
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
MBRBlockDevice.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 ARM Limited 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00020 * SOFTWARE. 00021 */ 00022 #ifndef MBED_MBR_BLOCK_DEVICE_H 00023 #define MBED_MBR_BLOCK_DEVICE_H 00024 00025 #include "BlockDevice.h" 00026 #include "mbed.h" 00027 00028 00029 /** Additional error codes used for MBR records 00030 */ 00031 enum { 00032 BD_ERROR_INVALID_MBR = -3101, 00033 BD_ERROR_INVALID_PARTITION = -3102, 00034 }; 00035 00036 00037 /** Block device for managing a Master Boot Record 00038 * https://en.wikipedia.org/wiki/Master_boot_record 00039 * 00040 * Here is an example of partitioning a heap backed block device 00041 * @code 00042 * #include "mbed.h" 00043 * #include "HeapBlockDevice.h" 00044 * #include "MBRBlockDevice.h" 00045 * 00046 * // Create a block device with 64 blocks of size 512 00047 * HeapBlockDevice mem(64*512, 512); 00048 * 00049 * // Partition into two partitions with ~half the blocks 00050 * MBRBlockDevice::partition(&mem, 1, 0x83, 0*512, 32*512); 00051 * MBRBlockDevice::partition(&mem, 2, 0x83, 32*512); 00052 * 00053 * // Create a block device that maps to the first 32 blocks (excluding MBR block) 00054 * MBRBlockDevice part1(&mem, 1); 00055 * 00056 * // Create a block device that maps to the last 32 blocks 00057 * MBRBlockDevice part2(&mem, 2); 00058 * @endcode 00059 * 00060 * Here is a more realistic example where the MBRBlockDevice is used 00061 * to partition a region of space on an SD card. When plugged into a computer, 00062 * the partitions will be recognized appropriately. 00063 * @code 00064 * #include "mbed.h" 00065 * #include "SDBlockDevice.h" 00066 * #include "MBRBlockDevice.h" 00067 * #include "FATFileSystem.h" 00068 * 00069 * // Create an SD card 00070 * SDBlockDevice sd(s0, s1, s2, s3); 00071 * 00072 * // Create a partition with 1 GB of space 00073 * MBRBlockDevice::partition(&sd, 1, 0x83, 0, 1024*1024); 00074 * 00075 * // Create the block device that represents the partition 00076 * MBRBlockDevice part1(&sd, 1); 00077 * 00078 * // Format the partition with a FAT filesystem 00079 * FATFileSystem::format(&part1); 00080 * 00081 * // Create the FAT filesystem instance, files can now be written to 00082 * // the FAT filesystem in partition 1 00083 * FATFileSystem fat("fat", &part1); 00084 * @endcode 00085 */ 00086 class MBRBlockDevice : public BlockDevice 00087 { 00088 public: 00089 /** Format the MBR to contain the following partition 00090 * 00091 * @param bd Block device to partition 00092 * @param part Partition to use, 1-4 00093 * @param type 8-bit partition type to identitfy partition's contents 00094 * @param start Start block address to map to block 0 of partition, 00095 * negative addresses are calculated from the end of the 00096 * underlying block devices. Block 0 is implicitly ignored 00097 * from the range to store the MBR. 00098 * @return 0 on success or a negative error code on failure 00099 * @note This is the same as partition(bd, part, type, start, bd->size()) 00100 */ 00101 static int partition(BlockDevice *bd, int part, uint8_t type, bd_addr_t start); 00102 00103 /** Format the MBR to contain the following partition 00104 * 00105 * @param bd Block device to partition 00106 * @param part Partition to use, 1-4 00107 * @param type 8-bit partition type to identitfy partition's contents 00108 * @param start Start block address to map to block 0 of partition, 00109 * negative addresses are calculated from the end of the 00110 * underlying block devices. Block 0 is implicitly ignored 00111 * from the range to store the MBR. 00112 * @param stop End block address to mark the end of the partition, 00113 * this block is not mapped, negative addresses are calculated 00114 * from the end of the underlying block device. 00115 * @return 0 on success or a negative error code on failure 00116 */ 00117 static int partition(BlockDevice *bd, int part, uint8_t type, bd_addr_t start, bd_addr_t stop); 00118 00119 /** Lifetime of the block device 00120 * 00121 * @param bd Block device to back the MBRBlockDevice 00122 * @param part Partition to use, 1-4 00123 */ 00124 MBRBlockDevice(BlockDevice *bd, int part); 00125 00126 /** Lifetime of the block device 00127 */ 00128 virtual ~MBRBlockDevice() {}; 00129 00130 /** Initialize a block device 00131 * 00132 * @return 0 on success or a negative error code on failure 00133 */ 00134 virtual int init(); 00135 00136 /** Deinitialize a block device 00137 * 00138 * @return 0 on success or a negative error code on failure 00139 */ 00140 virtual int deinit(); 00141 00142 /** Read blocks from a block device 00143 * 00144 * @param buffer Buffer to read blocks into 00145 * @param addr Address of block to begin reading from 00146 * @param size Size to read in bytes, must be a multiple of read block size 00147 * @return 0 on success, negative error code on failure 00148 */ 00149 virtual int read(void *buffer, bd_addr_t addr, bd_size_t size); 00150 00151 /** Program blocks to a block device 00152 * 00153 * The blocks must have been erased prior to being programmed 00154 * 00155 * @param buffer Buffer of data to write to blocks 00156 * @param addr Address of block to begin writing to 00157 * @param size Size to write in bytes, must be a multiple of program block size 00158 * @return 0 on success, negative error code on failure 00159 */ 00160 virtual int program(const void *buffer, bd_addr_t addr, bd_size_t size); 00161 00162 /** Erase blocks on a block device 00163 * 00164 * The state of an erased block is undefined until it has been programmed 00165 * 00166 * @param addr Address of block to begin erasing 00167 * @param size Size to erase in bytes, must be a multiple of erase block size 00168 * @return 0 on success, negative error code on failure 00169 */ 00170 virtual int erase(bd_addr_t addr, bd_size_t size); 00171 00172 /** Get the size of a readable block 00173 * 00174 * @return Size of a readable block in bytes 00175 */ 00176 virtual bd_size_t get_read_size() const; 00177 00178 /** Get the size of a programable block 00179 * 00180 * @return Size of a programable block in bytes 00181 * @note Must be a multiple of the read size 00182 */ 00183 virtual bd_size_t get_program_size() const; 00184 00185 /** Get the size of a eraseable block 00186 * 00187 * @return Size of a eraseable block in bytes 00188 * @note Must be a multiple of the program size 00189 */ 00190 virtual bd_size_t get_erase_size() const; 00191 00192 /** Get the total size of the underlying device 00193 * 00194 * @return Size of the underlying device in bytes 00195 */ 00196 virtual bd_size_t size() const; 00197 00198 /** Get the offset of the partition on the underlying block device 00199 * @return Offset of the partition on the underlying device 00200 */ 00201 virtual bd_addr_t get_partition_start() const; 00202 00203 /** Get size of partition on underlying block device 00204 * @return Size of the partition on the underlying device 00205 */ 00206 virtual bd_addr_t get_partition_stop() const; 00207 00208 /** Get 8-bit type of the partition 00209 * @return 8-bit type of partition assigned during format 00210 */ 00211 virtual uint8_t get_partition_type() const; 00212 00213 /** Get the partition number 00214 * @return The partition number, 1-4 00215 */ 00216 virtual int get_partition_number() const; 00217 00218 protected: 00219 BlockDevice *_bd; 00220 bd_size_t _offset; 00221 bd_size_t _size; 00222 uint8_t _type; 00223 uint8_t _part; 00224 }; 00225 00226 00227 #endif
Generated on Sun Jul 17 2022 08:25:28 by
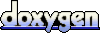