
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
Timer.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "drivers/Timer.h" 00017 #include "hal/ticker_api.h" 00018 #include "hal/us_ticker_api.h" 00019 #include "platform/mbed_critical.h" 00020 #include "hal/lp_ticker_api.h" 00021 00022 namespace mbed { 00023 00024 Timer::Timer() : _running(), _start(), _time(), _ticker_data(get_us_ticker_data()), _lock_deepsleep(true) { 00025 reset(); 00026 } 00027 00028 Timer::Timer(const ticker_data_t *data) : _running(), _start(), _time(), _ticker_data(data), _lock_deepsleep(true) { 00029 reset(); 00030 #if DEVICE_LOWPOWERTIMER 00031 _lock_deepsleep = (data != get_lp_ticker_data()); 00032 #endif 00033 } 00034 00035 Timer::~Timer() { 00036 core_util_critical_section_enter(); 00037 if (_running) { 00038 if(_lock_deepsleep) { 00039 sleep_manager_unlock_deep_sleep(); 00040 } 00041 } 00042 _running = 0; 00043 core_util_critical_section_exit(); 00044 } 00045 00046 void Timer::start() { 00047 core_util_critical_section_enter(); 00048 if (!_running) { 00049 if(_lock_deepsleep) { 00050 sleep_manager_lock_deep_sleep(); 00051 } 00052 _start = ticker_read_us(_ticker_data); 00053 _running = 1; 00054 } 00055 core_util_critical_section_exit(); 00056 } 00057 00058 void Timer::stop() { 00059 core_util_critical_section_enter(); 00060 _time += slicetime(); 00061 if (_running) { 00062 if(_lock_deepsleep) { 00063 sleep_manager_unlock_deep_sleep(); 00064 } 00065 } 00066 _running = 0; 00067 core_util_critical_section_exit(); 00068 } 00069 00070 int Timer::read_us() { 00071 return read_high_resolution_us(); 00072 } 00073 00074 float Timer::read() { 00075 return (float)read_us() / 1000000.0f; 00076 } 00077 00078 int Timer::read_ms() { 00079 return read_high_resolution_us() / 1000; 00080 } 00081 00082 us_timestamp_t Timer::read_high_resolution_us() { 00083 core_util_critical_section_enter(); 00084 us_timestamp_t time = _time + slicetime(); 00085 core_util_critical_section_exit(); 00086 return time; 00087 } 00088 00089 us_timestamp_t Timer::slicetime() { 00090 us_timestamp_t ret = 0; 00091 core_util_critical_section_enter(); 00092 if (_running) { 00093 ret = ticker_read_us(_ticker_data) - _start; 00094 } 00095 core_util_critical_section_exit(); 00096 return ret; 00097 } 00098 00099 void Timer::reset() { 00100 core_util_critical_section_enter(); 00101 _start = ticker_read_us(_ticker_data); 00102 _time = 0; 00103 core_util_critical_section_exit(); 00104 } 00105 00106 Timer::operator float() { 00107 return read(); 00108 } 00109 00110 } // namespace mbed
Generated on Sun Jul 17 2022 08:25:32 by
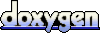