
Rtos API example
create.cpp File Reference
Test cases to close KVs in the CFSTORE using the drv->Create() API function. More...
Go to the source code of this file.
Functions | |
static int32_t | cfstore_create_kv_create (size_t name_len, char *name_tag, char *value_buf, size_t value_len) |
support function to create a KV
| |
control_t | cfstore_create_test_01_end (const size_t call_count) |
Test case to change the value blob size of pre-existing key. | |
control_t | cfstore_create_test_02_end (const size_t call_count) |
Test case to create ~10 kvs. | |
control_t | cfstore_create_test_03_end (const size_t call_count) |
Test to create the ~100 kvs to make the device run out of memory. | |
control_t | cfstore_create_test_04_end (const size_t call_count) |
Test to create the 100 kvs to make the device run out of memory. | |
int32_t | cfstore_create_test_05_core (const size_t call_count) |
Support function for test cases 05. | |
control_t | cfstore_create_test_05 (const size_t call_count) |
Test case to check cfstore recovers from out of memory errors without leaking memory. | |
bool | cfstore_create_key_name_validate (const char *key_name, bool should_create) |
Test whether a key name can be created or not. | |
control_t | cfstore_create_test_06_end (const size_t call_count) |
Test that key names with non-matching braces etc do no get created. | |
control_t | cfstore_create_test_07_end (const size_t call_count) |
Test case to change the value blob size of pre-existing key in a way that causes the memory area to realloc-ed,. |
Detailed Description
Test cases to close KVs in the CFSTORE using the drv->Create() API function.
Please consult the documentation under the test-case functions for a description of the individual test case.
Definition in file create.cpp.
Function Documentation
bool cfstore_create_key_name_validate | ( | const char * | key_name, |
bool | should_create | ||
) |
Test whether a key name can be created or not.
- Parameters:
-
key_name name of the key to create in the store should_create if true, then create KV should succeed, otherwise should fail.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 634 of file create.cpp.
static int32_t cfstore_create_kv_create | ( | size_t | name_len, |
char * | name_tag, | ||
char * | value_buf, | ||
size_t | value_len | ||
) | [static] |
support function to create a KV
- a kv name is generated with the length name_len
- a kv value blob is generated with the length value_len
- Parameters:
-
name_len the length of the kv_name name_tag tag to append to name, intended to enable large number of unique strings value_buf buffer to use for storing the generated value data value_len the length of the value to generate
Definition at line 173 of file create.cpp.
control_t cfstore_create_test_01_end | ( | const size_t | call_count ) |
Test case to change the value blob size of pre-existing key.
The test does the following:
- creates a cfstore with ~10 entries.
- for a mid-cfstore entry, double the value blob size.
- check all the cfstore entries can be read correctly and their data agrees with the data supplied upon creation.
- shrink the mid-entry value blob size to be ~half the initial size.
- check all the cfstore entries can be read correctly and their data agrees with the data supplied upon creation.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 290 of file create.cpp.
control_t cfstore_create_test_02_end | ( | const size_t | call_count ) |
Test case to create ~10 kvs.
The amount of data store in the cfstore is as follows:
- 10 kvs
- kv name lengths are ~220 => ~ 2200 bytes
- value blob length is 1x256, 2x256, 3x256, ... 10x256)) = 256(1+2+3+4+..10) = 256*10*11/2 = 14080
- kv overhead = 8bytes/kv = 8 * 10 = 80bytes
- total = (220*10)+256*10*11/2 10*8 = 143800 bytes
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 405 of file create.cpp.
control_t cfstore_create_test_03_end | ( | const size_t | call_count ) |
Test to create the ~100 kvs to make the device run out of memory.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 429 of file create.cpp.
control_t cfstore_create_test_04_end | ( | const size_t | call_count ) |
Test to create the 100 kvs to make the device run out of memory.
The amount of data store in the cfstore is as follows:
- total = (220*100)+256*100*101/2 100*8 = 1315600 = 1.315x10^6
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 458 of file create.cpp.
control_t cfstore_create_test_05 | ( | const size_t | call_count ) |
Test case to check cfstore recovers from out of memory errors without leaking memory.
This test case does the following: 1. Start loop. 2. Initializes CFSTORE. 3. Creates as many KVs as required to run out of memory. The number of bytes B allocated before running out of memory is recorded. 4. For loop i, check that B_i = B_i-1 for i>0 i.e. that no memory has been leaked 5. Uninitialize(), which should clean up any cfstore internal state including freeing the internal memeory area. 6. Repeat from step 1 N times.
Definition at line 569 of file create.cpp.
int32_t cfstore_create_test_05_core | ( | const size_t | call_count ) |
Support function for test cases 05.
Create enough KV's to consume the whole of available memory
Definition at line 511 of file create.cpp.
control_t cfstore_create_test_06_end | ( | const size_t | call_count ) |
Test that key names with non-matching braces etc do no get created.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 683 of file create.cpp.
control_t cfstore_create_test_07_end | ( | const size_t | call_count ) |
Test case to change the value blob size of pre-existing key in a way that causes the memory area to realloc-ed,.
The test is a modified version of cfstore_create_test_01_end which
- creates KVs,
- mallocs a memory object on the heap
- increases the size of one of the existing KVs, causing the internal memory area to be realloc-ed.
In detail, the test does the following: 1. creates a cfstore with ~10 entries. This causes the configuration store to realloc() heap memory for KV storage. 2. mallocs a memory object on heap. 3. for a mid-cfstore entry, double the value blob size. This will cause the cfstore memory area to be realloced. 4. check all the cfstore entries can be read correctly and their data agrees with the data supplied upon creation. 5. shrink the mid-entry value blob size to be ~half the initial size. check all the cfstore entries can be read correctly and their data agrees with the data supplied upon creation.
- Returns:
- on success returns CaseNext to continue to next test case, otherwise will assert on errors.
Definition at line 730 of file create.cpp.
Generated on Sun Jul 17 2022 08:25:34 by
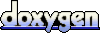