedtls_entropy_context *ctx, const char *path ); 00238 00239 /** 00240 * \brief Read and update a seed file. Seed is added to this 00241 * instance. No more than MBEDTLS_ENTROPY_MAX_SEED_SIZE bytes are 00242 * read from the seed file. The rest is ignored. 00243 * 00244 * \param ctx Entropy context 00245 * \param path Name of the file 00246 * 00247 * \return 0 if successful, 00248 * MBEDTLS_ERR_ENTROPY_FILE_IO_ERROR on file error, 00249 * MBEDTLS_ERR_ENTROPY_SOURCE_FAILED 00250 */ 00251 int mbedtls_entropy_update_seed_file( mbedtls_entropy_context *ctx, const char *path ); 00252 #endif /* MBEDTLS_FS_IO */ 00253 00254 #if defined(MBEDTLS_SELF_TEST) 00255 /** 00256 * \brief Checkup routine 00257 * 00258 * This module self-test also calls the entropy self-test, 00259 * mbedtls_entropy_source_self_test(); 00260 * 00261 * \return 0 if successful, or 1 if a test failed 00262 */ 00263 int mbedtls_entropy_self_test( int verbose ); 00264 00265 #if defined(MBEDTLS_ENTROPY_HARDWARE_ALT) 00266 /** 00267 * \brief Checkup routine 00268 * 00269 * Verifies the integrity of the hardware entropy source 00270 * provided by the function 'mbedtls_hardware_poll()'. 00271 * 00272 * Note this is the only hardware entropy source that is known 00273 * at link time, and other entropy sources configured 00274 * dynamically at runtime by the function 00275 * mbedtls_entropy_add_source() will not be tested. 00276 * 00277 * \return 0 if successful, or 1 if a test failed 00278 */ 00279 int mbedtls_entropy_source_self_test( int verbose ); 00280 #endif /* MBEDTLS_ENTROPY_HARDWARE_ALT */ 00281 #endif /* MBEDTLS_SELF_TEST */ 00282 00283 #ifdef __cplusplus 00284 } 00285 #endif 00286 00287 #endif /* entropy.h */
Generated on Sun Jul 17 2022 08:25:22 by
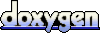