
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
create.cpp
Go to the documentation of this file.
00001 /* 00002 * mbed Microcontroller Library 00003 * Copyright (c) 2006-2016 ARM Limited 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); 00006 * you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, 00013 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00014 * See the License for the specific language governing permissions and 00015 * limitations under the License. 00016 * 00017 * Test cases to create KVs in the CFSTORE using the drv->Create() API call. 00018 */ 00019 00020 /** @file create.cpp Test cases to close KVs in the CFSTORE using the 00021 * drv->Create() API function. 00022 * 00023 * Please consult the documentation under the test-case functions for 00024 * a description of the individual test case. 00025 */ 00026 00027 #include "mbed.h" 00028 #include "mbed_stats.h" 00029 #include "cfstore_config.h" 00030 #include "cfstore_debug.h" 00031 #include "cfstore_test.h" 00032 #include "Driver_Common.h" 00033 #include "configuration_store.h" 00034 #include "utest/utest.h" 00035 #include "unity/unity.h" 00036 #include "greentea-client/test_env.h" 00037 #include "cfstore_utest.h" 00038 00039 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00040 #include "uvisor-lib/uvisor-lib.h" 00041 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00042 00043 #include <stdio.h> 00044 #include <stdlib.h> 00045 #include <string.h> 00046 #include <inttypes.h> 00047 00048 using namespace utest::v1; 00049 00050 #ifdef CFSTORE_DEBUG 00051 #define CFSTORE_CREATE_GREENTEA_TIMEOUT_S 360 00052 #else 00053 #define CFSTORE_CREATE_GREENTEA_TIMEOUT_S 60 00054 #endif 00055 #define CFSTORE_CREATE_MALLOC_SIZE 1024 00056 00057 static char cfstore_create_utest_msg_g[CFSTORE_UTEST_MSG_BUF_SIZE]; 00058 00059 /* Configure secure box. */ 00060 #ifdef YOTTA_CFG_CFSTORE_UVISOR 00061 UVISOR_BOX_NAMESPACE("com.arm.mbed.cfstore.test.create.box1"); 00062 UVISOR_BOX_CONFIG(cfstore_create_box1, UVISOR_BOX_STACK_SIZE); 00063 #endif /* YOTTA_CFG_CFSTORE_UVISOR */ 00064 00065 00066 /// @cond CFSTORE_DOXYGEN_DISABLE 00067 #define CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_01 { "Lefkada.Vathi.Meganisi.Atokos.Vathi.Ithaki.PeriPigathi.AgiosAndreas.Sami.Kefalonia.AgiaEffimia.AgiaSofia.Fiskardo.Frikes.Kioni.Meganissi.Lefkada", "Penelope"} 00068 #define CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_02 { "Iolcus.Lemnos.Salmydessus.Cyzicus.Cios.Berbryces.Symplegadese.IsleAres.Colchis.Anenea.Sirens.Scylia.Charybdis.Phaeacia.Triton.Iolcus", "Medea"} 00069 #define CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_03 { "338.Chaeronea.336.Macedonia.334.Granicus.333.Issus.332.Tyre.331.Gaugamela.330.Persepolis.Philotas.Parmenion.329.Bactria.Oxus.Samarkand.328.Cleitus.327.Roxane.326.Hydaspes.Bucephalus.324.Hephaestion.323.AlexanderDies", "TheGreat"} 00070 #define CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_01 { "0123456789abcdef0123456", "abcdefghijklmnopqrstuvwxyz"} 00071 #define CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_02 { "0123456789abcdef0123456", "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ"} 00072 #define CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_03 { "0123456789abcdef0123456", "nopqrstuvwxyz"} 00073 #define CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_01 { "Time.Will.Explain.It.All", "Aegeus"} 00074 #define CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_02 { "Cleverness.Is.Not.Wisdom", "Bacchae"} 00075 #define CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_03 { "Talk.Sense.To.A.Fool.And.He.Calls.You.Foolish", "Bacchae"} 00076 #define CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_04 { "There.is.in.the.worst.of.fortune.the.best.of.chances.for.a.happy.change", "Iphigenia.in.Tauris"} 00077 00078 static cfstore_kv_data_t cfstore_create_test_01_data[] = { 00079 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_01, 00080 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_02, 00081 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_03, 00082 { NULL, NULL}, 00083 }; 00084 00085 /* table 1: to initialise cfstore with CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_01 */ 00086 static cfstore_kv_data_t cfstore_create_test_01_data_step_01[] = { 00087 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_01, 00088 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_02, 00089 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_03, 00090 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_01, 00091 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_01, 00092 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_02, 00093 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_03, 00094 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_04, 00095 { NULL, NULL}, 00096 }; 00097 00098 /* table 2: to CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_01 grown to CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_02 */ 00099 static cfstore_kv_data_t cfstore_create_test_01_data_step_02[] = { 00100 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_01, 00101 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_02, 00102 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_03, 00103 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_02, 00104 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_01, 00105 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_02, 00106 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_03, 00107 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_04, 00108 { NULL, NULL}, 00109 }; 00110 00111 /* table 3: to CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_02 shrunk to CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_03 */ 00112 static cfstore_kv_data_t cfstore_create_test_01_data_step_03[] = { 00113 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_01, 00114 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_02, 00115 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_03, 00116 CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_03, 00117 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_01, 00118 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_02, 00119 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_03, 00120 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_04, 00121 { NULL, NULL}, 00122 }; 00123 00124 /* table 3: CFSTORE_CREATE_TEST_01_TABLE_MID_ENTRY_03 deleted */ 00125 static cfstore_kv_data_t cfstore_create_test_01_data_step_04[] = { 00126 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_01, 00127 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_02, 00128 CFSTORE_CREATE_TEST_01_TABLE_HEAD_ENTRY_03, 00129 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_01, 00130 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_02, 00131 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_03, 00132 CFSTORE_CREATE_TEST_01_TABLE_TAIL_ENTRY_04, 00133 { NULL, NULL}, 00134 }; 00135 /// @endcond 00136 00137 /* support functions */ 00138 00139 /* @brief support function for generating value blob data */ 00140 static int32_t cfstore_create_kv_value_gen(char* value, const size_t len) 00141 { 00142 size_t i = 0; 00143 size_t cpy_size = 0; 00144 00145 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00146 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: value pointer is null.\n", __func__); 00147 TEST_ASSERT_MESSAGE(value != NULL, cfstore_create_utest_msg_g); 00148 00149 while(i < len) 00150 { 00151 cpy_size = len - i > CFSTORE_TEST_BYTE_DATA_TABLE_SIZE ? CFSTORE_TEST_BYTE_DATA_TABLE_SIZE : len - i; 00152 memcpy(value + i, cfstore_test_byte_data_table, cpy_size); 00153 i += cpy_size; 00154 } 00155 return ARM_DRIVER_OK; 00156 } 00157 00158 00159 static char* CFSTORE_CREATE_KV_CREATE_NO_TAG = NULL; 00160 00161 /** @brief 00162 * 00163 * support function to create a KV 00164 * - a kv name is generated with the length name_len 00165 * - a kv value blob is generated with the length value_len 00166 * 00167 * @param name_len the length of the kv_name 00168 * @param name_tag tag to append to name, intended to enable large number of unique strings 00169 * @param value_buf buffer to use for storing the generated value data 00170 * @param value_len the length of the value to generate 00171 * 00172 */ 00173 static int32_t cfstore_create_kv_create(size_t name_len, char* name_tag, char* value_buf, size_t value_len) 00174 { 00175 int32_t ret = ARM_DRIVER_OK; 00176 size_t name_len_ex = name_len; 00177 char kv_name[CFSTORE_KEY_NAME_MAX_LENGTH+1]; /* extra char for terminating null */ 00178 ARM_CFSTORE_KEYDESC kdesc; 00179 00180 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00181 memset(kv_name, 0, CFSTORE_KEY_NAME_MAX_LENGTH+1); 00182 memset(&kdesc, 0, sizeof(kdesc)); 00183 kdesc.drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE; 00184 00185 name_len_ex = name_len; 00186 if(name_tag){ 00187 name_len_ex += strlen(name_tag); 00188 } 00189 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: max supported KV name length for testing exceeded.\n", __func__); 00190 TEST_ASSERT_MESSAGE(name_len_ex < CFSTORE_KEY_NAME_MAX_LENGTH+1, cfstore_create_utest_msg_g); 00191 00192 ret = cfstore_test_kv_name_gen(kv_name, name_len); 00193 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: unable to generate kv_name.\n", __func__); 00194 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK , cfstore_create_utest_msg_g); 00195 00196 /* append name tag */ 00197 if(name_tag){ 00198 strncat(kv_name, name_tag, CFSTORE_KEY_NAME_MAX_LENGTH); 00199 } 00200 00201 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: kv_name is not the correct length (name_len_ex=%d, expected=%d).\n", __func__, (int) strlen(kv_name), (int) name_len_ex); 00202 TEST_ASSERT_MESSAGE(strlen(kv_name) == name_len_ex, cfstore_create_utest_msg_g); 00203 00204 ret = cfstore_create_kv_value_gen(value_buf, value_len); 00205 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: unable to generate kv_name.\n", __func__); 00206 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK , cfstore_create_utest_msg_g); 00207 00208 ret = cfstore_test_create(kv_name, value_buf, &value_len, &kdesc); 00209 00210 if(ret == ARM_CFSTORE_DRIVER_ERROR_OUT_OF_MEMORY){ 00211 CFSTORE_ERRLOG("%s: Error: out of memory\n", __func__); 00212 return ret; 00213 } 00214 00215 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to create KV in store for kv_name_good(ret=%d).\n", __func__, (int) ret); 00216 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00217 00218 return ret; 00219 } 00220 00221 00222 /* @brief cfstore_create_test_01() support function change the size of a value blob in the cfstore */ 00223 static int32_t cfstore_create_test_KV_change(const cfstore_kv_data_t* old_node, const cfstore_kv_data_t* new_node ) 00224 { 00225 int32_t ret = ARM_DRIVER_ERROR; 00226 size_t len = 0; 00227 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00228 ARM_CFSTORE_HANDLE_INIT(hkey); 00229 ARM_CFSTORE_FMODE flags; 00230 ARM_CFSTORE_KEYDESC kdesc; 00231 00232 CFSTORE_DBGLOG("%s:entered\n", __func__); 00233 memset(&flags, 0, sizeof(flags)); 00234 memset(&kdesc, 0, sizeof(kdesc)); 00235 00236 /* check node key_names are identical */ 00237 if(strncmp(old_node->key_name, new_node->key_name, strlen(old_node->key_name)) != 0){ 00238 CFSTORE_ERRLOG("%s:old and new entries so not have the same key_name (old_key_name=%s, new_key_name=%s).\n", __func__, old_node->key_name, new_node->key_name); 00239 return ret; 00240 } 00241 len = strlen(new_node->value); 00242 /* supply NULL key descriptor to open a pre-existing key for increasing the blob size */ 00243 ret = drv->Create(new_node->key_name, len, NULL, hkey); 00244 if(ret < ARM_DRIVER_OK){ 00245 CFSTORE_ERRLOG("%s:Error: failed to change size of KV (key_name=%s)(ret=%d).\n", __func__, new_node->key_name, (int) ret); 00246 goto out1; 00247 } 00248 len = strlen(new_node->value); 00249 ret = drv->Write(hkey, new_node->value, &len); 00250 if(ret < ARM_DRIVER_OK){ 00251 CFSTORE_ERRLOG("%s:Error: failed to write KV (key_name=%s)(ret=%d).\n", __func__, new_node->key_name, (int) ret); 00252 goto out2; 00253 } 00254 if(len != strlen(new_node->value)){ 00255 CFSTORE_DBGLOG("%s:Failed wrote (%d) rather than the correct number of bytes (%d).\n", __func__, (int) len, (int) strlen(cfstore_create_test_01_data[1].value)); 00256 goto out2; 00257 } 00258 out2: 00259 drv->Close(hkey); 00260 out1: 00261 return ret; 00262 } 00263 00264 /* report whether built/configured for flash sync or async mode */ 00265 static control_t cfstore_create_test_00(const size_t call_count) 00266 { 00267 int32_t ret = ARM_DRIVER_ERROR; 00268 00269 (void) call_count; 00270 ret = cfstore_test_startup(); 00271 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to perform test startup (ret=%d).\n", __func__, (int) ret); 00272 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00273 return CaseNext; 00274 } 00275 00276 00277 /** @brief Test case to change the value blob size of pre-existing key. 00278 * 00279 * The test does the following: 00280 * - creates a cfstore with ~10 entries. 00281 * - for a mid-cfstore entry, double the value blob size. 00282 * - check all the cfstore entries can be read correctly and their 00283 * data agrees with the data supplied upon creation. 00284 * - shrink the mid-entry value blob size to be ~half the initial size. 00285 * - check all the cfstore entries can be read correctly and their 00286 * data agrees with the data supplied upon creation. 00287 * 00288 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00289 */ 00290 control_t cfstore_create_test_01_end(const size_t call_count) 00291 { 00292 int32_t ret = ARM_DRIVER_ERROR; 00293 ARM_CFSTORE_FMODE flags; 00294 cfstore_kv_data_t* node = NULL; 00295 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00296 00297 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00298 (void) call_count; 00299 memset(&flags, 0, sizeof(flags)); 00300 00301 ret = cfstore_test_create_table(cfstore_create_test_01_data_step_01); 00302 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to add cfstore_create_test_01_data_head (ret=%d).\n", __func__, (int) ret); 00303 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00304 00305 /* find cfstore_create_test_01_data[0] and grow the KV MID_ENTRY_01 to MID_ENTRY_02 */ 00306 ret = cfstore_create_test_KV_change(&cfstore_create_test_01_data[0], &cfstore_create_test_01_data[1]); 00307 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to increase size of KV (ret=%d).\n", __func__, (int) ret); 00308 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00309 00310 /* Now check that the KVs are all present and correct */ 00311 node = cfstore_create_test_01_data_step_02; 00312 while(node->key_name != NULL) 00313 { 00314 ret = cfstore_test_check_node_correct(node); 00315 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00316 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00317 node++; 00318 } 00319 /* revert CFSTORE_LOG for more trace */ 00320 CFSTORE_DBGLOG("KV successfully increased in size and other KVs remained unchanged.%s", "\n"); 00321 /* Shrink the KV from KV MID_ENTRY_02 to MID_ENTRY_03 */ 00322 ret = cfstore_create_test_KV_change(&cfstore_create_test_01_data[1], &cfstore_create_test_01_data[2]); 00323 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to decrease size of KV (ret=%d).\n", __func__, (int) ret); 00324 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00325 00326 /* Now check that the KVs are all present and correct */ 00327 node = cfstore_create_test_01_data_step_03; 00328 while(node->key_name != NULL) 00329 { 00330 ret = cfstore_test_check_node_correct(node); 00331 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00332 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00333 node++; 00334 } 00335 /* revert CFSTORE_LOG for more trace */ 00336 CFSTORE_DBGLOG("KV successfully decreased in size and other KVs remained unchanged.%s", "\n"); 00337 00338 /* Delete the KV */ 00339 ret = cfstore_test_delete(cfstore_create_test_01_data[2].key_name); 00340 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:failed to delete node(key_name=\"%s\")\n", __func__, node->key_name); 00341 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00342 00343 /* Now check that the KVs are all present and correct */ 00344 node = cfstore_create_test_01_data_step_04; 00345 while(node->key_name != NULL) 00346 { 00347 ret = cfstore_test_check_node_correct(node); 00348 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00349 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00350 node++; 00351 } 00352 ret = drv->Uninitialize(); 00353 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00354 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00355 return CaseNext; 00356 } 00357 00358 00359 static int32_t cfstore_create_test_02_core(const size_t call_count) 00360 { 00361 int32_t ret = ARM_DRIVER_ERROR; 00362 uint32_t i = 0; 00363 uint32_t bytes_stored = 0; 00364 const uint32_t max_num_kvs_create = 10; 00365 const size_t kv_name_min_len = CFSTORE_KEY_NAME_MAX_LENGTH - max_num_kvs_create; 00366 const size_t kv_value_min_len = CFSTORE_TEST_BYTE_DATA_TABLE_SIZE; 00367 const size_t max_value_buf_size = kv_value_min_len * (max_num_kvs_create +1); 00368 char value_buf[max_value_buf_size]; 00369 00370 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00371 (void) call_count; 00372 memset(value_buf, 0, max_value_buf_size); 00373 00374 for(i = 0; i < max_num_kvs_create; i++) 00375 { 00376 memset(value_buf, 0, max_value_buf_size); 00377 ret = cfstore_create_kv_create(kv_name_min_len +i, CFSTORE_CREATE_KV_CREATE_NO_TAG, value_buf, kv_value_min_len * (i+1)); 00378 bytes_stored += kv_name_min_len + i; /* kv_name */ 00379 bytes_stored += kv_value_min_len * (i+1); /* kv value blob */ 00380 bytes_stored += 8; /* kv overhead */ 00381 if(ret == ARM_CFSTORE_DRIVER_ERROR_OUT_OF_MEMORY){ 00382 CFSTORE_ERRLOG("Out of memory on %d-th KV, trying to allocate memory totalling %d.\n", (int) i, (int) bytes_stored); 00383 break; 00384 } 00385 CFSTORE_DBGLOG("Successfully stored %d-th KV bytes, totalling %d.\n", (int) i, (int) bytes_stored); 00386 } 00387 ret = cfstore_test_delete_all(); 00388 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete_all() attributes to clean up after test (ret=%d).\n", __func__, (int) ret); 00389 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00390 return ret; 00391 } 00392 00393 00394 /**@brief 00395 * 00396 * Test case to create ~10 kvs. The amount of data store in the cfstore is as follows: 00397 * - 10 kvs 00398 * - kv name lengths are ~220 => ~ 2200 bytes 00399 * - value blob length is 1x256, 2x256, 3x256, ... 10x256)) = 256(1+2+3+4+..10) = 256*10*11/2 = 14080 00400 * - kv overhead = 8bytes/kv = 8 * 10 = 80bytes 00401 * - total = (220*10)+256*10*11/2 10*8 = 143800 bytes 00402 * 00403 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00404 */ 00405 control_t cfstore_create_test_02_end(const size_t call_count) 00406 { 00407 int32_t ret; 00408 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00409 00410 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00411 (void) call_count; 00412 00413 ret = cfstore_create_test_02_core(call_count); 00414 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: something went wrong (ret=%d).\n", __func__, (int) ret); 00415 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00416 00417 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00418 TEST_ASSERT_MESSAGE(drv->Uninitialize() >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00419 return CaseNext; 00420 } 00421 00422 00423 /**@brief 00424 * 00425 * Test to create the ~100 kvs to make the device run out of memory. 00426 * 00427 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00428 */ 00429 control_t cfstore_create_test_03_end(const size_t call_count) 00430 { 00431 int32_t i = 0; 00432 int32_t ret; 00433 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00434 00435 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00436 for(i = 0; i < 100; i++) 00437 { 00438 ret = cfstore_create_test_02_core(call_count); 00439 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: something went wrong (ret=%d).\n", __func__, (int) ret); 00440 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00441 /* revert CFSTORE_LOG for more trace */ 00442 CFSTORE_DBGLOG("Successfully completed create/destroy loop %d.\n", (int) i); 00443 } 00444 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00445 TEST_ASSERT_MESSAGE(drv->Uninitialize() >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00446 return CaseNext; 00447 } 00448 00449 00450 /**@brief 00451 * 00452 * Test to create the 100 kvs to make the device run out of memory. 00453 * The amount of data store in the cfstore is as follows: 00454 * - total = (220*100)+256*100*101/2 100*8 = 1315600 = 1.315x10^6 00455 * 00456 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00457 */ 00458 control_t cfstore_create_test_04_end(const size_t call_count) 00459 { 00460 int32_t ret = ARM_DRIVER_ERROR; 00461 uint32_t i = 0; 00462 uint32_t bytes_stored = 0; 00463 const uint32_t max_num_kvs_create = 100; 00464 const size_t kv_name_min_len = CFSTORE_KEY_NAME_MAX_LENGTH - max_num_kvs_create; 00465 const size_t kv_value_min_len = CFSTORE_TEST_BYTE_DATA_TABLE_SIZE; 00466 const size_t max_value_buf_size = kv_value_min_len/8 * (max_num_kvs_create +1); 00467 char* value_buf = NULL; 00468 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00469 00470 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00471 (void) call_count; 00472 00473 CFSTORE_LOG("%s: cfstore_test_dump: dump here contents of CFSTORE so we know whats present\n", __func__); 00474 ret = cfstore_test_dump(); 00475 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: D.1.1 cfstore_test_dump failed (ret=%d).\n", __func__, (int) ret); 00476 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00477 00478 value_buf = (char*) malloc(max_value_buf_size); 00479 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: out of memory.\n", __func__); 00480 TEST_ASSERT_MESSAGE(value_buf != NULL, cfstore_create_utest_msg_g); 00481 00482 for(i = 0; i < max_num_kvs_create; i++) 00483 { 00484 memset(value_buf, 0, max_value_buf_size); 00485 ret = cfstore_create_kv_create(kv_name_min_len +i, CFSTORE_CREATE_KV_CREATE_NO_TAG, value_buf, kv_value_min_len/8 * (i+1)); 00486 bytes_stored += kv_name_min_len + i; /* kv_name */ 00487 bytes_stored += kv_value_min_len/8 * (i+1); /* kv value blob */ 00488 bytes_stored += 8; /* kv overhead */ 00489 if(ret == ARM_CFSTORE_DRIVER_ERROR_OUT_OF_MEMORY){ 00490 CFSTORE_ERRLOG("Out of memory on %d-th KV, trying to allocate memory totalling %d.\n", (int) i, (int) bytes_stored); 00491 break; 00492 } 00493 /* revert CFSTORE_LOG for more trace */ 00494 CFSTORE_DBGLOG("Successfully stored %d-th KV bytes, totalling %d.\n", (int) i, (int) bytes_stored); 00495 } 00496 ret = cfstore_test_delete_all(); 00497 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete_all() attributes to clean up after test (ret=%d).\n", __func__, (int) ret); 00498 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00499 free(value_buf); 00500 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00501 TEST_ASSERT_MESSAGE(drv->Uninitialize() >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00502 return CaseNext; 00503 } 00504 00505 00506 /** 00507 * @brief Support function for test cases 05 00508 * 00509 * Create enough KV's to consume the whole of available memory 00510 */ 00511 int32_t cfstore_create_test_05_core(const size_t call_count) 00512 { 00513 int32_t ret = ARM_DRIVER_ERROR; 00514 uint32_t i = 0; 00515 const uint32_t max_num_kvs_create = 200; 00516 const size_t kv_name_tag_len = 3; 00517 const size_t kv_name_min_len = 10; 00518 const size_t kv_value_min_len = CFSTORE_TEST_BYTE_DATA_TABLE_SIZE; 00519 const size_t max_value_buf_size = kv_value_min_len/64 * (max_num_kvs_create+1); 00520 char kv_name_tag_buf[kv_name_tag_len+1]; 00521 char* value_buf = NULL; 00522 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00523 00524 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00525 00526 /* Initialize() */ 00527 cfstore_utest_default_start(call_count); 00528 00529 value_buf = (char*) malloc(max_value_buf_size); 00530 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: out of memory.\n", __func__); 00531 TEST_ASSERT_MESSAGE(value_buf != NULL, cfstore_create_utest_msg_g); 00532 00533 for(i = 0; i < max_num_kvs_create; i++) 00534 { 00535 memset(value_buf, 0, max_value_buf_size); 00536 snprintf(kv_name_tag_buf, kv_name_tag_len+1, "%0d", (int) i); 00537 ret = cfstore_create_kv_create(kv_name_min_len, kv_name_tag_buf, value_buf, kv_value_min_len/64 * (i+1)); 00538 if(ret == ARM_CFSTORE_DRIVER_ERROR_OUT_OF_MEMORY){ 00539 CFSTORE_ERRLOG("Out of memory on %d-th KV.\n", (int) i); 00540 break; 00541 } 00542 /* revert CFSTORE_LOG for more trace */ 00543 CFSTORE_DBGLOG("Successfully stored %d-th KV.\n", (int) i); 00544 } 00545 ret = cfstore_test_delete_all(); 00546 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to delete_all() attributes to clean up after test.\n", __func__); 00547 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00548 free(value_buf); 00549 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00550 TEST_ASSERT_MESSAGE(drv->Uninitialize() >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00551 return ret; 00552 } 00553 00554 00555 /** 00556 * @brief Test case to check cfstore recovers from out of memory 00557 * errors without leaking memory 00558 * 00559 * This test case does the following: 00560 * 1. Start loop. 00561 * 2. Initializes CFSTORE. 00562 * 3. Creates as many KVs as required to run out of memory. The number of bytes B 00563 * allocated before running out of memory is recorded. 00564 * 4. For loop i, check that B_i = B_i-1 for i>0 i.e. that no memory has been leaked 00565 * 5. Uninitialize(), which should clean up any cfstore internal state including 00566 * freeing the internal memeory area. 00567 * 6. Repeat from step 1 N times. 00568 */ 00569 control_t cfstore_create_test_05(const size_t call_count) 00570 { 00571 uint32_t i = 0; 00572 int32_t ret = ARM_DRIVER_ERROR; 00573 const uint32_t max_loops = 50; 00574 mbed_stats_heap_t stats_before; 00575 mbed_stats_heap_t stats_after; 00576 00577 mbed_stats_heap_get(&stats_before); 00578 00579 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00580 for(i = 0; i < max_loops; i++) { 00581 ret = cfstore_create_test_05_core(call_count); 00582 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: cfstore_create_test_05_core() failed (ret = %d.\n", __func__, (int) ret); 00583 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00584 00585 mbed_stats_heap_get(&stats_after); 00586 if(i > 1) { 00587 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: memory leak: stored %d bytes on loop %d, but %d bytes on loop %d .\n", __func__, (int) stats_after.current_size, (int) i, (int) stats_before.current_size, (int) i-1); 00588 TEST_ASSERT_MESSAGE(stats_after.current_size == stats_before.current_size, cfstore_create_utest_msg_g); 00589 TEST_ASSERT(stats_after.alloc_fail_cnt > stats_before.alloc_fail_cnt); 00590 } 00591 stats_before = stats_after; 00592 } 00593 return CaseNext; 00594 } 00595 00596 00597 /// @cond CFSTORE_DOXYGEN_DISABLE 00598 /* structure to encode test data */ 00599 typedef struct cfstore_create_key_name_validate_t { 00600 const char* key_name; 00601 uint32_t f_allowed : 1; 00602 } cfstore_create_key_name_validate_t; 00603 00604 /* data table encoding test data */ 00605 cfstore_create_key_name_validate_t cfstore_create_test_06_data[] = { 00606 /* ruler for measuring text strings */ 00607 /* 1 1 1 1 1 1 1 1 1 1 2 2 2 */ 00608 /* 0 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 6 7 8 9 0 1 2 */ 00609 /* 1234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890 */ 00610 { "", false}, 00611 { "abc.{1}.efg", true }, 00612 { "abc.{1.efg", false }, 00613 { "abc.1}.efg", false }, 00614 { "abc.{{1}}.efg", false }, 00615 { "abc.{}.efg", true }, 00616 { "abc.}1{.efg", false }, 00617 { ".piety.demands.us.to.honour.truth.above.our.friends", false }, 00618 { "basement.medicine.pavement.government.trenchcoat.off.cough.off.kid.did.when.again.alleyway.friend.cap.pen.dollarbills.ten.foot.soot.put.but.anyway.say.May.DA.kid.did.toes.bows.those.hose.nose.clothes.man.blows.{100000000}", false }, 00619 { NULL, false}, 00620 }; 00621 /// @endcond 00622 00623 00624 /** 00625 * @brief Test whether a key name can be created or not. 00626 * 00627 * @param key_name 00628 * name of the key to create in the store 00629 * @param should_create 00630 * if true, then create KV should succeed, otherwise should fail. 00631 * 00632 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00633 */ 00634 bool cfstore_create_key_name_validate(const char *key_name, bool should_create) 00635 { 00636 bool bret = false; 00637 char* test_data = (char*) "test_data"; 00638 int32_t ret = ARM_DRIVER_ERROR; 00639 ARM_CFSTORE_SIZE len = 0; 00640 ARM_CFSTORE_KEYDESC kdesc; 00641 00642 CFSTORE_FENTRYLOG("%s:entered\r\n", __func__); 00643 memset(&kdesc, 0, sizeof(kdesc)); 00644 00645 /* create */ 00646 kdesc.drl = ARM_RETENTION_WHILE_DEVICE_ACTIVE; 00647 len = strlen(test_data); 00648 ret = cfstore_test_create((const char*) key_name, test_data, &len, &kdesc); 00649 /* dont not use any functions that require finding the created item as they may not work, 00650 * depending on the construction of the test key_name & match strings */ 00651 if(should_create == true) 00652 { 00653 if(ret < ARM_DRIVER_OK){ 00654 CFSTORE_ERRLOG("%s:Error: failed to create kv (key_name=%s.\r\n", __func__, key_name); 00655 return bret; 00656 } 00657 CFSTORE_DBGLOG("%s:Success: Create() behaved as expected.\r\n", __func__); 00658 /* delete using the actual name */ 00659 ret = cfstore_test_delete((const char*) key_name); 00660 if(ret < ARM_DRIVER_OK){ 00661 CFSTORE_ERRLOG("%s:Error: failed to delete kv (key_name=%s)(ret=%d).\r\n", __func__, key_name, (int)ret); 00662 } 00663 bret = true; 00664 } 00665 else 00666 { 00667 if(ret >= ARM_DRIVER_OK){ 00668 CFSTORE_ERRLOG("%s:Error: created kv (key_name=%s) when Create() should have failed.\r\n", __func__, key_name); 00669 return bret; 00670 } 00671 /* the operation failed which was the expected result hence return true*/ 00672 bret = true; 00673 } 00674 return bret; 00675 } 00676 00677 /** @brief 00678 * 00679 * Test that key names with non-matching braces etc do no get created. 00680 * 00681 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00682 */ 00683 control_t cfstore_create_test_06_end(const size_t call_count) 00684 { 00685 bool ret = false; 00686 int32_t ret32 = ARM_DRIVER_ERROR; 00687 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00688 cfstore_create_key_name_validate_t* node = cfstore_create_test_06_data; 00689 00690 (void) call_count; 00691 00692 while(node->key_name != NULL) 00693 { 00694 ret = cfstore_create_key_name_validate(node->key_name, node->f_allowed); 00695 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: test failed (ret=%d, key_name=%s, f_allowed=%d)\n", __func__, (int) ret, node->key_name, (int) node->f_allowed); 00696 TEST_ASSERT_MESSAGE(ret == true, cfstore_create_utest_msg_g); 00697 node++; 00698 } 00699 00700 ret32 = drv->Uninitialize(); 00701 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00702 TEST_ASSERT_MESSAGE(ret32 >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00703 return CaseNext; 00704 } 00705 00706 00707 /** @brief Test case to change the value blob size of pre-existing 00708 * key in a way that causes the memory area to realloc-ed, 00709 * 00710 * The test is a modified version of cfstore_create_test_01_end which 00711 * - creates KVs, 00712 * - mallocs a memory object on the heap 00713 * - increases the size of one of the existing KVs, causing the 00714 * internal memory area to be realloc-ed. 00715 * 00716 * In detail, the test does the following: 00717 * 1. creates a cfstore with ~10 entries. This causes the configuration 00718 * store to realloc() heap memory for KV storage. 00719 * 2. mallocs a memory object on heap. 00720 * 3. for a mid-cfstore entry, double the value blob size. This will cause the 00721 * cfstore memory area to be realloced. 00722 * 4. check all the cfstore entries can be read correctly and their 00723 * data agrees with the data supplied upon creation. 00724 * 5. shrink the mid-entry value blob size to be ~half the initial size. 00725 * check all the cfstore entries can be read correctly and their 00726 * data agrees with the data supplied upon creation. 00727 * 00728 * @return on success returns CaseNext to continue to next test case, otherwise will assert on errors. 00729 */ 00730 control_t cfstore_create_test_07_end(const size_t call_count) 00731 { 00732 int32_t ret = ARM_DRIVER_ERROR; 00733 void *test_buf1 = NULL; 00734 ARM_CFSTORE_FMODE flags; 00735 cfstore_kv_data_t* node = NULL; 00736 ARM_CFSTORE_DRIVER* drv = &cfstore_driver; 00737 00738 CFSTORE_FENTRYLOG("%s:entered\n", __func__); 00739 (void) call_count; 00740 memset(&flags, 0, sizeof(flags)); 00741 00742 /* step 1 */ 00743 ret = cfstore_test_create_table(cfstore_create_test_01_data_step_01); 00744 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to add cfstore_create_test_01_data_head (ret=%d).\n", __func__, (int) ret); 00745 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00746 00747 /* step 2 */ 00748 test_buf1 = malloc(CFSTORE_CREATE_MALLOC_SIZE); 00749 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: failed to allocate memory (test_buf1=%p)\n", __func__, test_buf1); 00750 TEST_ASSERT_MESSAGE(test_buf1 != NULL, cfstore_create_utest_msg_g); 00751 00752 /* step 3. find cfstore_create_test_01_data[0] and grow the KV MID_ENTRY_01 to MID_ENTRY_02 */ 00753 ret = cfstore_create_test_KV_change(&cfstore_create_test_01_data[0], &cfstore_create_test_01_data[1]); 00754 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to increase size of KV (ret=%d).\n", __func__, (int) ret); 00755 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00756 00757 /* step 4. Now check that the KVs are all present and correct */ 00758 node = cfstore_create_test_01_data_step_02; 00759 while(node->key_name != NULL) 00760 { 00761 ret = cfstore_test_check_node_correct(node); 00762 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00763 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00764 node++; 00765 } 00766 /* revert CFSTORE_LOG for more trace */ 00767 CFSTORE_DBGLOG("KV successfully increased in size and other KVs remained unchanged.%s", "\n"); 00768 00769 /* Shrink the KV from KV MID_ENTRY_02 to MID_ENTRY_03 */ 00770 ret = cfstore_create_test_KV_change(&cfstore_create_test_01_data[1], &cfstore_create_test_01_data[2]); 00771 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Failed to decrease size of KV (ret=%d).\n", __func__, (int) ret); 00772 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00773 00774 /* Step 5. Now check that the KVs are all present and correct */ 00775 node = cfstore_create_test_01_data_step_03; 00776 while(node->key_name != NULL) 00777 { 00778 ret = cfstore_test_check_node_correct(node); 00779 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00780 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00781 node++; 00782 } 00783 /* revert CFSTORE_LOG for more trace */ 00784 CFSTORE_DBGLOG("KV successfully decreased in size and other KVs remained unchanged.%s", "\n"); 00785 00786 /* Delete the KV */ 00787 ret = cfstore_test_delete(cfstore_create_test_01_data[2].key_name); 00788 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:failed to delete node(key_name=\"%s\")\n", __func__, node->key_name); 00789 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00790 00791 /* Now check that the KVs are all present and correct */ 00792 node = cfstore_create_test_01_data_step_04; 00793 while(node->key_name != NULL) 00794 { 00795 ret = cfstore_test_check_node_correct(node); 00796 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:node (key_name=\"%s\", value=\"%s\") not correct in cfstore\n", __func__, node->key_name, node->value); 00797 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00798 node++; 00799 } 00800 00801 free(test_buf1); 00802 ret = drv->Uninitialize(); 00803 CFSTORE_TEST_UTEST_MESSAGE(cfstore_create_utest_msg_g, CFSTORE_UTEST_MSG_BUF_SIZE, "%s:Error: Uninitialize() call failed.\n", __func__); 00804 TEST_ASSERT_MESSAGE(ret >= ARM_DRIVER_OK, cfstore_create_utest_msg_g); 00805 return CaseNext; 00806 } 00807 00808 00809 /// @cond CFSTORE_DOXYGEN_DISABLE 00810 utest::v1::status_t greentea_setup(const size_t number_of_cases) 00811 { 00812 GREENTEA_SETUP(CFSTORE_CREATE_GREENTEA_TIMEOUT_S, "default_auto"); 00813 return greentea_test_setup_handler(number_of_cases); 00814 } 00815 00816 Case cases[] = { 00817 /* 1 2 3 4 5 6 7 */ 00818 /* 1234567890123456789012345678901234567890123456789012345678901234567890 */ 00819 Case("CREATE_test_00", cfstore_create_test_00), 00820 Case("CREATE_test_01_start", cfstore_utest_default_start), 00821 Case("CREATE_test_01_end", cfstore_create_test_01_end), 00822 Case("CREATE_test_02_start", cfstore_utest_default_start), 00823 Case("CREATE_test_02_end", cfstore_create_test_02_end), 00824 Case("CREATE_test_03_start", cfstore_utest_default_start), 00825 Case("CREATE_test_03_end", cfstore_create_test_03_end), 00826 Case("CREATE_test_04_start", cfstore_utest_default_start), 00827 Case("CREATE_test_04_end", cfstore_create_test_04_end), 00828 #if defined(MBED_HEAP_STATS_ENABLED) && MBED_HEAP_STATS_ENABLED && !defined(__ICCARM__) 00829 Case("CREATE_test_05", cfstore_create_test_05), 00830 #endif 00831 Case("CREATE_test_06_start", cfstore_utest_default_start), 00832 Case("CREATE_test_06_end", cfstore_create_test_06_end), 00833 Case("CREATE_test_07_start", cfstore_utest_default_start), 00834 Case("CREATE_test_07_end", cfstore_create_test_07_end), 00835 }; 00836 00837 00838 /* Declare your test specification with a custom setup handler */ 00839 Specification specification(greentea_setup, cases); 00840 00841 int main() 00842 { 00843 return !Harness::run(specification); 00844 } 00845 /// @endcond 00846
Generated on Sun Jul 17 2022 08:25:21 by
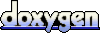