
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
PalGattClient.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017-2017 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef BLE_PAL_GATT_CLIENT_H_ 00018 #define BLE_PAL_GATT_CLIENT_H_ 00019 00020 #include "ble/UUID.h " 00021 #include "ble/BLETypes.h" 00022 #include "ble/ArrayView.h " 00023 #include "ble/blecommon.h" 00024 00025 #include "platform/Callback.h" 00026 00027 #include "AttServerMessage.h" 00028 00029 namespace ble { 00030 namespace pal { 00031 00032 /** 00033 * Adaptation layer for a GATT client. 00034 * 00035 * Define the primitive necessary to implement a proper GATT client. These 00036 * primitives are sometime GATT procedure, ATT procedure or a bit of both. 00037 * 00038 * In general, discovery procedures follow strictly GATT formulation while 00039 * attribute manipulation procedures (read/write) follow the ATT formulation 00040 * to avoid multiple identical implementation for characteristic values and 00041 * characteristic descriptors value, it also fill a hole leave by the 00042 * specification which doesn't define any GATT procedure to get the UUID of an 00043 * included service with a 128 bit UUID. 00044 * 00045 * Complementary informations around ATT procedures can be found in the Section 00046 * 3.4 of the Vol3, Part F of the Bluetooth specification while more informations 00047 * around the GATT procedures can be found in the Section 4 of the Vol 3, Part 00048 * G of the Bluetooth specification. 00049 * 00050 * Complete and compliant Gatt Client used by developer is realized at an higher 00051 * level using the primitives defined in this adaptation layer. 00052 * 00053 * If a stack expose the complete ATT layer then it is possible to provide an 00054 * implementation for GattClient by subclassing the AttClient class and use 00055 * the class AttClientToGattClientAdapter 00056 */ 00057 class GattClient { 00058 00059 public: 00060 /** 00061 * Initialisation of the instance. An implementation can use this function 00062 * to initialise the subsystems needed to realize the operations of this 00063 * interface. 00064 * 00065 * This function has to be called before any other operations. 00066 * 00067 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00068 * appropriate error otherwise. 00069 */ 00070 virtual ble_error_t initialize() = 0; 00071 00072 /** 00073 * Termination of the instance. An implementation can use this function 00074 * to release the subsystems initialised to realise the operations of 00075 * this interface. 00076 * 00077 * After a call to this function, initialise should be called again to 00078 * allow usage of the interface. 00079 * 00080 * @return BLE_ERROR_NONE if the request has been successfully sent or the 00081 * appropriate error otherwise. 00082 */ 00083 virtual ble_error_t terminate() = 0; 00084 00085 /** 00086 * Negotiate the mtu to use by this connection. 00087 * First the client send to the server the maximum rx mtu that it can receive 00088 * then the client reply with the maximum rx mtu it can receive. 00089 * The mtu chosen for the connection is the minimum of the client Rx mtu 00090 * and server Rx mtu values. 00091 * 00092 * If an error occurred then the mtu used remains the default value. 00093 * 00094 * The server will reply to this request with an AttExchangeMTUResponse in 00095 * case of success or AttErrorResponse in case of failure. 00096 * 00097 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.3.1 00098 * 00099 * @param connection The handle of the connection to send this request to. 00100 * @return BLE_ERROR_NONE or an appropriate error. 00101 */ 00102 virtual ble_error_t exchange_mtu(connection_handle_t connection) = 0; 00103 00104 /** 00105 * Acquire the size of the mtu for a given connection. 00106 * 00107 * @param connection The handle of the connection for which the the MTU size 00108 * should be acquired. 00109 * 00110 * @param mtu_size Output parameter which will contain the MTU size. 00111 * 00112 * @return BLE_ERROR_NONE if the MTU size has been acquired or the 00113 * appropriate error otherwise. 00114 */ 00115 virtual ble_error_t get_mtu_size( 00116 connection_handle_t connection_handle, 00117 uint16_t& mtu_size 00118 ) = 0; 00119 00120 /** 00121 * Discover primary services in the range [begin - 0xFFFF]. 00122 * 00123 * If services exists in the range provided, the server will reply with a 00124 * ReadByGoupType response where for each attribute_data exposed: 00125 * - attribute_handle is the service attribute handle 00126 * - end_group_handle is the last handle of the service 00127 * - attribute_value is the UUID of the service. 00128 * 00129 * If the end of the range is not reached, this procedure can be relaunched 00130 * with the last handle of the last service discovered plus one as the 00131 * beginning of the discovery range. 00132 * 00133 * If there is not services left to discover in the range, the server can 00134 * either: 00135 * * Reply with an ErrorResponse with the Error code set to ATTRIBUTE_NOT_FOUND 00136 * * Set the end handle of the last service to 0xFFFF. 00137 * 00138 * @note This function realize a partial implementation of the Discover All 00139 * Primary Services procedure. The complete implementation of the procedure 00140 * is realized at an higher level. 00141 * @note This function issue a Read By Group Type ATT request where the 00142 * type parameter is equal to Primary Service and the Ending Handle parameter 00143 * is equal to 0xFFFF. 00144 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.4.1 00145 * 00146 * @param connection The handle of the connection to send this request to. 00147 * @param begin The beginning of the discovery range. 00148 * 00149 * @return BLE_ERROR_NONE or an appropriate error. 00150 */ 00151 virtual ble_error_t discover_primary_service( 00152 connection_handle_t connection, 00153 attribute_handle_t discovery_range_begining 00154 ) = 0; 00155 00156 /** 00157 * Discover primary services by UUID in the range [discovery_range_begining - 0xFFFF]. 00158 * 00159 * If services exists in the range provided, the server will reply with a 00160 * FindByTypeValueResponse containing the attribute range of each service 00161 * discovered. 00162 * 00163 * If the end of the range is not reached, this procedure can be relaunched 00164 * with the last handle of the last service discovered plus one as the 00165 * beginning of the discovery range. 00166 * 00167 * If there is not services left to discover in the range, the server can 00168 * either: 00169 * * Reply with an ErrorResponse with the Error code set to ATTRIBUTE_NOT_FOUND 00170 * * Set the end handle of the last service to 0xFFFF. 00171 * 00172 * @note This function realize a partial implementation of the Discover 00173 * Primary Service by Service UUID procedure. The complete implementation of 00174 * the procedure is realized at an higher level. 00175 * @note This function issue a Find By Type Value ATT request where the 00176 * type parameter is equal to Primary Service and the Ending Handle 00177 * parameter is equal to 0xFFFF. 00178 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.4.2 00179 * 00180 * @param connection The handle of the connection to send this request to. 00181 * @param begin The beginning of the discovery range. 00182 * @param uuid The UUID of the service to discover. 00183 * 00184 * @return BLE_ERROR_NONE or an appropriate error. 00185 */ 00186 virtual ble_error_t discover_primary_service_by_service_uuid( 00187 connection_handle_t connection_handle, 00188 attribute_handle_t discovery_range_beginning, 00189 const UUID& uuid 00190 ) = 0; 00191 00192 /** 00193 * Find included services within a service. 00194 * 00195 * If services are included in the service range then the server will reply 00196 * with a ReadByTypeResponse where for each attribute record: 00197 * - attribute_handle The handle where the service is included within the 00198 * service definition. 00199 * - attribute_data Contains two handles defining the range of the included. 00200 * If the service found have a 16 bit uuid then its UUID is also included 00201 * in the attribute data. 00202 * 00203 * If the end of the service range is not reached, this procedure can be 00204 * relaunched with the handle of the last included service discovered plus 00205 * one as the beginning of the new discovery range. 00206 * 00207 * The procedure is complete when either: 00208 * - The server reply with an ErrorResponse with the Error code set to 00209 * ATTRIBUTE_NOT_FOUND 00210 * - An included service handle returned is equal to the end of the range. 00211 * 00212 * If the service UUID is a 128 bits one then it is necessary to issue a read 00213 * attribute request on the first handle of the service discovered to get it. 00214 * 00215 * @note This function realize a partial implementation of the Find Included 00216 * Services procedure. The complete implementation of the procedure is 00217 * realized at an higher level. 00218 * @note This function issue a Read By Type ATT request where the 00219 * type parameter is equal to Include. 00220 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.5.1 00221 * 00222 * @param connection The handle of the connection to send this request to. 00223 * @param service_range The range of the service where service inclusion has 00224 * to be discovered. 00225 * 00226 * @return BLE_ERROR_NONE or an appropriate error. 00227 */ 00228 virtual ble_error_t find_included_service( 00229 connection_handle_t connection_handle, 00230 attribute_handle_range_t service_range 00231 ) = 0; 00232 00233 /** 00234 * Find characteristic declarations within a service definition. 00235 * 00236 * If characteristic declarations are found within the range then the server 00237 * should reply with a ReadByTypeResponse where for each attribute record: 00238 * - attribute_handle is the handle of the characteristic definition 00239 * - attribute_data contains the the following values attached to the 00240 * characteristic : 00241 * + properties: the properties of the characteristic. 00242 * + value handle: the handle of the value of the characteristic. 00243 * + uuid: the UUID of the characteristic. 00244 * 00245 * The procedure is considered complete when the server send an ErrorResponse 00246 * with the ErrorCode set to ATTRIBUTE_NOT_FOUND or a ReadByType response 00247 * has an attribute_handle set to the end of the discovery range. 00248 * 00249 * If the procedure is not complete after a server response, it should be 00250 * relaunched with an updated range starting at the last attribute_handle 00251 * discovered plus one. 00252 * 00253 * @note This function realize a partial implementation of the Discover All 00254 * Characteristics of a Service procedure. The complete implementation of 00255 * the procedure is realized at an higher level. 00256 * @note This function issue a Read By Type ATT request where the type 00257 * parameter is equal to Characteristic. 00258 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.6.1 00259 * 00260 * @note The GATT procedure Discover Characteristics by UUID is implemented 00261 * at a higher level using this function as a base. 00262 * 00263 * @param connection The handle of the connection to send this request to. 00264 * @param discovery_range The range of attributes where the characteristics 00265 * are discovered. It should be contained within a service. 00266 * 00267 * @return BLE_ERROR_NONE or an appropriate error. 00268 */ 00269 virtual ble_error_t discover_characteristics_of_a_service( 00270 connection_handle_t connection_handle, 00271 attribute_handle_range_t discovery_range 00272 ) = 0; 00273 00274 /** 00275 * Discover characteristic descriptors of a characteristic. 00276 * 00277 * If descriptors are found within the range provided then the server should 00278 * reply with a FindInformationResponse containing a list of 00279 * attribute_handle_t, UUID pairs where the attribute handle is the 00280 * descriptor handle and UUID is the UUID of the descriptor. 00281 * 00282 * The procedure is complete when the server send an ErrorResponse with the 00283 * error code ATTRIBUTE_NOT_FOUND or the FindInformationResponse has an 00284 * attribute handle that is equal to the end handle of the discovery range. 00285 * 00286 * @note This function realize a partial implementation of the Discover All 00287 * Characteristics Descriptors procedure. The complete implementation of 00288 * the procedure is realized at an higher level. 00289 * @note This function issue a Find Information ATT request.. 00290 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.7.1 00291 * 00292 * @note It should be possible to use this function to issue a regular 00293 * ATT Find Information Request. 00294 * 00295 * @param connection The handle of the connection to send this request to. 00296 * @param descriptors_discovery_range The range of attributes where the 00297 * descriptors are discovered. The first handle shall be no less than the 00298 * characteristic value handle plus one and the last handle shall be no more 00299 * than the last handle of the characteristic. 00300 * 00301 * @return BLE_ERROR_NONE or an appropriate error. 00302 */ 00303 virtual ble_error_t discover_characteristics_descriptors( 00304 connection_handle_t connection_handle, 00305 attribute_handle_range_t descriptors_discovery_range 00306 ) = 0; 00307 00308 /** 00309 * Read the value of an attribute. 00310 * 00311 * The server will reply with an AttReadResponse. In case of error, the 00312 * server will reply with an AttErrorResponse. 00313 * 00314 * @note This function issue an ATT Read Request. 00315 * 00316 * @note: This function is the base function for the read Characteristic 00317 * Value and Read Characteristic Descriptor GATT procedures. It can also 00318 * be used to read the 128 bit UUID of a service discovered with 00319 * find_included_service. 00320 * 00321 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.8.1 00322 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.12.1 00323 * 00324 * @param connection The handle of the connection to send this request to. 00325 * @param attribute_handle Handle of the attribute to read. 00326 * 00327 * @return BLE_ERROR_NONE or an appropriate error. 00328 */ 00329 virtual ble_error_t read_attribute_value( 00330 connection_handle_t connection_handle, 00331 attribute_handle_t attribute_handle 00332 ) = 0; 00333 00334 /** 00335 * Read a characteristic value using its UUID (type). 00336 * 00337 * The server will respond a ReadByTypeResponse containing a sequence of 00338 * attribute handle and attribute value pairs. 00339 * To read remaining attributes, the client should launch a new request 00340 * with an updated range. 00341 * 00342 * The procedure is considered complete when the server respond with an 00343 * ErrorResponse containing the ErrorCode ATTRIBUTE_NOT_FOUND or when an 00344 * handle in the ReadByTypeResponse is equal to the end of the discovered 00345 * range. 00346 * 00347 * @note This function realize a partial implementation of the Read Using 00348 * Characteristics Characteristic procedure. The complete implementation of 00349 * the procedure is realized at an higher level. 00350 * @note This function issue a Read By Type ATT request. 00351 * @note BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.8.2 00352 * 00353 * @note It should be possible to use this function to issue a regular 00354 * ATT Read By Type Request. 00355 * 00356 * @param connection The handle of the connection to send this request to. 00357 * @param attribute_range Range of the handle where an attribute with 00358 * uuid as type is present. 00359 * @param uuid UUID of the characteristic(s) to read. 00360 * 00361 * @return BLE_ERROR_NONE or an appropriate error. 00362 */ 00363 virtual ble_error_t read_using_characteristic_uuid( 00364 connection_handle_t connection_handle, 00365 attribute_handle_range_t read_range, 00366 const UUID& uuid 00367 ) = 0; 00368 00369 /** 00370 * Read a partial value of an attribute. 00371 * 00372 * The server will respond with a ReadBlobResponse containing the data read 00373 * or an ErrorResponse in case of error. 00374 * 00375 * The procedure is not complete as long as the value in response have the 00376 * same size as the mtu minus one. If the procedure is not complete, it can 00377 * be launch again with an updated offset to read the remaining part of the 00378 * attribute value. 00379 * 00380 * @note This function issue an ATT Read Blob Request. 00381 * 00382 * @note: This function is the base function for the Read Long 00383 * Characteristic Value and Read Long Characteristic Descriptor GATT 00384 * procedures. 00385 * 00386 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.8.3 00387 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.12.2 00388 * 00389 * @param connection_handle The handle of the connection to send this request to. 00390 * @param attribute_handle Handle of the attribute to read. 00391 * @param offset Beginning offset for the read operation. 00392 * 00393 * @return BLE_ERROR_NONE or an appropriate error. 00394 */ 00395 virtual ble_error_t read_attribute_blob( 00396 connection_handle_t connection_handle, 00397 attribute_handle_t attribute_handle, 00398 uint16_t offset 00399 ) = 0; 00400 00401 /** 00402 * Read atomically multiple characteristics values. 00403 * 00404 * The server will respond with a ReadMultiple response containing the 00405 * concatenation of the values of the characteristics. 00406 * 00407 * @note values might be truncated 00408 * 00409 * In case of error, the server should respond a with an ErrorResponse. 00410 * 00411 * @note This function issue an ATT Read Multiple Request. 00412 * 00413 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.8.4 00414 * 00415 * @param connection_handle The handle of the connection to send this request to. 00416 * @param characteristic_value_handles Handle of the characteristic values 00417 * to read. 00418 * 00419 * @return BLE_ERROR_NONE or an appropriate error. 00420 */ 00421 virtual ble_error_t read_multiple_characteristic_values( 00422 connection_handle_t connection_handle, 00423 const ArrayView<const attribute_handle_t>& characteristic_value_handles 00424 ) = 0; 00425 00426 /** 00427 * Send a write command to the server. 00428 * 00429 * @note This function issue an ATT Write Command. 00430 * 00431 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.1 00432 * 00433 * @param connection_handle The handle of the connection to send this request to. 00434 * @param attribute_handle Handle of the attribute to write. 00435 * @param value The value to write. 00436 * 00437 * @return BLE_ERROR_NONE or an appropriate error. 00438 */ 00439 virtual ble_error_t write_without_response( 00440 connection_handle_t connection_handle, 00441 attribute_handle_t characteristic_value_handle, 00442 const ArrayView<const uint8_t> & value 00443 ) = 0; 00444 00445 /** 00446 * Send a Signed Write without Response command to the server. 00447 * 00448 * @note This function issue an ATT Write Command with the signed flag and 00449 * the signature. 00450 * 00451 * @note signature is calculated by the stack. 00452 * 00453 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.2 00454 * 00455 * @param connection_handle The handle of the connection to send this request to. 00456 * @param attribute_handle Handle of the attribute to write. 00457 * @param value The value to write. 00458 * 00459 * @return BLE_ERROR_NONE or an appropriate error. 00460 */ 00461 virtual ble_error_t signed_write_without_response( 00462 connection_handle_t connection_handle, 00463 attribute_handle_t characteristic_value_handle, 00464 const ArrayView<const uint8_t> & value 00465 ) = 0; 00466 00467 /** 00468 * Send a write request to the server. 00469 * 00470 * The server should respond with a WriteResponse in case of success or an 00471 * ErrorResponse in case of error. 00472 * 00473 * @note This function issue an ATT Write Request. 00474 * 00475 * @note: This function is the base function for the Write Characteristic 00476 * Value and Write Characteristic Descriptors GATT procedures. 00477 * 00478 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.3 00479 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.12.3 00480 * 00481 * @param connection_handle The handle of the connection to send this request to. 00482 * @param attribute_handle Handle of the attribute to write. 00483 * @param value The value to write. 00484 * 00485 * @return BLE_ERROR_NONE or an appropriate error. 00486 */ 00487 virtual ble_error_t write_attribute( 00488 connection_handle_t connection_handle, 00489 attribute_handle_t attribute_handle, 00490 const ArrayView<const uint8_t> & value 00491 ) = 0; 00492 00493 /** 00494 * Send a prepare write request to the server. 00495 * 00496 * The write request will go into a queue until the client execute or cancel 00497 * the request with an execute write request which will write all the values 00498 * in the queue atomically. 00499 * 00500 * The server should respond with a PrepareWriteResponse containing the 00501 * content of the request in case of success and an ErrorResponse in case of 00502 * error. 00503 * 00504 * If an ErrorResponse is received it doesn't invalidate what is already in 00505 * the queue. 00506 * 00507 * @note This function issue an ATT Prepare Write Request. 00508 * 00509 * @note: This function is one of the base function for the Write Long 00510 * Characteristic Value and Reliable Writes GATT procedures. 00511 * 00512 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.4 00513 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.5 00514 * 00515 * @param connection_handle The handle of the connection to send this request to. 00516 * @param attribute_handle Handle of the attribute to write. 00517 * @param value The value to write. 00518 * @param offset offset where the value should be written. 00519 * 00520 * @return BLE_ERROR_NONE or an appropriate error. 00521 */ 00522 virtual ble_error_t queue_prepare_write( 00523 connection_handle_t connection_handle, 00524 attribute_handle_t characteristic_value_handle, 00525 const ArrayView<const uint8_t> & value, 00526 uint16_t offset 00527 ) = 0; 00528 00529 /** 00530 * Send a request to the server to execute the queue of prepared write 00531 * requests. 00532 * 00533 * The server should respond with an ExecuteWriteResponse in case of success 00534 * and an Error response in case of failure. 00535 * 00536 * @note This function issue an ATT Execute Write Request. 00537 * 00538 * @note: This function is one of the base function for the Write Long 00539 * Characteristic Value and Reliable Writes GATT procedures. 00540 * 00541 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.4 00542 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.9.5 00543 * 00544 * @param connection_handle The handle of the connection to send this request to. 00545 * @param execute If true, execute the write request queue otherwise cancel it. 00546 * 00547 * @return BLE_ERROR_NONE or an appropriate error. 00548 */ 00549 virtual ble_error_t execute_write_queue( 00550 connection_handle_t connection_handle, 00551 bool execute 00552 ) = 0; 00553 00554 /** 00555 * Register a callback which will handle messages from the server. 00556 * 00557 * @param cb The callback object which will handle messages from the server. 00558 * It accept two parameters in input: The handle of the connection where the 00559 * message was received and the message received. Real type of the message 00560 * can be obtained from its opcode. 00561 */ 00562 void when_server_message_received( 00563 mbed::Callback<void(connection_handle_t, const AttServerMessage&)> cb 00564 ) { 00565 _server_message_cb = cb; 00566 } 00567 00568 /** 00569 * Register a callback handling transaction timeout. 00570 * 00571 * @param cb The callback handling timeout of a transaction. It accepts as 00572 * a parameter the connection handle involved in the timeout. 00573 * 00574 * @note No more attribute protocol requests, commands, indication or 00575 * notification shall be sent over a connection implied in a transaction 00576 * timeout. To send a new ATT message, the conenction should be 00577 * reestablished. 00578 */ 00579 void when_transaction_timeout( 00580 mbed::Callback<void(connection_handle_t)> cb 00581 ) { 00582 _transaction_timeout_cb = cb; 00583 } 00584 00585 protected: 00586 GattClient() { } 00587 00588 virtual ~GattClient() { } 00589 00590 /** 00591 * Upon server message reception an implementation shall call this function. 00592 * 00593 * @param connection_handle The handle of the connection which has received 00594 * the server message. 00595 * @param server_message The message received from the server. 00596 */ 00597 void on_server_event( 00598 connection_handle_t connection_handle, 00599 const AttServerMessage& server_message 00600 ) { 00601 if (_server_message_cb) { 00602 _server_message_cb(connection_handle, server_message); 00603 } 00604 } 00605 00606 /** 00607 * Upon transaction timeout an implementation shall call this function. 00608 * 00609 * @param connection_handle The handle of the connection of the transaction 00610 * which has times out. 00611 * 00612 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part F Section 3.3.3 00613 * @note see BLUETOOTH SPECIFICATION Version 5.0 | Vol 3, Part G Section 4.4.14 00614 */ 00615 void on_transaction_timeout( 00616 connection_handle_t connection_handle 00617 ) { 00618 if (_transaction_timeout_cb) { 00619 _transaction_timeout_cb(connection_handle); 00620 } 00621 } 00622 00623 private: 00624 /** 00625 * Callback called when the client receive a message from the server. 00626 */ 00627 mbed::Callback<void(connection_handle_t, const AttServerMessage&)> _server_message_cb; 00628 00629 /** 00630 * Callback called when a transaction times out. 00631 */ 00632 mbed::Callback<void(connection_handle_t)> _transaction_timeout_cb; 00633 00634 // Disallow copy construction and copy assignment. 00635 GattClient(const GattClient&); 00636 GattClient& operator=(const GattClient&); 00637 }; 00638 00639 } // namespace pal 00640 } // namespace ble 00641 00642 #endif /* BLE_PAL_GATT_CLIENT_H_ */
Generated on Sun Jul 17 2022 08:25:29 by
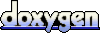