
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
emac_lwip.c
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #if DEVICE_EMAC 00018 00019 #include "emac_api.h" 00020 #include "emac_stack_mem.h" 00021 #include "lwip/tcpip.h" 00022 #include "lwip/tcp.h" 00023 #include "lwip/ip.h" 00024 #include "netif/etharp.h" 00025 00026 static err_t emac_lwip_low_level_output(struct netif *netif, struct pbuf *p) 00027 { 00028 emac_interface_t *mac = (emac_interface_t *)netif->state; 00029 bool ret = mac->ops.link_out(mac, (emac_stack_mem_t *)p); 00030 00031 return ret ? ERR_OK : ERR_IF; 00032 } 00033 00034 static void emac_lwip_input(void *data, emac_stack_t *buf) 00035 { 00036 struct pbuf *p = (struct pbuf *)buf; 00037 struct netif *netif = (struct netif *)data; 00038 00039 /* pass all packets to ethernet_input, which decides what packets it supports */ 00040 if (netif->input(p, netif) != ERR_OK) { 00041 LWIP_DEBUGF(NETIF_DEBUG, ("Emac LWIP: IP input error\n")); 00042 00043 pbuf_free(p); 00044 } 00045 } 00046 00047 static void emac_lwip_state_change(void *data, bool up) 00048 { 00049 struct netif *netif = (struct netif *)data; 00050 00051 if (up) { 00052 tcpip_callback_with_block((tcpip_callback_fn)netif_set_link_up, netif, 1); 00053 } else { 00054 tcpip_callback_with_block((tcpip_callback_fn)netif_set_link_down, netif, 1); 00055 } 00056 } 00057 00058 err_t emac_lwip_if_init(struct netif *netif) 00059 { 00060 int err = ERR_OK; 00061 emac_interface_t *mac = (emac_interface_t *)netif->state; 00062 00063 mac->ops.set_link_input_cb(mac, emac_lwip_input, netif); 00064 mac->ops.set_link_state_cb(mac, emac_lwip_state_change, netif); 00065 00066 if (!mac->ops.power_up(mac)) { 00067 err = ERR_IF; 00068 } 00069 00070 netif->mtu = mac->ops.get_mtu_size(mac); 00071 netif->hwaddr_len = mac->ops.get_hwaddr_size(mac); 00072 mac->ops.get_hwaddr(mac, netif->hwaddr); 00073 00074 /* Interface capabilities */ 00075 netif->flags = NETIF_FLAG_BROADCAST | NETIF_FLAG_ETHARP | NETIF_FLAG_ETHERNET | NETIF_FLAG_IGMP; 00076 00077 mac->ops.get_ifname(mac, netif->name, 2); 00078 00079 #if LWIP_IPV4 00080 netif->output = etharp_output; 00081 #endif /* LWIP_IPV4 */ 00082 00083 netif->linkoutput = emac_lwip_low_level_output; 00084 00085 return err; 00086 } 00087 00088 #endif /* DEVICE_EMAC */
Generated on Sun Jul 17 2022 08:25:22 by
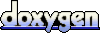