
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
BlockDevice.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_BLOCK_DEVICE_H 00018 #define MBED_BLOCK_DEVICE_H 00019 00020 #include <stdint.h> 00021 00022 00023 /** Enum of standard error codes 00024 * 00025 * @enum bd_error 00026 */ 00027 enum bd_error { 00028 BD_ERROR_OK = 0, /*!< no error */ 00029 BD_ERROR_DEVICE_ERROR = -4001, /*!< device specific error */ 00030 }; 00031 00032 /** Type representing the address of a specific block 00033 */ 00034 typedef uint64_t bd_addr_t; 00035 00036 /** Type representing a quantity of 8-bit bytes 00037 */ 00038 typedef uint64_t bd_size_t; 00039 00040 00041 /** A hardware device capable of writing and reading blocks 00042 */ 00043 class BlockDevice 00044 { 00045 public: 00046 /** Lifetime of a block device 00047 */ 00048 virtual ~BlockDevice() {}; 00049 00050 /** Initialize a block device 00051 * 00052 * @return 0 on success or a negative error code on failure 00053 */ 00054 virtual int init() = 0; 00055 00056 /** Deinitialize a block device 00057 * 00058 * @return 0 on success or a negative error code on failure 00059 */ 00060 virtual int deinit() = 0; 00061 00062 /** Read blocks from a block device 00063 * 00064 * If a failure occurs, it is not possible to determine how many bytes succeeded 00065 * 00066 * @param buffer Buffer to write blocks to 00067 * @param addr Address of block to begin reading from 00068 * @param size Size to read in bytes, must be a multiple of read block size 00069 * @return 0 on success, negative error code on failure 00070 */ 00071 virtual int read(void *buffer, bd_addr_t addr, bd_size_t size) = 0; 00072 00073 /** Program blocks to a block device 00074 * 00075 * The blocks must have been erased prior to being programmed 00076 * 00077 * If a failure occurs, it is not possible to determine how many bytes succeeded 00078 * 00079 * @param buffer Buffer of data to write to blocks 00080 * @param addr Address of block to begin writing to 00081 * @param size Size to write in bytes, must be a multiple of program block size 00082 * @return 0 on success, negative error code on failure 00083 */ 00084 virtual int program(const void *buffer, bd_addr_t addr, bd_size_t size) = 0; 00085 00086 /** Erase blocks on a block device 00087 * 00088 * The state of an erased block is undefined until it has been programmed 00089 * 00090 * @param addr Address of block to begin erasing 00091 * @param size Size to erase in bytes, must be a multiple of erase block size 00092 * @return 0 on success, negative error code on failure 00093 */ 00094 virtual int erase(bd_addr_t addr, bd_size_t size) 00095 { 00096 return 0; 00097 } 00098 00099 /** Mark blocks as no longer in use 00100 * 00101 * This function provides a hint to the underlying block device that a region of blocks 00102 * is no longer in use and may be erased without side effects. Erase must still be called 00103 * before programming, but trimming allows flash-translation-layers to schedule erases when 00104 * the device is not busy. 00105 * 00106 * @param addr Address of block to mark as unused 00107 * @param size Size to mark as unused in bytes, must be a multiple of erase block size 00108 * @return 0 on success, negative error code on failure 00109 */ 00110 virtual int trim(bd_addr_t addr, bd_size_t size) 00111 { 00112 return 0; 00113 } 00114 00115 /** Get the size of a readable block 00116 * 00117 * @return Size of a readable block in bytes 00118 */ 00119 virtual bd_size_t get_read_size() const = 0; 00120 00121 /** Get the size of a programable block 00122 * 00123 * @return Size of a programable block in bytes 00124 * @note Must be a multiple of the read size 00125 */ 00126 virtual bd_size_t get_program_size() const = 0; 00127 00128 /** Get the size of a eraseable block 00129 * 00130 * @return Size of a eraseable block in bytes 00131 * @note Must be a multiple of the program size 00132 */ 00133 virtual bd_size_t get_erase_size() const 00134 { 00135 return get_program_size(); 00136 } 00137 00138 /** Get the total size of the underlying device 00139 * 00140 * @return Size of the underlying device in bytes 00141 */ 00142 virtual bd_size_t size() const = 0; 00143 00144 /** Convenience function for checking block read validity 00145 * 00146 * @param addr Address of block to begin reading from 00147 * @param size Size to read in bytes 00148 * @return True if read is valid for underlying block device 00149 */ 00150 bool is_valid_read(bd_addr_t addr, bd_size_t size) const 00151 { 00152 return ( 00153 addr % get_read_size() == 0 && 00154 size % get_read_size() == 0 && 00155 addr + size <= this->size()); 00156 } 00157 00158 /** Convenience function for checking block program validity 00159 * 00160 * @param addr Address of block to begin writing to 00161 * @param size Size to write in bytes 00162 * @return True if program is valid for underlying block device 00163 */ 00164 bool is_valid_program(bd_addr_t addr, bd_size_t size) const 00165 { 00166 return ( 00167 addr % get_program_size() == 0 && 00168 size % get_program_size() == 0 && 00169 addr + size <= this->size()); 00170 } 00171 00172 /** Convenience function for checking block erase validity 00173 * 00174 * @param addr Address of block to begin erasing 00175 * @param size Size to erase in bytes 00176 * @return True if erase is valid for underlying block device 00177 */ 00178 bool is_valid_erase(bd_addr_t addr, bd_size_t size) const 00179 { 00180 return ( 00181 addr % get_erase_size() == 0 && 00182 size % get_erase_size() == 0 && 00183 addr + size <= this->size()); 00184 } 00185 }; 00186 00187 00188 #endif
Generated on Sun Jul 17 2022 08:25:20 by
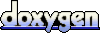