
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
NanostackInterface.h
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef NANOSTACK_INTERFACE_H_ 00018 #define NANOSTACK_INTERFACE_H_ 00019 00020 #include "mbed.h" 00021 #include "NetworkStack.h" 00022 #include "MeshInterface.h" 00023 // Include here for backward compatibility 00024 #include "LoWPANNDInterface.h" 00025 #include "ThreadInterface.h" 00026 #include "NanostackEthernetInterface.h" 00027 #include "MeshInterfaceNanostack.h" 00028 00029 struct ns_address; 00030 00031 class NanostackInterface : public NetworkStack { 00032 public: 00033 static NanostackInterface *get_stack(); 00034 00035 protected: 00036 00037 /** Get the local IP address 00038 * 00039 * @return Null-terminated representation of the local IP address 00040 * or null if not yet connected 00041 */ 00042 virtual const char *get_ip_address(); 00043 00044 /** Opens a socket 00045 * 00046 * Creates a network socket and stores it in the specified handle. 00047 * The handle must be passed to following calls on the socket. 00048 * 00049 * A stack may have a finite number of sockets, in this case 00050 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00051 * 00052 * @param handle Destination for the handle to a newly created socket 00053 * @param proto Protocol of socket to open, NSAPI_TCP or NSAPI_UDP 00054 * @return 0 on success, negative error code on failure 00055 */ 00056 virtual nsapi_error_t socket_open(void **handle, nsapi_protocol_t proto); 00057 00058 /** Close the socket 00059 * 00060 * Closes any open connection and deallocates any memory associated 00061 * with the socket. 00062 * 00063 * @param handle Socket handle 00064 * @return 0 on success, negative error code on failure 00065 */ 00066 virtual nsapi_error_t socket_close(void *handle); 00067 00068 /** Bind a specific address to a socket 00069 * 00070 * Binding a socket specifies the address and port on which to recieve 00071 * data. If the IP address is zeroed, only the port is bound. 00072 * 00073 * @param handle Socket handle 00074 * @param address Local address to bind 00075 * @return 0 on success, negative error code on failure. 00076 */ 00077 virtual nsapi_error_t socket_bind(void *handle, const SocketAddress &address); 00078 00079 /** Listen for connections on a TCP socket 00080 * 00081 * Marks the socket as a passive socket that can be used to accept 00082 * incoming connections. 00083 * 00084 * @param handle Socket handle 00085 * @param backlog Number of pending connections that can be queued 00086 * simultaneously 00087 * @return 0 on success, negative error code on failure 00088 */ 00089 virtual nsapi_error_t socket_listen(void *handle, int backlog); 00090 00091 /** Connects TCP socket to a remote host 00092 * 00093 * Initiates a connection to a remote server specified by the 00094 * indicated address. 00095 * 00096 * @param handle Socket handle 00097 * @param address The SocketAddress of the remote host 00098 * @return 0 on success, negative error code on failure 00099 */ 00100 virtual nsapi_error_t socket_connect(void *handle, const SocketAddress &address); 00101 00102 /** Accepts a connection on a TCP socket 00103 * 00104 * The server socket must be bound and set to listen for connections. 00105 * On a new connection, creates a network socket and stores it in the 00106 * specified handle. The handle must be passed to following calls on 00107 * the socket. 00108 * 00109 * A stack may have a finite number of sockets, in this case 00110 * NSAPI_ERROR_NO_SOCKET is returned if no socket is available. 00111 * 00112 * This call is non-blocking. If accept would block, 00113 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00114 * 00115 * @param server Socket handle to server to accept from 00116 * @param handle Destination for a handle to the newly created socket 00117 * @param address Destination for the remote address or NULL 00118 * @return 0 on success, negative error code on failure 00119 */ 00120 virtual nsapi_error_t socket_accept(void *handle, void **server, SocketAddress *address); 00121 00122 /** Send data over a TCP socket 00123 * 00124 * The socket must be connected to a remote host. Returns the number of 00125 * bytes sent from the buffer. 00126 * 00127 * This call is non-blocking. If send would block, 00128 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00129 * 00130 * @param handle Socket handle 00131 * @param data Buffer of data to send to the host 00132 * @param size Size of the buffer in bytes 00133 * @return Number of sent bytes on success, negative error 00134 * code on failure 00135 */ 00136 virtual nsapi_size_or_error_t socket_send(void *handle, const void *data, nsapi_size_t size); 00137 00138 /** Receive data over a TCP socket 00139 * 00140 * The socket must be connected to a remote host. Returns the number of 00141 * bytes received into the buffer. 00142 * 00143 * This call is non-blocking. If recv would block, 00144 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00145 * 00146 * @param handle Socket handle 00147 * @param data Destination buffer for data received from the host 00148 * @param size Size of the buffer in bytes 00149 * @return Number of received bytes on success, negative error 00150 * code on failure 00151 */ 00152 virtual nsapi_size_or_error_t socket_recv(void *handle, void *data, nsapi_size_t size); 00153 00154 /** Send a packet over a UDP socket 00155 * 00156 * Sends data to the specified address. Returns the number of bytes 00157 * sent from the buffer. 00158 * 00159 * This call is non-blocking. If sendto would block, 00160 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00161 * 00162 * @param handle Socket handle 00163 * @param address The SocketAddress of the remote host 00164 * @param data Buffer of data to send to the host 00165 * @param size Size of the buffer in bytes 00166 * @return Number of sent bytes on success, negative error 00167 * code on failure 00168 */ 00169 virtual nsapi_size_or_error_t socket_sendto(void *handle, const SocketAddress &address, const void *data, nsapi_size_t size); 00170 00171 /** Receive a packet over a UDP socket 00172 * 00173 * Receives data and stores the source address in address if address 00174 * is not NULL. Returns the number of bytes received into the buffer. 00175 * 00176 * This call is non-blocking. If recvfrom would block, 00177 * NSAPI_ERROR_WOULD_BLOCK is returned immediately. 00178 * 00179 * @param handle Socket handle 00180 * @param address Destination for the source address or NULL 00181 * @param data Destination buffer for data received from the host 00182 * @param size Size of the buffer in bytes 00183 * @return Number of received bytes on success, negative error 00184 * code on failure 00185 */ 00186 virtual nsapi_size_or_error_t socket_recvfrom(void *handle, SocketAddress *address, void *buffer, nsapi_size_t size); 00187 00188 /** Register a callback on state change of the socket 00189 * 00190 * The specified callback will be called on state changes such as when 00191 * the socket can recv/send/accept successfully and on when an error 00192 * occurs. The callback may also be called spuriously without reason. 00193 * 00194 * The callback may be called in an interrupt context and should not 00195 * perform expensive operations such as recv/send calls. 00196 * 00197 * @param handle Socket handle 00198 * @param callback Function to call on state change 00199 * @param data Argument to pass to callback 00200 */ 00201 virtual void socket_attach(void *handle, void (*callback)(void *), void *data); 00202 00203 /* Set stack-specific socket options 00204 * 00205 * The setsockopt allow an application to pass stack-specific hints 00206 * to the underlying stack. For unsupported options, 00207 * NSAPI_ERROR_UNSUPPORTED is returned and the socket is unmodified. 00208 * 00209 * @param handle Socket handle 00210 * @param level Stack-specific protocol level 00211 * @param optname Stack-specific option identifier 00212 * @param optval Option value 00213 * @param optlen Length of the option value 00214 * @return 0 on success, negative error code on failure 00215 */ 00216 virtual nsapi_error_t setsockopt(void *handle, int level, int optname, const void *optval, unsigned optlen); 00217 00218 /* Get stack-specific socket options 00219 * 00220 * The getstackopt allow an application to retrieve stack-specific hints 00221 * from the underlying stack. For unsupported options, 00222 * NSAPI_ERROR_UNSUPPORTED is returned and optval is unmodified. 00223 * 00224 * @param handle Socket handle 00225 * @param level Stack-specific protocol level 00226 * @param optname Stack-specific option identifier 00227 * @param optval Destination for option value 00228 * @param optlen Length of the option value 00229 * @return 0 on success, negative error code on failure 00230 */ 00231 virtual nsapi_error_t getsockopt(void *handle, int level, int optname, void *optval, unsigned *optlen); 00232 00233 private: 00234 nsapi_size_or_error_t do_sendto(void *handle, const struct ns_address *address, const void *data, nsapi_size_t size); 00235 char text_ip_address[40]; 00236 static NanostackInterface * _ns_interface; 00237 }; 00238 00239 nsapi_error_t map_mesh_error(mesh_error_t err); 00240 00241 #endif /* NANOSTACK_INTERFACE_H_ */
Generated on Sun Jul 17 2022 08:25:28 by
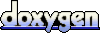