
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ccmLIB.h
Go to the documentation of this file.
00001 /* 00002 * Copyright (c) 2014-2016 ARM Limited. All rights reserved. 00003 * 00004 * SPDX-License-Identifier: LicenseRef-PBL 00005 * 00006 * Licensed under the Permissive Binary License, Version 1.0 (the "License"); you may not use this file except in compliance with the License. 00007 * You may obtain a copy of the License at 00008 * 00009 * https://www.mbed.com/licenses/PBL-1.0 00010 * 00011 * See the License for the specific language governing permissions and limitations under the License. 00012 * 00013 */ 00014 #ifndef CCMLIB_H_ 00015 #define CCMLIB_H_ 00016 00017 #include "ns_types.h" 00018 00019 /** 00020 * 00021 * \file ccmLIB.h 00022 * \brief CCM Library API. 00023 * 00024 * \section ccm-api CCM Library API: 00025 * - ccm_sec_init(), A function to init CCM library. 00026 * - ccm_process_run(), A function to run configured CCM process. 00027 * 00028 * \section ccm-instruction CCM process sequence: 00029 * 1. Init CCM library by, ccm key, ccm_sec_init() 00030 * - security level 00031 * - 128-bit CCM key 00032 * - mode: AES_CCM_ENCRYPT or AES_CCM_DECRYPT 00033 * - CCM L parameter: 2 or 3 depending on the nonce length (802.15.4 use 2 and TLS security use 3) 00034 * 2. Define ADATA pointer and length, if returned global structure mic_len field is > 0 00035 * 3. Set data pointer and length 00036 * 4. Do configured CCM process ccm_process_run() 00037 * 5. Check return value: 00038 * -If 0 Process ok 00039 * -< 0 MIC fail or parameter fail 00040 * 00041 * \section ccm-mutex CCM Mutex for Multi Thread System 00042 * If you are running a multi thread system and the CCM library will be used for multiple thread, do the following: 00043 * 1. Add compiler flag to library build process CCM_USE_MUTEX. 00044 * 2. Define OS-specific mutex at the application. 00045 * 3. Implement arm_ccm_mutex_lock() arm_ccm_mutex_unlock() function for using the generated and initialized mutex. 00046 */ 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 #define AES_NO_SECURITY 0x00 /**< No security */ 00051 #define AES_SECURITY_LEVEL_MIC32 0x01 /**< MIC32 */ 00052 #define AES_SECURITY_LEVEL_MIC64 0x02 /**< MIC64 */ 00053 #define AES_SECURITY_LEVEL_MIC128 0x03 /**< MIC128 */ 00054 #define AES_SECURITY_LEVEL_ENC 0x04 /**< ENC */ 00055 #define AES_SECURITY_LEVEL_ENC_MIC32 0x05 /**< ENC_MIC32 */ 00056 #define AES_SECURITY_LEVEL_ENC_MIC64 0x06 /**< ENC_MIC64 */ 00057 #define AES_SECURITY_LEVEL_ENC_MIC128 0x07 /**< ENC_MIC128 */ 00058 00059 #define AES_CCM_ENCRYPT 0x00 /**< Encryption mode */ 00060 #define AES_CCM_DECRYPT 0x01 /**< Decryption mode */ 00061 00062 00063 /** 00064 * \brief A function for locking CCM mutex if the OS is multi thread. If you are using single thread create an empty function. 00065 */ 00066 extern void arm_ccm_mutex_lock(void); 00067 /** 00068 * \brief A function for unlocking CCM mutex if the OS is multi thread. If you are using single thread create an empty function 00069 */ 00070 extern void arm_ccm_mutex_unlock(void); 00071 00072 /*! 00073 * \struct ccm_globals_t 00074 * \brief CCM global structure. 00075 * The structure is used for configuring NONCE, adata and data before calling ccm_process_run(). 00076 */ 00077 typedef struct { 00078 uint8_t exp_nonce[15]; /**< CCM NONCE buffer Nonce. */ 00079 uint8_t *data_ptr; /**< Pointer to data IN. */ 00080 uint16_t data_len; /**< Length of data IN. */ 00081 const uint8_t *adata_ptr; /**< Pointer to authentication data. */ 00082 uint16_t adata_len; /**< Length of authentication data. */ 00083 uint8_t mic_len; /**< ccm_sec_init() sets here the length of MIC. */ 00084 uint8_t *mic; /**< Encrypt process writes MIC. Decrypt reads it and compares it with the MIC obtained from data. */ 00085 } ccm_globals_t; 00086 00087 /** 00088 * \brief A function to initialize the CCM library. 00089 * \param sec_level Used CCM security level (0-7). 00090 * \param ccm_key Pointer to 128-key. 00091 * \param mode AES_CCM_ENCRYPT or AES_CCM_DECRYPT. 00092 * \param ccm_l Can be 2 or 3. 2 when NONCE length is 13 and 3 when 12. (NONCE length = (15-ccm_l)) 00093 * 00094 * \return Pointer to Global CCM parameter buffer. 00095 * \return 0 When parameter fails or CCM is busy. 00096 */ 00097 extern ccm_globals_t *ccm_sec_init(uint8_t sec_level, const uint8_t *ccm_key, uint8_t mode, uint8_t ccm_l); 00098 00099 /** 00100 * \brief A function to run the configured CCM process. 00101 * When AES_CCM_ENCRYPT mode is selected and MIC is needed, the library saves MIC right after the encrypted data. 00102 * \param ccm_params CCM parameters 00103 * 00104 * \return 0 CCM process OK and when AES_CCM_DECRYPT mode was selected also MIC was correct. 00105 * \return -1 Init not called or data or adata pointers or lengths are zero. 00106 * \return -2 Null pointer given to function. 00107 */ 00108 extern int8_t ccm_process_run(ccm_globals_t *ccm_params); 00109 #ifdef __cplusplus 00110 } 00111 #endif 00112 00113 #endif /* CCMLIB_H_ */
Generated on Sun Jul 17 2022 08:25:20 by
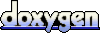