
Rtos API example
EventFlags Class Reference
[EventFlags class]
The EventFlags class is used to signal or wait for an arbitrary event or events. More...
#include <EventFlags.h>
Inherits NonCopyable< EventFlags >.
Public Member Functions | |
EventFlags () | |
Create and Initialize a EventFlags object. | |
EventFlags (const char *name) | |
Create and Initialize a EventFlags object. | |
uint32_t | set (uint32_t flags) |
Set the specified Event Flags. | |
uint32_t | clear (uint32_t flags=0x7fffffff) |
Clear the specified Event Flags. | |
uint32_t | get () const |
Get the currently set Event Flags. | |
uint32_t | wait_all (uint32_t flags=0, uint32_t timeout=osWaitForever, bool clear=true) |
Wait for all of the specified event flags to become signaled. | |
uint32_t | wait_any (uint32_t flags=0, uint32_t timeout=osWaitForever, bool clear=true) |
Wait for any of the specified event flags to become signaled. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
The EventFlags class is used to signal or wait for an arbitrary event or events.
- Note:
- EventFlags support 31 flags so the MSB flag is ignored, it is used to return an error code (osFlagsError)
- Memory considerations: The EventFlags control structures will be created on current thread's stack, both for the mbed OS and underlying RTOS objects (static or dynamic RTOS memory pools are not being used).
Definition at line 47 of file EventFlags.h.
Constructor & Destructor Documentation
EventFlags | ( | ) |
Create and Initialize a EventFlags object.
Definition at line 29 of file EventFlags.cpp.
EventFlags | ( | const char * | name ) |
Create and Initialize a EventFlags object.
- Parameters:
-
name name to be used for this EventFlags. It has to stay allocated for the lifetime of the thread.
Definition at line 34 of file EventFlags.cpp.
Member Function Documentation
uint32_t clear | ( | uint32_t | flags = 0x7fffffff ) |
Clear the specified Event Flags.
- Parameters:
-
flags specifies the flags that shall be cleared. (default: 0x7fffffff - all flags)
- Returns:
- event flags before clearing or error code if highest bit set (osFlagsError).
Definition at line 55 of file EventFlags.cpp.
uint32_t get | ( | ) | const |
Get the currently set Event Flags.
- Returns:
- set event flags.
Definition at line 60 of file EventFlags.cpp.
uint32_t set | ( | uint32_t | flags ) |
Set the specified Event Flags.
- Parameters:
-
flags specifies the flags that shall be set.
- Returns:
- event flags after setting or error code if highest bit set (osFlagsError).
Definition at line 50 of file EventFlags.cpp.
uint32_t wait_all | ( | uint32_t | flags = 0 , |
uint32_t | timeout = osWaitForever , |
||
bool | clear = true |
||
) |
Wait for all of the specified event flags to become signaled.
- Parameters:
-
flags specifies the flags to wait for. timeout timeout value or 0 in case of no time-out. (default: osWaitForever) clear specifies wether to clear the flags after waiting for them. (default: true)
- Returns:
- event flags before clearing or error code if highest bit set (osFlagsError).
Definition at line 65 of file EventFlags.cpp.
uint32_t wait_any | ( | uint32_t | flags = 0 , |
uint32_t | timeout = osWaitForever , |
||
bool | clear = true |
||
) |
Wait for any of the specified event flags to become signaled.
- Parameters:
-
flags specifies the flags to wait for. (default: 0) timeout timeout value or 0 in case of no time-out. (default: osWaitForever) clear specifies wether to clear the flags after waiting for them. (default: true)
- Returns:
- event flags before clearing or error code if highest bit set (osFlagsError).
Definition at line 70 of file EventFlags.cpp.
Generated on Sun Jul 17 2022 08:25:44 by
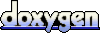