
Rtos API example
Cache Class Reference
Public Member Functions | |
def | cache_file |
def | pdsc_to_pack |
def | get_urls |
def | get_flash_algorthim_binary |
def | get_svd_file |
def | index |
def | aliases |
def | cache_everything |
def | cache_descriptors |
def | cache_descriptor_list |
def | cache_pack_list |
def | pdsc_from_cache |
def | pack_from_cache |
def | cache_and_parse |
Detailed Description
The Cache object is the only relevant API object at the moment Constructing the Cache object does not imply any caching. A user of the API must explicitly call caching functions. :param silent: A boolean that, when True, significantly reduces the printing of this Object :type silent: bool :param no_timeouts: A boolean that, when True, disables the default connection timeout and low speed timeout for downloading things. :type no_timeouts: bool
Definition at line 59 of file arm_pack_manager/__init__.py.
Member Function Documentation
def aliases | ( | self ) |
An index of most of the important data in all cached PDSC files. :Example: >>> from ArmPackManager import Cache >>> a = Cache() >>> a.index["LPC1768"] {u'algorithm': {u'RAMsize': u'0x0FE0', u'RAMstart': u'0x10000000', u'name': u'Flash/LPC_IAP_512.FLM', u'size': u'0x80000', u'start': u'0x00000000'}, u'compile': [u'Device/Include/LPC17xx.h', u'LPC175x_6x'], u'debug': u'SVD/LPC176x5x.svd', u'pdsc_file': u'http://www.keil.com/pack/Keil.LPC1700_DFP.pdsc', u'memory': {u'IRAM1': {u'size': u'0x8000', u'start': u'0x10000000'}, u'IRAM2': {u'size': u'0x8000', u'start': u'0x2007C000'}, u'IROM1': {u'size': u'0x80000', u'start': u'0x00000000'}}}
Definition at line 337 of file arm_pack_manager/__init__.py.
def cache_and_parse | ( | self, | |
url | |||
) |
A low level shortcut that Caches and Parses a PDSC file. :param url: The URL of the PDSC file. :type url: str :return: A parsed representation of the PDSC file. :rtype: BeautifulSoup
Definition at line 438 of file arm_pack_manager/__init__.py.
def cache_descriptor_list | ( | self, | |
list | |||
) |
Cache a list of PDSC files. :param list: URLs of PDSC files to cache. :type list: [str]
Definition at line 386 of file arm_pack_manager/__init__.py.
def cache_descriptors | ( | self ) |
Cache every PDSC file known. Generates an index afterwards. .. note:: This process may use 11MB of drive space and take upwards of 1 minute.
Definition at line 375 of file arm_pack_manager/__init__.py.
def cache_everything | ( | self ) |
Cache every PACK and PDSC file known. Generates an index afterwards. .. note:: This process may use 4GB of drive space and take upwards of 10 minutes to complete.
Definition at line 364 of file arm_pack_manager/__init__.py.
def cache_file | ( | self, | |
url | |||
) |
Low level interface to caching a single file. :param url: The URL to cache. :type url: str :rtype: None
Definition at line 84 of file arm_pack_manager/__init__.py.
def cache_pack_list | ( | self, | |
list | |||
) |
Cache a list of PACK files, referenced by their PDSC URL :param list: URLs of PDSC files to cache. :type list: [str]
Definition at line 397 of file arm_pack_manager/__init__.py.
def get_flash_algorthim_binary | ( | self, | |
device_name, | |||
all = False |
|||
) |
Retrieve the flash algorithm file for a particular part. Assumes that both the PDSC and the PACK file associated with that part are in the cache. :param device_name: The exact name of a device :param all: Return an iterator of all flash algos for this device :type device_name: str :return: A file-like object that, when read, is the ELF file that describes the flashing algorithm :return: A file-like object that, when read, is the ELF file that describes the flashing algorithm. When "all" is set to True then an iterator for file-like objects is returned :rtype: ZipExtFile or ZipExtFile iterator if all is True
Definition at line 250 of file arm_pack_manager/__init__.py.
def get_svd_file | ( | self, | |
device_name | |||
) |
Retrieve the flash algorithm file for a particular part. Assumes that both the PDSC and the PACK file associated with that part are in the cache. :param device_name: The exact name of a device :type device_name: str :return: A file-like object that, when read, is the ELF file that describes the flashing algorithm :rtype: ZipExtFile
Definition at line 268 of file arm_pack_manager/__init__.py.
def get_urls | ( | self ) |
Extract the URLs of all know PDSC files. Will pull the index from the internet if it is not cached. :return: A list of all PDSC URLs :rtype: [str]
Definition at line 132 of file arm_pack_manager/__init__.py.
def index | ( | self ) |
An index of most of the important data in all cached PDSC files. :Example: >>> from ArmPackManager import Cache >>> a = Cache() >>> a.index["LPC1768"] {u'algorithm': {u'RAMsize': u'0x0FE0', u'RAMstart': u'0x10000000', u'name': u'Flash/LPC_IAP_512.FLM', u'size': u'0x80000', u'start': u'0x00000000'}, u'compile': [u'Device/Include/LPC17xx.h', u'LPC175x_6x'], u'debug': u'SVD/LPC176x5x.svd', u'pdsc_file': u'http://www.keil.com/pack/Keil.LPC1700_DFP.pdsc', u'memory': {u'IRAM1': {u'size': u'0x8000', u'start': u'0x10000000'}, u'IRAM2': {u'size': u'0x8000', u'start': u'0x2007C000'}, u'IROM1': {u'size': u'0x80000', u'start': u'0x00000000'}}}
Definition at line 310 of file arm_pack_manager/__init__.py.
def pack_from_cache | ( | self, | |
device | |||
) |
Low level inteface for extracting a PACK file from the cache. Assumes that the file specified is a PACK file and is in the cache. :param url: The URL of a PACK file. :type url: str :return: A parsed representation of the PACK file. :rtype: ZipFile
Definition at line 422 of file arm_pack_manager/__init__.py.
def pdsc_from_cache | ( | self, | |
url | |||
) |
Low level inteface for extracting a PDSC file from the cache. Assumes that the file specified is a PDSC file and is in the cache. :param url: The URL of a PDSC file. :type url: str :return: A parsed representation of the PDSC file. :rtype: BeautifulSoup
Definition at line 408 of file arm_pack_manager/__init__.py.
def pdsc_to_pack | ( | self, | |
url | |||
) |
Find the URL of the specified pack file described by a PDSC. The PDSC is assumed to be cached and is looked up in the cache by its URL. :param url: The url used to look up the PDSC. :type url: str :return: The url of the PACK file. :rtype: str
Definition at line 106 of file arm_pack_manager/__init__.py.
Generated on Sun Jul 17 2022 08:25:44 by
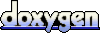