
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
EventFlags.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2017 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef EVENT_FLAG_H 00023 #define EVENT_FLAG_H 00024 00025 #include <stdint.h> 00026 #include "cmsis_os2.h" 00027 #include "mbed_rtos1_types.h" 00028 #include "mbed_rtos_storage.h" 00029 00030 #include "platform/NonCopyable.h" 00031 00032 namespace rtos { 00033 /** \addtogroup rtos */ 00034 /** @{*/ 00035 /** 00036 * \defgroup rtos_EventFlags EventFlags class 00037 * @{ 00038 */ 00039 00040 /** The EventFlags class is used to signal or wait for an arbitrary event or events. 00041 @note 00042 EventFlags support 31 flags so the MSB flag is ignored, it is used to return an error code (@a osFlagsError) 00043 @note 00044 Memory considerations: The EventFlags control structures will be created on current thread's stack, both for the mbed OS 00045 and underlying RTOS objects (static or dynamic RTOS memory pools are not being used). 00046 */ 00047 class EventFlags : private mbed::NonCopyable<EventFlags> { 00048 public: 00049 /** Create and Initialize a EventFlags object */ 00050 EventFlags(); 00051 00052 /** Create and Initialize a EventFlags object 00053 00054 @param name name to be used for this EventFlags. It has to stay allocated for the lifetime of the thread. 00055 */ 00056 EventFlags(const char *name); 00057 00058 /** Set the specified Event Flags. 00059 @param flags specifies the flags that shall be set. 00060 @return event flags after setting or error code if highest bit set (@a osFlagsError). 00061 */ 00062 uint32_t set(uint32_t flags); 00063 00064 /** Clear the specified Event Flags. 00065 @param flags specifies the flags that shall be cleared. (default: 0x7fffffff - all flags) 00066 @return event flags before clearing or error code if highest bit set (@a osFlagsError). 00067 */ 00068 uint32_t clear(uint32_t flags = 0x7fffffff); 00069 00070 /** Get the currently set Event Flags. 00071 @return set event flags. 00072 */ 00073 uint32_t get() const; 00074 00075 /** Wait for all of the specified event flags to become signaled. 00076 @param flags specifies the flags to wait for. 00077 @param timeout timeout value or 0 in case of no time-out. (default: osWaitForever) 00078 @param clear specifies wether to clear the flags after waiting for them. (default: true) 00079 @return event flags before clearing or error code if highest bit set (@a osFlagsError). 00080 */ 00081 uint32_t wait_all(uint32_t flags = 0, uint32_t timeout = osWaitForever, bool clear = true); 00082 00083 /** Wait for any of the specified event flags to become signaled. 00084 @param flags specifies the flags to wait for. (default: 0) 00085 @param timeout timeout value or 0 in case of no time-out. (default: osWaitForever) 00086 @param clear specifies wether to clear the flags after waiting for them. (default: true) 00087 @return event flags before clearing or error code if highest bit set (@a osFlagsError). 00088 */ 00089 uint32_t wait_any(uint32_t flags = 0, uint32_t timeout = osWaitForever, bool clear = true); 00090 00091 ~EventFlags(); 00092 00093 private: 00094 void constructor(const char *name = NULL); 00095 uint32_t wait(uint32_t flags, uint32_t opt, uint32_t timeout, bool clear); 00096 osEventFlagsId_t _id; 00097 mbed_rtos_storage_event_flags_t _obj_mem; 00098 }; 00099 00100 /** @}*/ 00101 /** @}*/ 00102 00103 } 00104 #endif 00105
Generated on Sun Jul 17 2022 08:25:22 by
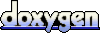