
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
ServiceDiscovery.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MBED_BLE_SERVICE_DISOVERY_H__ 00018 #define MBED_BLE_SERVICE_DISOVERY_H__ 00019 00020 #include "UUID.h " 00021 #include "Gap.h" 00022 #include "GattAttribute.h" 00023 00024 class DiscoveredService; 00025 class DiscoveredCharacteristic; 00026 00027 /** 00028 * @addtogroup ble 00029 * @{ 00030 * @addtogroup gatt 00031 * @{ 00032 * @addtogroup client 00033 * @{ 00034 */ 00035 00036 /** 00037 * Host callback types needed by the service discovery procedure. 00038 * 00039 * This class is also an interface that may be used in vendor port to model 00040 * the service discovery process. This interface is not used in user code. 00041 * 00042 * @important Implementing this interface is not a requirement for the 00043 * implementation of the service discover process. 00044 */ 00045 class ServiceDiscovery { 00046 public: 00047 /** 00048 * Service discovered event handler. 00049 * 00050 * The callback accepts a pointer to a DiscoveredService as parameter. 00051 * 00052 * @important The argument passed to the callback may not persist after the 00053 * callback invocation; therefore, the callbacks must make a shallow copy 00054 * of the DiscoveredService passed as parameter to access its value beyond 00055 * the callback scope. 00056 */ 00057 typedef FunctionPointerWithContext<const DiscoveredService *> 00058 ServiceCallback_t; 00059 00060 /** 00061 * Characteristic discovered event handler. 00062 * 00063 * The callback accepts a pointer to a DiscoveredCharacteristic as 00064 * parameter. 00065 * 00066 * @important The argument passed to the callback may not persist after the 00067 * callback invocation; therefore, the callbacks must make a shallow copy 00068 * of the DiscoveredCharacteristic passed as parameter to access its value 00069 * beyond the callback scope. 00070 */ 00071 typedef FunctionPointerWithContext<const DiscoveredCharacteristic *> 00072 CharacteristicCallback_t; 00073 00074 /** 00075 * Service discovery ended event. 00076 * 00077 * The callback accepts a connection handle as parameter. This 00078 * parameter is used to identify on which connection the service discovery 00079 * process ended. 00080 */ 00081 typedef FunctionPointerWithContext<Gap::Handle_t> TerminationCallback_t; 00082 00083 public: 00084 /** 00085 * Launch service discovery. Once launched, service discovery remains 00086 * active with callbacks being issued back into the application for matching 00087 * services or characteristics. isActive() can be used to determine status, and 00088 * a termination callback (if set up) is invoked at the end. Service 00089 * discovery can be terminated prematurely, if needed, using terminate(). 00090 * 00091 * @param connectionHandle 00092 * Handle for the connection with the peer. 00093 * @param sc 00094 * This is the application callback for a matching service. Taken as 00095 * NULL by default. Note: service discovery may still be active 00096 * when this callback is issued; calling asynchronous BLE-stack 00097 * APIs from within this application callback might cause the 00098 * stack to abort service discovery. If this becomes an issue, it 00099 * may be better to make a local copy of the discoveredService and 00100 * wait for service discovery to terminate before operating on the 00101 * service. 00102 * @param cc 00103 * This is the application callback for a matching characteristic. 00104 * Taken as NULL by default. Note: service discovery may still be 00105 * active when this callback is issued; calling asynchronous 00106 * BLE-stack APIs from within this application callback might cause 00107 * the stack to abort service discovery. If this becomes an issue, 00108 * it may be better to make a local copy of the discoveredCharacteristic 00109 * and wait for service discovery to terminate before operating on the 00110 * characteristic. 00111 * @param matchingServiceUUID 00112 * UUID-based filter for specifying a service in which the application is 00113 * interested. By default, it is set as the wildcard UUID_UNKNOWN, 00114 * in which case it matches all services. If characteristic-UUID 00115 * filter (below) is set to the wildcard value, then a service 00116 * callback is invoked for the matching service (or for every 00117 * service if the service filter is a wildcard). 00118 * @param matchingCharacteristicUUIDIn 00119 * UUID-based filter for specifying a characteristic in which the application 00120 * is interested. By default, it is set as the wildcard UUID_UKNOWN 00121 * to match against any characteristic. If both service-UUID 00122 * filter and characteristic-UUID filter are used with nonwildcard 00123 * values, then only a single characteristic callback is 00124 * invoked for the matching characteristic. 00125 * 00126 * @note Using wildcard values for both service-UUID and characteristic- 00127 * UUID result in complete service discovery: callbacks being 00128 * called for every service and characteristic. 00129 * 00130 * @note Providing NULL for the characteristic callback results in 00131 * characteristic discovery being skipped for each matching 00132 * service. This allows for an inexpensive method to discover only 00133 * services. 00134 * 00135 * @return 00136 * BLE_ERROR_NONE if service discovery is launched successfully; else an appropriate error. 00137 */ 00138 virtual ble_error_t launch(Gap::Handle_t connectionHandle, 00139 ServiceCallback_t sc = NULL, 00140 CharacteristicCallback_t cc = NULL, 00141 const UUID &matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN), 00142 const UUID &matchingCharacteristicUUIDIn = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN)) = 0; 00143 00144 /** 00145 * Check whether service-discovery is currently active. 00146 */ 00147 virtual bool isActive(void) const = 0; 00148 00149 /** 00150 * Terminate an ongoing service discovery. This should result in an 00151 * invocation of the TerminationCallback if service discovery is active. 00152 */ 00153 virtual void terminate(void) = 0; 00154 00155 /** 00156 * Set up a callback to be invoked when service discovery is terminated. 00157 */ 00158 virtual void onTermination(TerminationCallback_t callback) = 0; 00159 00160 /** 00161 * Clear all ServiceDiscovery state of the associated object. 00162 * 00163 * This function is meant to be overridden in the platform-specific 00164 * subclass. Nevertheless, the subclass is only expected to reset its 00165 * state and not the data held in ServiceDiscovery members. This is 00166 * achieved by a call to ServiceDiscovery::reset() from the subclass' 00167 * reset() implementation. 00168 * 00169 * @return BLE_ERROR_NONE on success. 00170 */ 00171 virtual ble_error_t reset(void) { 00172 connHandle = 0; 00173 matchingServiceUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN); 00174 serviceCallback = NULL; 00175 matchingCharacteristicUUID = UUID::ShortUUIDBytes_t(BLE_UUID_UNKNOWN); 00176 characteristicCallback = NULL; 00177 00178 return BLE_ERROR_NONE; 00179 } 00180 00181 protected: 00182 /** 00183 * Connection handle as provided by the SoftDevice. 00184 */ 00185 Gap::Handle_t connHandle; 00186 /** 00187 * UUID-based filter that specifies the service that the application is 00188 * interested in. 00189 */ 00190 UUID matchingServiceUUID; 00191 /** 00192 * The registered callback handle for when a matching service is found 00193 * during service-discovery. 00194 */ 00195 ServiceCallback_t serviceCallback; 00196 /** 00197 * UUID-based filter that specifies the characteristic that the 00198 * application is interested in. 00199 */ 00200 UUID matchingCharacteristicUUID; 00201 /** 00202 * The registered callback handler for when a matching characteristic is 00203 * found during service-discovery. 00204 */ 00205 CharacteristicCallback_t characteristicCallback; 00206 }; 00207 00208 /** 00209 * @} 00210 * @} 00211 * @} 00212 */ 00213 00214 #endif /* ifndef MBED_BLE_SERVICE_DISOVERY_H__ */
Generated on Sun Jul 17 2022 08:25:30 by
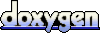