
Rtos API example
Queue< T, queue_sz > Class Template Reference
[EventFlags class]
The Queue class allow to control, send, receive, or wait for messages. More...
#include <Queue.h>
Inherits NonCopyable< Queue< T, queue_sz > >.
Public Member Functions | |
Queue () | |
Create and initialize a message Queue. | |
bool | empty () const |
Check if the queue is empty. | |
bool | full () const |
Check if the queue is full. | |
osStatus | put (T *data, uint32_t millisec=0, uint8_t prio=0) |
Put a message in a Queue. | |
osEvent | get (uint32_t millisec=osWaitForever) |
Get a message or Wait for a message from a Queue. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
template<typename T, uint32_t queue_sz>
class rtos::Queue< T, queue_sz >
The Queue class allow to control, send, receive, or wait for messages.
A message can be a integer or pointer value to a certain type T that is send to a thread or interrupt service routine.
- Template Parameters:
-
T data type of a single message element. queue_sz maximum number of messages in queue.
- Note:
- Memory considerations: The queue control structures will be created on current thread's stack, both for the mbed OS and underlying RTOS objects (static or dynamic RTOS memory pools are not being used).
Definition at line 53 of file Queue.h.
Constructor & Destructor Documentation
Member Function Documentation
bool empty | ( | ) | const |
bool full | ( | ) | const |
osEvent get | ( | uint32_t | millisec = osWaitForever ) |
Get a message or Wait for a message from a Queue.
Messages are retrieved in a descending priority order or first in first out when the priorities are the same.
- Parameters:
-
millisec timeout value or 0 in case of no time-out. (default: osWaitForever).
- Returns:
- event information that includes the message in event.value and the status code in event.status: osEventMessage message received. osOK no message is available in the queue and no timeout was specified. osEventTimeout no message has arrived during the given timeout period. osErrorParameter a parameter is invalid or outside of a permitted range.
osStatus put | ( | T * | data, |
uint32_t | millisec = 0 , |
||
uint8_t | prio = 0 |
||
) |
Put a message in a Queue.
- Parameters:
-
data message pointer. millisec timeout value or 0 in case of no time-out. (default: 0) prio priority value or 0 in case of default. (default: 0)
- Returns:
- status code that indicates the execution status of the function: osOK the message has been put into the queue. osErrorTimeout the message could not be put into the queue in the given time. osErrorResource not enough space in the queue. osErrorParameter internal error or non-zero timeout specified in an ISR.
Generated on Sun Jul 17 2022 08:25:44 by
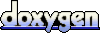