
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
GattCharacteristic.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __GATT_CHARACTERISTIC_H__ 00018 #define __GATT_CHARACTERISTIC_H__ 00019 00020 #include "Gap.h" 00021 #include "SecurityManager.h" 00022 #include "GattAttribute.h" 00023 #include "GattCallbackParamTypes.h" 00024 #include "FunctionPointerWithContext.h " 00025 00026 /** 00027 * @addtogroup ble 00028 * @{ 00029 * @addtogroup gatt 00030 * @{ 00031 * @addtogroup server 00032 * @{ 00033 */ 00034 00035 /** 00036 * Representation of a GattServer characteristic. 00037 * 00038 * A characteristic is a typed value enclosed in a GATT service (GattService). 00039 * 00040 * @par Type 00041 * 00042 * The type of the value defines the purpose of the characteristic, and a 00043 * UUID represents it. Standard characteristic types may be consulted at 00044 * https://www.bluetooth.com/specifications/gatt/characteristics 00045 * 00046 * @par Supported operations 00047 * A set of properties define what client operations the characteristic 00048 * supports. See GattServer::Properties_t 00049 * 00050 * @par Descriptors 00051 * 00052 * Additional information, such as the unit of the characteristic value, a 00053 * description string or a client control point, can be added to the 00054 * characteristic. 00055 * 00056 * See BLUETOOTH SPECIFICATION Version 4.2 [Vol 3, Part G] - 3.3.1.1 00057 * 00058 * One of the most important types of descriptor is the Client Characteristic 00059 * Configuration Descriptor (CCCD) that must be present if the characteristic 00060 * properties allow a client to subscribe to updates of the characteristic 00061 * value. 00062 * 00063 * @par Characteristic breakdown 00064 * 00065 * A characteristic is composed of several GATT attributes (GattAttribute): 00066 * - Characteristic declaration: It contains the properties of the 00067 * characteristic, its type and the handle of its value. 00068 * - Characteristic value: The value of the characteristic. 00069 * - Descriptors: A single GATT attribute stores each descriptor. 00070 * 00071 * When the GattService containing the characteristic is registered in the 00072 * GattServer, a unique attribute handle is assigned to the various attributes 00073 * of the characteristic. Clients use this handle to interact with the 00074 * characteristic. This handle is used locally in GattServer APIs. 00075 * 00076 * 00077 * 00078 * 00079 * 00080 * 00081 * 00082 * 00083 * Representation of a GattServer characteristic. 00084 * 00085 * A characteristic is a typed value used in a service. It contains a set of 00086 * properties that define client operations supported by the characteristic. 00087 * A characteristic may also include descriptors; a descriptor exposes 00088 * metainformation associated to a characteristic, such as the unit of its value, 00089 * its human readable name or a control point attribute that allows the client to 00090 * subscribe to the characteristic notifications. 00091 * 00092 * The GattCharacteristic class allows application code to construct 00093 * and monitor characteristics presents in a GattServer. 00094 */ 00095 class GattCharacteristic { 00096 public: 00097 00098 /* 00099 * Enumeration of characteristic UUID defined by the Bluetooth body. 00100 */ 00101 enum { 00102 /** 00103 * Not used in actual BLE service. 00104 */ 00105 UUID_BATTERY_LEVEL_STATE_CHAR = 0x2A1B, 00106 00107 /** 00108 * Not used in actual BLE service. 00109 */ 00110 UUID_BATTERY_POWER_STATE_CHAR = 0x2A1A, 00111 00112 /** 00113 * Not used in actual BLE service. 00114 */ 00115 UUID_REMOVABLE_CHAR = 0x2A3A, 00116 00117 /** 00118 * Not used in actual BLE service. 00119 */ 00120 UUID_SERVICE_REQUIRED_CHAR = 0x2A3B, 00121 00122 /** 00123 * Not used as a characteristic UUID. 00124 */ 00125 UUID_ALERT_CATEGORY_ID_CHAR = 0x2A43, 00126 00127 /** 00128 * Not used as a characteristic UUID. 00129 */ 00130 UUID_ALERT_CATEGORY_ID_BIT_MASK_CHAR = 0x2A42, 00131 00132 /** 00133 * Control point of the Immediate Alert service that allows the client to 00134 * command the server to alert to a given level. 00135 */ 00136 UUID_ALERT_LEVEL_CHAR = 0x2A06, 00137 00138 /** 00139 * Control point of the Alert Notification service that allows the client 00140 * finely tune the notification configuration. 00141 */ 00142 UUID_ALERT_NOTIFICATION_CONTROL_POINT_CHAR = 0x2A44, 00143 00144 /** 00145 * Part of the Alert Notification service, which exposes the count of 00146 * unread alert events existing in the server. 00147 */ 00148 UUID_ALERT_STATUS_CHAR = 0x2A3F, 00149 00150 /** 00151 * Characteristic of the Battery service, which exposes the current 00152 * battery level as a percentage. 00153 */ 00154 UUID_BATTERY_LEVEL_CHAR = 0x2A19, 00155 00156 /** 00157 * Describe the features supported by the blood pressure sensor exposed 00158 * by the Blood Pressure service. 00159 */ 00160 UUID_BLOOD_PRESSURE_FEATURE_CHAR = 0x2A49, 00161 00162 /** 00163 * Characteristic of the Blood Pressure service that exposes the 00164 * measurement of the blood sensor. 00165 */ 00166 UUID_BLOOD_PRESSURE_MEASUREMENT_CHAR = 0x2A35, 00167 00168 /** 00169 * Characteristic of the Heart Rate service that indicate the intended 00170 * location of the heart rate monitor. 00171 */ 00172 UUID_BODY_SENSOR_LOCATION_CHAR = 0x2A38, 00173 00174 /** 00175 * Part of the Human Interface Device service. 00176 */ 00177 UUID_BOOT_KEYBOARD_INPUT_REPORT_CHAR = 0x2A22, 00178 00179 /** 00180 * Part of the Human Interface Device service. 00181 */ 00182 UUID_BOOT_KEYBOARD_OUTPUT_REPORT_CHAR = 0x2A32, 00183 00184 /** 00185 * Part of the Human Interface Device service. 00186 */ 00187 UUID_BOOT_MOUSE_INPUT_REPORT_CHAR = 0x2A33, 00188 00189 /** 00190 * Characteristic of the Current Time service that contains the current 00191 * time. 00192 */ 00193 UUID_CURRENT_TIME_CHAR = 0x2A2B, 00194 00195 /** 00196 * Not used in a service as a characteristic. 00197 */ 00198 UUID_DATE_TIME_CHAR = 0x2A08, 00199 00200 /** 00201 * Not used in a service as a characteristic. 00202 */ 00203 UUID_DAY_DATE_TIME_CHAR = 0x2A0A, 00204 00205 /** 00206 * Not used in a service as a characteristic. 00207 */ 00208 UUID_DAY_OF_WEEK_CHAR = 0x2A09, 00209 00210 /** 00211 * Not used in a service as a characteristic. 00212 */ 00213 UUID_DST_OFFSET_CHAR = 0x2A0D, 00214 00215 /** 00216 * Not used in a service as a characteristic. 00217 */ 00218 UUID_EXACT_TIME_256_CHAR = 0x2A0C, 00219 00220 /** 00221 * Characteristic of the Device Information Service that contains a 00222 * UTF8 string representing the firmware revision for the firmware within 00223 * the device. 00224 */ 00225 UUID_FIRMWARE_REVISION_STRING_CHAR = 0x2A26, 00226 00227 /** 00228 * Characteristic of the Glucose service that exposes features supported 00229 * by the server. 00230 */ 00231 UUID_GLUCOSE_FEATURE_CHAR = 0x2A51, 00232 00233 /** 00234 * Characteristic of the Glucose service that exposes glucose 00235 * measurements. 00236 */ 00237 UUID_GLUCOSE_MEASUREMENT_CHAR = 0x2A18, 00238 00239 /** 00240 * Characteristic of the Glucose service that sends additional 00241 * information related to the glucose measurements. 00242 */ 00243 UUID_GLUCOSE_MEASUREMENT_CONTEXT_CHAR = 0x2A34, 00244 00245 /** 00246 * Characteristic of the Device Information Service that contains a 00247 * UTF8 string representing the hardware revision of the device. 00248 */ 00249 UUID_HARDWARE_REVISION_STRING_CHAR = 0x2A27, 00250 00251 /** 00252 * Characteristic of the Heart Rate service used by the client to control 00253 * the service behavior. 00254 */ 00255 UUID_HEART_RATE_CONTROL_POINT_CHAR = 0x2A39, 00256 00257 /** 00258 * Characteristic of the Heart Rate that sends heart rate measurements to 00259 * registered clients. 00260 */ 00261 UUID_HEART_RATE_MEASUREMENT_CHAR = 0x2A37, 00262 00263 /** 00264 * Part of the Human Interface Device service. 00265 */ 00266 UUID_HID_CONTROL_POINT_CHAR = 0x2A4C, 00267 00268 /** 00269 * Part of the Human Interface Device service. 00270 */ 00271 UUID_HID_INFORMATION_CHAR = 0x2A4A, 00272 00273 /** 00274 * Characteristic of the Environmental Sensing service, which exposes 00275 * humidity measurements. 00276 */ 00277 UUID_HUMIDITY_CHAR = 0x2A6F, 00278 00279 /** 00280 * Characteristic of the Device Information Service, which exposes 00281 * various regulatory or certification compliance items to which the 00282 * device claims adherence. 00283 */ 00284 UUID_IEEE_REGULATORY_CERTIFICATION_DATA_LIST_CHAR = 0x2A2A, 00285 00286 /** 00287 * Characteristic of the Blood Pressure service, which exposes intermediate 00288 * cuff pressure measurements. 00289 */ 00290 UUID_INTERMEDIATE_CUFF_PRESSURE_CHAR = 0x2A36, 00291 00292 /** 00293 * Characteristic of the Health Thermometer service that sends intermediate 00294 * temperature values while the measurement is in progress. 00295 */ 00296 UUID_INTERMEDIATE_TEMPERATURE_CHAR = 0x2A1E, 00297 00298 /** 00299 * Characteristic of the current Time service that exposes information 00300 * about the local time. 00301 */ 00302 UUID_LOCAL_TIME_INFORMATION_CHAR = 0x2A0F, 00303 00304 /** 00305 * Characteristic of the Device Information Service that contains a 00306 * UTF8 string representing the manufacturer name of the device. 00307 */ 00308 UUID_MANUFACTURER_NAME_STRING_CHAR = 0x2A29, 00309 00310 /** 00311 * Characteristic of the Health Thermometer service that exposes the 00312 * interval time between two measurements. 00313 */ 00314 UUID_MEASUREMENT_INTERVAL_CHAR = 0x2A21, 00315 00316 /** 00317 * Characteristic of the Device Information Service that contains a 00318 * UTF8 string representing the model number of the device assigned by 00319 * the vendor. 00320 */ 00321 UUID_MODEL_NUMBER_STRING_CHAR = 0x2A24, 00322 00323 /** 00324 * Characteristic of the Alert Notification Service that shows how many 00325 * numbers of unread alerts exist in the specific category in the device. 00326 */ 00327 UUID_UNREAD_ALERT_CHAR = 0x2A45, 00328 00329 /** 00330 * Characteristic of the Alert Notification Service that defines the 00331 * category of the alert and how many new alerts of that category have 00332 * occurred in the server. 00333 */ 00334 UUID_NEW_ALERT_CHAR = 0x2A46, 00335 00336 /** 00337 * Characteristic of the Device Information Service; it is a set of 00338 * values used to create a device ID that is unique for this device. 00339 */ 00340 UUID_PNP_ID_CHAR = 0x2A50, 00341 00342 /** 00343 * Characteristic of the Environmental Sensing Service that exposes the 00344 * pressure measured. 00345 */ 00346 UUID_PRESSURE_CHAR = 0x2A6D, 00347 00348 /** 00349 * Part of the Human Interface Device service. 00350 */ 00351 UUID_PROTOCOL_MODE_CHAR = 0x2A4E, 00352 00353 /** 00354 * Pulse Oxymeter, Glucose and Continuous Glucose Monitoring services 00355 * use this control point to provide basic management of the patient 00356 * record database. 00357 */ 00358 UUID_RECORD_ACCESS_CONTROL_POINT_CHAR = 0x2A52, 00359 00360 /** 00361 * Characteristic of the Current Time service that exposes information 00362 * related to the current time served (accuracy, source, hours since 00363 * update and so on). 00364 */ 00365 UUID_REFERENCE_TIME_INFORMATION_CHAR = 0x2A14, 00366 00367 /** 00368 * Part of the Human Interface Device service. 00369 */ 00370 UUID_REPORT_CHAR = 0x2A4D, 00371 00372 /** 00373 * Part of the Human Interface Device service. 00374 */ 00375 UUID_REPORT_MAP_CHAR = 0x2A4B, 00376 00377 /** 00378 * Characteristic of the Phone Alert Status service that allows a client 00379 * to configure operating mode. 00380 */ 00381 UUID_RINGER_CONTROL_POINT_CHAR = 0x2A40, 00382 00383 /** 00384 * Characteristic of the Phone Alert Status service that returns the 00385 * ringer setting when read. 00386 */ 00387 UUID_RINGER_SETTING_CHAR = 0x2A41, 00388 00389 /** 00390 * Characteristic of the Scan Parameter service that stores the client's 00391 * scan parameters (scan interval and scan window). 00392 */ 00393 UUID_SCAN_INTERVAL_WINDOW_CHAR = 0x2A4F, 00394 00395 /** 00396 * Characteristic of the Scan Parameter service that sends a notification 00397 * to a client when the server requires its latest scan parameters. 00398 */ 00399 UUID_SCAN_REFRESH_CHAR = 0x2A31, 00400 00401 /** 00402 * Characteristic of the Device Information Service that contains a 00403 * UTF8 string representing the serial number of the device. 00404 */ 00405 UUID_SERIAL_NUMBER_STRING_CHAR = 0x2A25, 00406 00407 /** 00408 * Characteristic of the Device Information Service that contains an 00409 * UTF8 string representing the software revision of the device. 00410 */ 00411 UUID_SOFTWARE_REVISION_STRING_CHAR = 0x2A28, 00412 00413 /** 00414 * Characteristic of the Alert Notification Service that notifies the 00415 * count of new alerts for a given category to a subscribed client. 00416 */ 00417 UUID_SUPPORTED_NEW_ALERT_CATEGORY_CHAR = 0x2A47, 00418 00419 /** 00420 * Characteristic of the Alert Notification service, which exposes 00421 * categories of unread alert supported by the server. 00422 */ 00423 UUID_SUPPORTED_UNREAD_ALERT_CATEGORY_CHAR = 0x2A48, 00424 00425 /** 00426 * Characteristic of the Device Information Service that exposes a 00427 * structure containing an Organizationally Unique Identifier (OUI) 00428 * followed by a manufacturer-defined identifier. The value of the 00429 * structure is unique for each individual instance of the product. 00430 */ 00431 UUID_SYSTEM_ID_CHAR = 0x2A23, 00432 00433 /** 00434 * Characteristic of the Environmental Sensing service that exposes the 00435 * temperature measurement with a resolution of 0.01 degree Celsius. 00436 */ 00437 UUID_TEMPERATURE_CHAR = 0x2A6E, 00438 00439 /** 00440 * Characteristic of the Health Thermometer service that sends temperature 00441 * measurement to clients. 00442 */ 00443 UUID_TEMPERATURE_MEASUREMENT_CHAR = 0x2A1C, 00444 00445 /** 00446 * Characteristic of the Health Thermometer service that describes 00447 * where the measurement takes place. 00448 */ 00449 UUID_TEMPERATURE_TYPE_CHAR = 0x2A1D, 00450 00451 /** 00452 * Not used in a service as a characteristic. 00453 */ 00454 UUID_TIME_ACCURACY_CHAR = 0x2A12, 00455 00456 /** 00457 * Not used in a service as a characteristic. 00458 */ 00459 UUID_TIME_SOURCE_CHAR = 0x2A13, 00460 00461 /** 00462 * Characteristic of the Reference Time service that allows clients to 00463 * control time update. 00464 */ 00465 UUID_TIME_UPDATE_CONTROL_POINT_CHAR = 0x2A16, 00466 00467 /** 00468 * Characteristic of the Reference Time service that informs clients of 00469 * the status of the time update operation. 00470 */ 00471 UUID_TIME_UPDATE_STATE_CHAR = 0x2A17, 00472 00473 /** 00474 * Characteristic of the Next DST Change service that returns to clients 00475 * the time with DST. 00476 */ 00477 UUID_TIME_WITH_DST_CHAR = 0x2A11, 00478 00479 /** 00480 * Not used in a service as a characteristic. 00481 */ 00482 UUID_TIME_ZONE_CHAR = 0x2A0E, 00483 00484 /** 00485 * Characteristic of the TX Power service that exposes the current 00486 * transmission power in dBm. 00487 */ 00488 UUID_TX_POWER_LEVEL_CHAR = 0x2A07, 00489 00490 /** 00491 * Characteristic of the Cycling Speed and Cadence (CSC) service that 00492 * exposes features supported by the server. 00493 */ 00494 UUID_CSC_FEATURE_CHAR = 0x2A5C, 00495 00496 /** 00497 * Characteristic of the Cycling Speed and Cadence (CSC) service that 00498 * exposes measurements made by the server. 00499 */ 00500 UUID_CSC_MEASUREMENT_CHAR = 0x2A5B, 00501 00502 /** 00503 * Characteristic of the Running Speed and Cadence (RSC) service that 00504 * exposes features supported by the server. 00505 */ 00506 UUID_RSC_FEATURE_CHAR = 0x2A54, 00507 00508 /** 00509 * Characteristic of the Running Speed and Cadence (RSC) service that 00510 * exposes measurements made by the server. 00511 */ 00512 UUID_RSC_MEASUREMENT_CHAR = 0x2A53 00513 }; 00514 00515 /** 00516 * Unit type of a characteristic value. 00517 * 00518 * These unit types are used to describe what the raw numeric data in a 00519 * characteristic actually represents. A server can expose that information 00520 * to its clients by adding a Characteristic Presentation Format descriptor 00521 * to relevant characteristics. 00522 * 00523 * @note See https://developer.bluetooth.org/gatt/units/Pages/default.aspx 00524 */ 00525 enum { 00526 00527 /** 00528 * No specified unit type. 00529 */ 00530 BLE_GATT_UNIT_NONE = 0x2700, 00531 00532 /** 00533 * Length, meter. 00534 */ 00535 BLE_GATT_UNIT_LENGTH_METRE = 0x2701, 00536 00537 /** 00538 * Mass, kilogram. 00539 */ 00540 BLE_GATT_UNIT_MASS_KILOGRAM = 0x2702, 00541 00542 /** 00543 * Time, second. 00544 */ 00545 BLE_GATT_UNIT_TIME_SECOND = 0x2703, 00546 00547 /** 00548 * Electric current, ampere. 00549 */ 00550 BLE_GATT_UNIT_ELECTRIC_CURRENT_AMPERE = 0x2704, 00551 00552 /** 00553 * Thermodynamic temperature, kelvin. 00554 */ 00555 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_KELVIN = 0x2705, 00556 00557 /** Amount of substance, mole. 00558 * 00559 */ 00560 BLE_GATT_UNIT_AMOUNT_OF_SUBSTANCE_MOLE = 0x2706, 00561 00562 /** 00563 * Luminous intensity, candela. 00564 */ 00565 BLE_GATT_UNIT_LUMINOUS_INTENSITY_CANDELA = 0x2707, 00566 00567 /** 00568 * Area, square meters. 00569 */ 00570 BLE_GATT_UNIT_AREA_SQUARE_METRES = 0x2710, 00571 00572 /** 00573 * Volume, cubic meters. 00574 */ 00575 BLE_GATT_UNIT_VOLUME_CUBIC_METRES = 0x2711, 00576 00577 /** 00578 * Velocity, meters per second. 00579 */ 00580 BLE_GATT_UNIT_VELOCITY_METRES_PER_SECOND = 0x2712, 00581 00582 /** 00583 * Acceleration, meters per second squared. 00584 */ 00585 BLE_GATT_UNIT_ACCELERATION_METRES_PER_SECOND_SQUARED = 0x2713, 00586 00587 /** 00588 * Wave number reciprocal, meter. 00589 */ 00590 BLE_GATT_UNIT_WAVENUMBER_RECIPROCAL_METRE = 0x2714, 00591 00592 /** 00593 * Density, kilogram per cubic meter. 00594 */ 00595 BLE_GATT_UNIT_DENSITY_KILOGRAM_PER_CUBIC_METRE = 0x2715, 00596 00597 /** 00598 * Surface density (kilogram per square meter). 00599 */ 00600 BLE_GATT_UNIT_SURFACE_DENSITY_KILOGRAM_PER_SQUARE_METRE = 0x2716, 00601 00602 /** 00603 * Specific volume (cubic meter per kilogram). 00604 */ 00605 BLE_GATT_UNIT_SPECIFIC_VOLUME_CUBIC_METRE_PER_KILOGRAM = 0x2717, 00606 00607 /** 00608 * Current density (ampere per square meter). 00609 */ 00610 BLE_GATT_UNIT_CURRENT_DENSITY_AMPERE_PER_SQUARE_METRE = 0x2718, 00611 00612 /** 00613 * Magnetic field strength, ampere per meter. 00614 */ 00615 BLE_GATT_UNIT_MAGNETIC_FIELD_STRENGTH_AMPERE_PER_METRE = 0x2719, 00616 00617 /** 00618 * Amount concentration (mole per cubic meter). 00619 */ 00620 BLE_GATT_UNIT_AMOUNT_CONCENTRATION_MOLE_PER_CUBIC_METRE = 0x271A, 00621 00622 /** 00623 * Mass concentration (kilogram per cubic meter). 00624 */ 00625 BLE_GATT_UNIT_MASS_CONCENTRATION_KILOGRAM_PER_CUBIC_METRE = 0x271B, 00626 00627 /** 00628 * Luminance (candela per square meter). 00629 */ 00630 BLE_GATT_UNIT_LUMINANCE_CANDELA_PER_SQUARE_METRE = 0x271C, 00631 00632 /** 00633 * Refractive index. 00634 */ 00635 BLE_GATT_UNIT_REFRACTIVE_INDEX = 0x271D, 00636 00637 /** 00638 * Relative permeability. 00639 */ 00640 BLE_GATT_UNIT_RELATIVE_PERMEABILITY = 0x271E, 00641 00642 /** 00643 * Plane angle (radian). 00644 */ 00645 BLE_GATT_UNIT_PLANE_ANGLE_RADIAN = 0x2720, 00646 00647 /** 00648 * Solid angle (steradian). 00649 */ 00650 BLE_GATT_UNIT_SOLID_ANGLE_STERADIAN = 0x2721, 00651 00652 /** 00653 * Frequency, hertz. 00654 */ 00655 BLE_GATT_UNIT_FREQUENCY_HERTZ = 0x2722, 00656 00657 /** 00658 * Force, newton. 00659 */ 00660 BLE_GATT_UNIT_FORCE_NEWTON = 0x2723, 00661 00662 /** 00663 * Pressure, pascal. 00664 */ 00665 BLE_GATT_UNIT_PRESSURE_PASCAL = 0x2724, 00666 00667 /** 00668 * Energy, joule. 00669 */ 00670 BLE_GATT_UNIT_ENERGY_JOULE = 0x2725, 00671 00672 /** 00673 * Power, watt. 00674 */ 00675 BLE_GATT_UNIT_POWER_WATT = 0x2726, 00676 00677 /** 00678 * Electrical charge, coulomb. 00679 */ 00680 BLE_GATT_UNIT_ELECTRIC_CHARGE_COULOMB = 0x2727, 00681 00682 /** 00683 * Electrical potential difference, voltage. 00684 */ 00685 BLE_GATT_UNIT_ELECTRIC_POTENTIAL_DIFFERENCE_VOLT = 0x2728, 00686 00687 /** 00688 * Capacitance, farad. 00689 */ 00690 BLE_GATT_UNIT_CAPACITANCE_FARAD = 0x2729, 00691 00692 /** 00693 * Electric resistance, ohm. 00694 */ 00695 BLE_GATT_UNIT_ELECTRIC_RESISTANCE_OHM = 0x272A, 00696 00697 /** 00698 * Electric conductance, siemens. 00699 */ 00700 BLE_GATT_UNIT_ELECTRIC_CONDUCTANCE_SIEMENS = 0x272B, 00701 00702 /** 00703 * Magnetic flux, weber. 00704 */ 00705 BLE_GATT_UNIT_MAGNETIC_FLEX_WEBER = 0x272C, 00706 00707 /** 00708 * Magnetic flux density, tesla. 00709 */ 00710 BLE_GATT_UNIT_MAGNETIC_FLEX_DENSITY_TESLA = 0x272D, 00711 00712 /** 00713 * Inductance, henry. 00714 */ 00715 BLE_GATT_UNIT_INDUCTANCE_HENRY = 0x272E, 00716 00717 /** 00718 * Celsius temperature, degree Celsius. 00719 */ 00720 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_DEGREE_CELSIUS = 0x272F, 00721 00722 /** 00723 * Luminous flux, lumen. 00724 */ 00725 BLE_GATT_UNIT_LUMINOUS_FLUX_LUMEN = 0x2730, 00726 00727 /** 00728 * Illuminance, lux. 00729 */ 00730 BLE_GATT_UNIT_ILLUMINANCE_LUX = 0x2731, 00731 00732 /** 00733 * Activity referred to a radionuclide, becquerel. 00734 */ 00735 BLE_GATT_UNIT_ACTIVITY_REFERRED_TO_A_RADIONUCLIDE_BECQUEREL = 0x2732, 00736 00737 /** 00738 * Absorbed dose, gray. 00739 */ 00740 BLE_GATT_UNIT_ABSORBED_DOSE_GRAY = 0x2733, 00741 00742 /** 00743 * Dose equivalent, sievert. 00744 */ 00745 BLE_GATT_UNIT_DOSE_EQUIVALENT_SIEVERT = 0x2734, 00746 00747 /** 00748 * Catalytic activity, katal. 00749 */ 00750 BLE_GATT_UNIT_CATALYTIC_ACTIVITY_KATAL = 0x2735, 00751 00752 /** 00753 * Dynamic viscosity, pascal second. 00754 */ 00755 BLE_GATT_UNIT_DYNAMIC_VISCOSITY_PASCAL_SECOND = 0x2740, 00756 00757 /** 00758 * Moment of force, newton meter. 00759 */ 00760 BLE_GATT_UNIT_MOMENT_OF_FORCE_NEWTON_METRE = 0x2741, 00761 00762 /** 00763 * Surface tension, newton per meter. 00764 */ 00765 BLE_GATT_UNIT_SURFACE_TENSION_NEWTON_PER_METRE = 0x2742, 00766 00767 /** 00768 * Angular velocity, radian per second. 00769 */ 00770 BLE_GATT_UNIT_ANGULAR_VELOCITY_RADIAN_PER_SECOND = 0x2743, 00771 00772 /** 00773 * Angular acceleration, radian per second squared. 00774 */ 00775 BLE_GATT_UNIT_ANGULAR_ACCELERATION_RADIAN_PER_SECOND_SQUARED = 0x2744, 00776 00777 /** 00778 * Heat flux density, watt per square meter. 00779 */ 00780 BLE_GATT_UNIT_HEAT_FLUX_DENSITY_WATT_PER_SQUARE_METRE = 0x2745, 00781 00782 /** 00783 * Heat capacity, joule per kelvin. 00784 */ 00785 BLE_GATT_UNIT_HEAT_CAPACITY_JOULE_PER_KELVIN = 0x2746, 00786 00787 /** 00788 * Specific heat capacity, joule per kilogram kelvin. 00789 */ 00790 BLE_GATT_UNIT_SPECIFIC_HEAT_CAPACITY_JOULE_PER_KILOGRAM_KELVIN = 0x2747, 00791 00792 /** 00793 * Specific energy, joule per kilogram. 00794 */ 00795 BLE_GATT_UNIT_SPECIFIC_ENERGY_JOULE_PER_KILOGRAM = 0x2748, 00796 00797 /** 00798 * Thermal conductivity, watt per meter kelvin. 00799 */ 00800 BLE_GATT_UNIT_THERMAL_CONDUCTIVITY_WATT_PER_METRE_KELVIN = 0x2749, 00801 00802 /** 00803 * Energy density, joule per cubic meter. 00804 */ 00805 BLE_GATT_UNIT_ENERGY_DENSITY_JOULE_PER_CUBIC_METRE = 0x274A, 00806 00807 /** 00808 * Electric field strength, volt per meter. 00809 */ 00810 BLE_GATT_UNIT_ELECTRIC_FIELD_STRENGTH_VOLT_PER_METRE = 0x274B, 00811 00812 /** 00813 * Electric charge density, coulomb per cubic meter. 00814 */ 00815 BLE_GATT_UNIT_ELECTRIC_CHARGE_DENSITY_COULOMB_PER_CUBIC_METRE = 0x274C, 00816 00817 /** 00818 * Surface charge density, coulomb per square meter. 00819 */ 00820 BLE_GATT_UNIT_SURFACE_CHARGE_DENSITY_COULOMB_PER_SQUARE_METRE = 0x274D, 00821 00822 /** 00823 * Electric flux density, coulomb per square meter. 00824 */ 00825 BLE_GATT_UNIT_ELECTRIC_FLUX_DENSITY_COULOMB_PER_SQUARE_METRE = 0x274E, 00826 00827 /** 00828 * Permittivity, farad per meter. 00829 */ 00830 BLE_GATT_UNIT_PERMITTIVITY_FARAD_PER_METRE = 0x274F, 00831 00832 /** 00833 * Permeability, henry per meter. 00834 */ 00835 BLE_GATT_UNIT_PERMEABILITY_HENRY_PER_METRE = 0x2750, 00836 00837 /** 00838 * Molar energy, joule per mole. 00839 */ 00840 BLE_GATT_UNIT_MOLAR_ENERGY_JOULE_PER_MOLE = 0x2751, 00841 00842 /** 00843 * Molar entropy, joule per mole kelvin. 00844 */ 00845 BLE_GATT_UNIT_MOLAR_ENTROPY_JOULE_PER_MOLE_KELVIN = 0x2752, 00846 00847 /** 00848 * Exposure, coulomb per kilogram. 00849 */ 00850 BLE_GATT_UNIT_EXPOSURE_COULOMB_PER_KILOGRAM = 0x2753, 00851 00852 /** 00853 * Absorbed dose rate, gray per second. 00854 */ 00855 BLE_GATT_UNIT_ABSORBED_DOSE_RATE_GRAY_PER_SECOND = 0x2754, 00856 00857 /** 00858 * Radiant intensity, watt per steradian. 00859 */ 00860 BLE_GATT_UNIT_RADIANT_INTENSITY_WATT_PER_STERADIAN = 0x2755, 00861 00862 /** 00863 * Radiance, watt per square meter steradian. 00864 */ 00865 BLE_GATT_UNIT_RADIANCE_WATT_PER_SQUARE_METRE_STERADIAN = 0x2756, 00866 00867 /** 00868 * Catalytic activity concentration, katal per cubic meter. 00869 */ 00870 BLE_GATT_UNIT_CATALYTIC_ACTIVITY_CONCENTRATION_KATAL_PER_CUBIC_METRE = 0x2757, 00871 00872 /** 00873 * Time, minute. 00874 */ 00875 BLE_GATT_UNIT_TIME_MINUTE = 0x2760, 00876 00877 /** 00878 * Time, hour. 00879 */ 00880 BLE_GATT_UNIT_TIME_HOUR = 0x2761, 00881 00882 /** 00883 * Time, day. 00884 */ 00885 BLE_GATT_UNIT_TIME_DAY = 0x2762, 00886 00887 /** 00888 * Plane angle, degree. 00889 */ 00890 BLE_GATT_UNIT_PLANE_ANGLE_DEGREE = 0x2763, 00891 00892 /** 00893 * Plane angle, minute. 00894 */ 00895 BLE_GATT_UNIT_PLANE_ANGLE_MINUTE = 0x2764, 00896 00897 /** 00898 * Plane angle, seconds. 00899 */ 00900 BLE_GATT_UNIT_PLANE_ANGLE_SECOND = 0x2765, 00901 00902 /** 00903 * Area, hectare. 00904 */ 00905 BLE_GATT_UNIT_AREA_HECTARE = 0x2766, 00906 00907 /** 00908 * Volume, liter. 00909 */ 00910 BLE_GATT_UNIT_VOLUME_LITRE = 0x2767, 00911 00912 /** 00913 * Mass, ton. 00914 */ 00915 BLE_GATT_UNIT_MASS_TONNE = 0x2768, 00916 00917 /** 00918 * Pressure, bar. 00919 */ 00920 BLE_GATT_UNIT_PRESSURE_BAR = 0x2780, 00921 00922 /** 00923 * Pressure, millimeter of mercury. 00924 */ 00925 BLE_GATT_UNIT_PRESSURE_MILLIMETRE_OF_MERCURY = 0x2781, 00926 00927 /** 00928 * Length, ngstrm. 00929 */ 00930 BLE_GATT_UNIT_LENGTH_ANGSTROM = 0x2782, 00931 00932 /** 00933 * Length, nautical mile. 00934 */ 00935 BLE_GATT_UNIT_LENGTH_NAUTICAL_MILE = 0x2783, 00936 00937 /** 00938 * Area, barn. 00939 */ 00940 BLE_GATT_UNIT_AREA_BARN = 0x2784, 00941 00942 /** 00943 * Velocity, knot. 00944 */ 00945 BLE_GATT_UNIT_VELOCITY_KNOT = 0x2785, 00946 00947 /** 00948 * Logarithmic radio quantity, neper. 00949 */ 00950 BLE_GATT_UNIT_LOGARITHMIC_RADIO_QUANTITY_NEPER = 0x2786, 00951 00952 /** 00953 * Logarithmic radio quantity, bel. 00954 */ 00955 BLE_GATT_UNIT_LOGARITHMIC_RADIO_QUANTITY_BEL = 0x2787, 00956 00957 /** 00958 * Length, yard. 00959 */ 00960 BLE_GATT_UNIT_LENGTH_YARD = 0x27A0, 00961 00962 /** 00963 * Length, parsec. 00964 */ 00965 BLE_GATT_UNIT_LENGTH_PARSEC = 0x27A1, 00966 00967 /** 00968 * Length, inch. 00969 */ 00970 BLE_GATT_UNIT_LENGTH_INCH = 0x27A2, 00971 00972 /** 00973 * Length, foot. 00974 */ 00975 BLE_GATT_UNIT_LENGTH_FOOT = 0x27A3, 00976 00977 /** 00978 * Length, mile. 00979 */ 00980 BLE_GATT_UNIT_LENGTH_MILE = 0x27A4, 00981 00982 /** 00983 * Pressure, pound-force per square inch. 00984 */ 00985 BLE_GATT_UNIT_PRESSURE_POUND_FORCE_PER_SQUARE_INCH = 0x27A5, 00986 00987 /** 00988 * Velocity, kilometer per hour. 00989 */ 00990 BLE_GATT_UNIT_VELOCITY_KILOMETRE_PER_HOUR = 0x27A6, 00991 00992 /** Velocity, mile per hour. 00993 * 00994 */ 00995 BLE_GATT_UNIT_VELOCITY_MILE_PER_HOUR = 0x27A7, 00996 00997 /** 00998 * Angular Velocity, revolution per minute. 00999 */ 01000 BLE_GATT_UNIT_ANGULAR_VELOCITY_REVOLUTION_PER_MINUTE = 0x27A8, 01001 01002 /** 01003 * Energy, gram calorie. 01004 */ 01005 BLE_GATT_UNIT_ENERGY_GRAM_CALORIE = 0x27A9, 01006 01007 /** 01008 * Energy, kilogram calorie. 01009 */ 01010 BLE_GATT_UNIT_ENERGY_KILOGRAM_CALORIE = 0x27AA, 01011 01012 /** 01013 * Energy, killowatt hour. 01014 */ 01015 BLE_GATT_UNIT_ENERGY_KILOWATT_HOUR = 0x27AB, 01016 01017 /** 01018 * Thermodynamic temperature, degree Fahrenheit. 01019 */ 01020 BLE_GATT_UNIT_THERMODYNAMIC_TEMPERATURE_DEGREE_FAHRENHEIT = 0x27AC, 01021 01022 /** 01023 * Percentage. 01024 */ 01025 BLE_GATT_UNIT_PERCENTAGE = 0x27AD, 01026 01027 /** 01028 * Per mille. 01029 */ 01030 BLE_GATT_UNIT_PER_MILLE = 0x27AE, 01031 01032 /** 01033 * Period, beats per minute. 01034 */ 01035 BLE_GATT_UNIT_PERIOD_BEATS_PER_MINUTE = 0x27AF, 01036 01037 /** 01038 * Electric charge, ampere hours. 01039 */ 01040 BLE_GATT_UNIT_ELECTRIC_CHARGE_AMPERE_HOURS = 0x27B0, 01041 01042 /** 01043 * Mass density, milligram per deciliter. 01044 */ 01045 BLE_GATT_UNIT_MASS_DENSITY_MILLIGRAM_PER_DECILITRE = 0x27B1, 01046 01047 /** 01048 * Mass density, millimole per liter. 01049 */ 01050 BLE_GATT_UNIT_MASS_DENSITY_MILLIMOLE_PER_LITRE = 0x27B2, 01051 01052 /** 01053 * Time, year. 01054 */ 01055 BLE_GATT_UNIT_TIME_YEAR = 0x27B3, 01056 01057 /** 01058 * Time, month. 01059 */ 01060 BLE_GATT_UNIT_TIME_MONTH = 0x27B4, 01061 01062 /** 01063 * Concentration, count per cubic meter. 01064 */ 01065 BLE_GATT_UNIT_CONCENTRATION_COUNT_PER_CUBIC_METRE = 0x27B5, 01066 01067 /** 01068 * Irradiance, watt per square meter. 01069 */ 01070 BLE_GATT_UNIT_IRRADIANCE_WATT_PER_SQUARE_METRE = 0x27B6 01071 }; 01072 01073 /** 01074 * Presentation format of a characteristic. 01075 * 01076 * It determines how the value of a characteristic is formatted. A server 01077 * can expose that information to its clients by adding a Characteristic 01078 * Presentation Format descriptor to relevant characteristics. 01079 * 01080 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.3.5.2. 01081 */ 01082 enum { 01083 /** 01084 * Reserved for future use. 01085 */ 01086 BLE_GATT_FORMAT_RFU = 0x00, 01087 01088 /** 01089 * Boolean. 01090 */ 01091 BLE_GATT_FORMAT_BOOLEAN = 0x01, 01092 01093 /** 01094 * Unsigned 2-bit integer. 01095 */ 01096 BLE_GATT_FORMAT_2BIT = 0x02, 01097 01098 /** 01099 * Unsigned 4-bit integer. 01100 */ 01101 BLE_GATT_FORMAT_NIBBLE = 0x03, 01102 01103 /** 01104 * Unsigned 8-bit integer. 01105 */ 01106 BLE_GATT_FORMAT_UINT8 = 0x04, 01107 01108 /** 01109 * Unsigned 12-bit integer. 01110 */ 01111 BLE_GATT_FORMAT_UINT12 = 0x05, 01112 01113 /** 01114 * Unsigned 16-bit integer. 01115 */ 01116 BLE_GATT_FORMAT_UINT16 = 0x06, 01117 01118 /** 01119 * Unsigned 24-bit integer. 01120 */ 01121 BLE_GATT_FORMAT_UINT24 = 0x07, 01122 01123 /** 01124 * Unsigned 32-bit integer. 01125 */ 01126 BLE_GATT_FORMAT_UINT32 = 0x08, 01127 01128 /** 01129 * Unsigned 48-bit integer. 01130 */ 01131 BLE_GATT_FORMAT_UINT48 = 0x09, 01132 01133 /** 01134 * Unsigned 64-bit integer. 01135 */ 01136 BLE_GATT_FORMAT_UINT64 = 0x0A, 01137 01138 /** 01139 * Unsigned 128-bit integer. 01140 */ 01141 BLE_GATT_FORMAT_UINT128 = 0x0B, 01142 01143 /** 01144 * Signed 8-bit integer. 01145 */ 01146 BLE_GATT_FORMAT_SINT8 = 0x0C, 01147 01148 /** 01149 * Signed 12-bit integer. 01150 */ 01151 BLE_GATT_FORMAT_SINT12 = 0x0D, 01152 01153 /** 01154 * Signed 16-bit integer. 01155 */ 01156 BLE_GATT_FORMAT_SINT16 = 0x0E, 01157 01158 /** 01159 * Signed 24-bit integer. 01160 */ 01161 BLE_GATT_FORMAT_SINT24 = 0x0F, 01162 01163 /** 01164 * Signed 32-bit integer. 01165 */ 01166 BLE_GATT_FORMAT_SINT32 = 0x10, 01167 01168 /** 01169 * Signed 48-bit integer. 01170 */ 01171 BLE_GATT_FORMAT_SINT48 = 0x11, 01172 01173 /** 01174 * Signed 64-bit integer. 01175 */ 01176 BLE_GATT_FORMAT_SINT64 = 0x12, 01177 01178 /** 01179 * Signed 128-bit integer. 01180 */ 01181 BLE_GATT_FORMAT_SINT128 = 0x13, 01182 01183 /** 01184 * IEEE-754 32-bit floating point. 01185 */ 01186 BLE_GATT_FORMAT_FLOAT32 = 0x14, 01187 01188 /** 01189 * IEEE-754 64-bit floating point. 01190 */ 01191 BLE_GATT_FORMAT_FLOAT64 = 0x15, 01192 01193 /** 01194 * IEEE-11073 16-bit SFLOAT. 01195 */ 01196 BLE_GATT_FORMAT_SFLOAT = 0x16, 01197 01198 /** 01199 * IEEE-11073 32-bit FLOAT. 01200 */ 01201 BLE_GATT_FORMAT_FLOAT = 0x17, 01202 01203 /** 01204 * IEEE-20601 format. 01205 */ 01206 BLE_GATT_FORMAT_DUINT16 = 0x18, 01207 01208 /** 01209 * UTF8 string. 01210 */ 01211 BLE_GATT_FORMAT_UTF8S = 0x19, 01212 01213 /** 01214 * UTF16 string. 01215 */ 01216 BLE_GATT_FORMAT_UTF16S = 0x1A, 01217 01218 /** 01219 * Opaque Structure. 01220 */ 01221 BLE_GATT_FORMAT_STRUCT = 0x1B 01222 }; 01223 01224 /*! 01225 * Characteristic properties. 01226 * 01227 * It is a bitfield that determines how a characteristic value can be used. 01228 * 01229 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.1.1 01230 * and Section 3.3.3.1 for Extended Properties. 01231 */ 01232 enum Properties_t { 01233 /** 01234 * No property defined. 01235 */ 01236 BLE_GATT_CHAR_PROPERTIES_NONE = 0x00, 01237 01238 /** 01239 * Permits broadcasts of the characteristic value using the Server 01240 * Characteristic Configuration descriptor. 01241 */ 01242 BLE_GATT_CHAR_PROPERTIES_BROADCAST = 0x01, 01243 01244 /** 01245 * Permits reads of the characteristic value. 01246 */ 01247 BLE_GATT_CHAR_PROPERTIES_READ = 0x02, 01248 01249 /** 01250 * Permits writes of the characteristic value without response. 01251 */ 01252 BLE_GATT_CHAR_PROPERTIES_WRITE_WITHOUT_RESPONSE = 0x04, 01253 01254 /** 01255 * Permits writes of the characteristic value with response. 01256 */ 01257 BLE_GATT_CHAR_PROPERTIES_WRITE = 0x08, 01258 01259 /** 01260 * Permits notifications of a characteristic value without acknowledgment. 01261 */ 01262 BLE_GATT_CHAR_PROPERTIES_NOTIFY = 0x10, 01263 01264 /** 01265 * Permits indications of a characteristic value with acknowledgment. 01266 */ 01267 BLE_GATT_CHAR_PROPERTIES_INDICATE = 0x20, 01268 01269 /** 01270 * Permits signed writes to the characteristic value. 01271 */ 01272 BLE_GATT_CHAR_PROPERTIES_AUTHENTICATED_SIGNED_WRITES = 0x40, 01273 01274 /** 01275 * The Characteristic Extended Properties descriptor 01276 * defines additional characteristic properties. 01277 */ 01278 BLE_GATT_CHAR_PROPERTIES_EXTENDED_PROPERTIES = 0x80 01279 01280 }; 01281 01282 /** 01283 * Value of a Characteristic Presentation Format descriptor. 01284 * 01285 * Characteristic Presentation Format descriptor expresses the format of a 01286 * characteristic value. 01287 * 01288 * @note See Bluetooth Specification 4.0 (Vol. 3), Part G, Section 3.3.3.5. 01289 */ 01290 struct PresentationFormat_t { 01291 /** 01292 * Format of the value. 01293 */ 01294 uint8_t gatt_format; 01295 01296 /** 01297 * Exponent for integer data types. 01298 * 01299 * Example: if Exponent = -3 and the char value is 3892, the actual 01300 * value is 3.892 01301 */ 01302 int8_t exponent; 01303 01304 /** 01305 * Unit of the characteristic value. 01306 * 01307 * It is a UUID from Bluetooth Assigned Numbers. 01308 */ 01309 uint16_t gatt_unit; 01310 01311 /** 01312 * Namespace of the description field. 01313 * 01314 * This field identifies the organization that is responsible for 01315 * defining the enumerations for the description field. 01316 * 01317 * The namespace of the Bluetooth Body is 0x01. 01318 */ 01319 uint8_t gatt_namespace; 01320 01321 /** 01322 * Description. 01323 * 01324 * @note The value 0x0000 means unknown in the Bluetooth namespace. 01325 */ 01326 uint16_t gatt_nsdesc; 01327 01328 }; 01329 01330 /** 01331 * @brief Constructs a new GattCharacteristic. 01332 * 01333 * @param[in] uuid The UUID of this characteristic. 01334 * @param[in] valuePtr Memory buffer holding the initial value. The value is 01335 * copied into the Bluetooth subsytem when the enclosing service is added. 01336 * Thereafter, the stack maintains it internally. 01337 * @param[in] len The length in bytes of this characteristic's value. 01338 * @param[in] maxLen The capacity in bytes of the characteristic value 01339 * buffer. 01340 * @param[in] props An 8-bit field that contains the characteristic's 01341 * properties. 01342 * @param[in] descriptors A pointer to an array of descriptors to be included 01343 * within this characteristic. The caller owns the memory for the descriptor 01344 * array, which must remain valid at least until the enclosing service is 01345 * added to the GATT table. 01346 * @param[in] numDescriptors The number of descriptors presents in @p 01347 * descriptors array. 01348 * @param[in] hasVariableLen Flag that indicates if the attribute's value 01349 * length can change throughout time. 01350 * 01351 * @note If valuePtr is NULL, length is equal to 0 and the characteristic 01352 * is readable, then that particular characteristic may be considered 01353 * optional and dropped while instantiating the service with the underlying 01354 * BLE stack. 01355 * 01356 * @note A Client Characteristic Configuration Descriptor (CCCD) should not 01357 * be allocated if either the notify or indicate flag in the @p props bit 01358 * field; the underlying BLE stack handles it. 01359 * 01360 * @important GattCharacteristic registered in a GattServer must remain 01361 * valid for the lifetime of the GattServer. 01362 */ 01363 GattCharacteristic( 01364 const UUID &uuid, 01365 uint8_t *valuePtr = NULL, 01366 uint16_t len = 0, 01367 uint16_t maxLen = 0, 01368 uint8_t props = BLE_GATT_CHAR_PROPERTIES_NONE, 01369 GattAttribute *descriptors[] = NULL, 01370 unsigned numDescriptors = 0, 01371 bool hasVariableLen = true 01372 ) : _valueAttribute(uuid, valuePtr, len, maxLen, hasVariableLen), 01373 _properties(props), 01374 _requiredSecurity(SecurityManager::SECURITY_MODE_ENCRYPTION_OPEN_LINK), 01375 _descriptors(descriptors), 01376 _descriptorCount(numDescriptors), 01377 enabledReadAuthorization(false), 01378 enabledWriteAuthorization(false), 01379 readAuthorizationCallback(), 01380 writeAuthorizationCallback() { 01381 } 01382 01383 public: 01384 /** 01385 * Set up the minimum security (mode and level) requirements for access to 01386 * the characteristic's value attribute. 01387 * 01388 * @param[in] securityMode Can be one of encryption or signing, with or 01389 * without protection for man in the middle attacks (MITM). 01390 */ 01391 void requireSecurity(SecurityManager::SecurityMode_t securityMode) 01392 { 01393 _requiredSecurity = securityMode; 01394 } 01395 01396 public: 01397 /** 01398 * Register a callback handling client's write requests or commands. 01399 * 01400 * The callback registered is invoked when the client attempts to write the 01401 * characteristic value; the event handler can accept or reject the write 01402 * request with the appropriate error code. 01403 * 01404 * @param[in] callback Event handler being registered. 01405 */ 01406 void setWriteAuthorizationCallback( 01407 void (*callback)(GattWriteAuthCallbackParams *) 01408 ) { 01409 writeAuthorizationCallback.attach(callback); 01410 enabledWriteAuthorization = true; 01411 } 01412 01413 /** 01414 * Register a callback handling client's write requests or commands. 01415 * 01416 * The callback registered is invoked when the client attempts to write the 01417 * characteristic value; the event handler can accept or reject the write 01418 * request with the appropriate error code. 01419 * 01420 * @param[in] object Pointer to the object of a class defining the event 01421 * handler (@p member). It must remain valid for the lifetime of the 01422 * GattCharacteristic. 01423 * @param[in] member The member function that handles the write event. 01424 */ 01425 template <typename T> 01426 void setWriteAuthorizationCallback( 01427 T *object, 01428 void (T::*member)(GattWriteAuthCallbackParams *) 01429 ) { 01430 writeAuthorizationCallback.attach(object, member); 01431 enabledWriteAuthorization = true; 01432 } 01433 01434 /** 01435 * Register the read requests event handler. 01436 * 01437 * The callback registered is invoked when the client attempts to read the 01438 * characteristic value; the event handler can accept or reject the read 01439 * request with the appropriate error code. It can also set specific outgoing 01440 * data. 01441 * 01442 * @param[in] callback Event handler being registered. 01443 */ 01444 void setReadAuthorizationCallback( 01445 void (*callback)(GattReadAuthCallbackParams *) 01446 ) { 01447 readAuthorizationCallback.attach(callback); 01448 enabledReadAuthorization = true; 01449 } 01450 01451 /** 01452 * Register the read requests event handler. 01453 * 01454 * The callback registered is invoked when the client attempts to read the 01455 * characteristic value; the event handler can accept or reject the read 01456 * request with the appropriate error code. It can also set specific outgoing 01457 * data. 01458 * 01459 * @param[in] object Pointer to the object of a class defining the event 01460 * handler (@p member). It must remain valid for the lifetime of the 01461 * GattCharacteristic. 01462 * @param[in] member The member function that handles the read event. 01463 */ 01464 template <typename T> 01465 void setReadAuthorizationCallback( 01466 T *object, 01467 void (T::*member)(GattReadAuthCallbackParams *) 01468 ) { 01469 readAuthorizationCallback.attach(object, member); 01470 enabledReadAuthorization = true; 01471 } 01472 01473 /** 01474 * Invoke the write authorization callback. 01475 * 01476 * This function is a helper that calls the registered write handler to 01477 * determine the authorization reply for a write request. 01478 * 01479 * @important This function is not meant to be called by user code. 01480 * 01481 * @param[in] params Context of the write-auth request; it contains an 01482 * out-parameter used as a reply. 01483 * 01484 * @return A GattAuthCallbackReply_t value indicating whether authorization 01485 * is granted. 01486 */ 01487 GattAuthCallbackReply_t authorizeWrite(GattWriteAuthCallbackParams *params) 01488 { 01489 if (!isWriteAuthorizationEnabled()) { 01490 return AUTH_CALLBACK_REPLY_SUCCESS; 01491 } 01492 01493 /* Initialized to no-error by default. */ 01494 params->authorizationReply = AUTH_CALLBACK_REPLY_SUCCESS; 01495 writeAuthorizationCallback.call(params); 01496 return params->authorizationReply; 01497 } 01498 01499 /** 01500 * Invoke the read authorization callback. 01501 * 01502 * This function is a helper that calls the registered read handler to 01503 * determine the authorization reply for a read request. 01504 * 01505 * @important This function is not meant to be called by user code. 01506 * 01507 * @param[in] params Context of the read-auth request; it contains an 01508 * out-parameter used as a reply and the handler can fill it with outgoing 01509 * data. 01510 * 01511 * @return A GattAuthCallbackReply_t value indicating whether authorization 01512 * is granted. 01513 * 01514 * @note If the read request is approved and params->data remains NULL, then 01515 * the current characteristic value is used in the read response payload. 01516 * 01517 * @note If the read is approved, the event handler can specify an outgoing 01518 * value directly with the help of the fields 01519 * GattReadAuthCallbackParams::data and GattReadAuthCallbackParams::len. 01520 */ 01521 GattAuthCallbackReply_t authorizeRead(GattReadAuthCallbackParams *params) 01522 { 01523 if (!isReadAuthorizationEnabled()) { 01524 return AUTH_CALLBACK_REPLY_SUCCESS; 01525 } 01526 01527 /* Initialized to no-error by default. */ 01528 params->authorizationReply = AUTH_CALLBACK_REPLY_SUCCESS; 01529 readAuthorizationCallback.call(params); 01530 return params->authorizationReply; 01531 } 01532 01533 public: 01534 /** 01535 * Get the characteristic's value attribute. 01536 * 01537 * @return A reference to the characteristic's value attribute. 01538 */ 01539 GattAttribute& getValueAttribute() 01540 { 01541 return _valueAttribute; 01542 } 01543 01544 /** 01545 * Get the characteristic's value attribute. 01546 * 01547 * @return A const reference to the characteristic's value attribute. 01548 */ 01549 const GattAttribute& getValueAttribute() const 01550 { 01551 return _valueAttribute; 01552 } 01553 01554 /** 01555 * Get the characteristic's value attribute handle in the ATT table. 01556 * 01557 * @return The value attribute handle. 01558 * 01559 * @note The underlying BLE stack assigns the attribute handle when the 01560 * enclosing service is added. 01561 */ 01562 GattAttribute::Handle_t getValueHandle(void) const 01563 { 01564 return getValueAttribute().getHandle(); 01565 } 01566 01567 /** 01568 * Get the characteristic's properties. 01569 * 01570 * @note Refer to GattCharacteristic::Properties_t. 01571 * 01572 * @return The characteristic's properties. 01573 */ 01574 uint8_t getProperties(void) const 01575 { 01576 return _properties; 01577 } 01578 01579 /** 01580 * Get the characteristic's required security. 01581 * 01582 * @return The characteristic's required security. 01583 */ 01584 SecurityManager::SecurityMode_t getRequiredSecurity() const 01585 { 01586 return _requiredSecurity; 01587 } 01588 01589 /** 01590 * Get the total number of descriptors within this characteristic. 01591 * 01592 * @return The total number of descriptors. 01593 */ 01594 uint8_t getDescriptorCount(void) const 01595 { 01596 return _descriptorCount; 01597 } 01598 01599 /** 01600 * Check whether read authorization is enabled. 01601 * 01602 * Read authorization is enabled when a read authorization event handler is 01603 * set up. 01604 * 01605 * @return true if read authorization is enabled and false otherwise. 01606 */ 01607 bool isReadAuthorizationEnabled() const 01608 { 01609 return enabledReadAuthorization; 01610 } 01611 01612 /** 01613 * Check whether write authorization is enabled. 01614 * 01615 * Write authorization is enabled when a write authorization event handler is 01616 * set up. 01617 * 01618 * @return true if write authorization is enabled, false otherwise. 01619 */ 01620 bool isWriteAuthorizationEnabled() const 01621 { 01622 return enabledWriteAuthorization; 01623 } 01624 01625 /** 01626 * Get this characteristic's descriptor at a specific index. 01627 * 01628 * @param[in] index The index of the descriptor to get. 01629 * 01630 * @return A pointer the requested descriptor if @p index is within the 01631 * range of the descriptor array or NULL otherwise. 01632 */ 01633 GattAttribute *getDescriptor(uint8_t index) 01634 { 01635 if (index = _descriptorCount) { 01636 return NULL; 01637 } 01638 01639 return _descriptors[index]; 01640 } 01641 01642 private: 01643 /** 01644 * Attribute that contains the actual value of this characteristic. 01645 */ 01646 GattAttribute _valueAttribute; 01647 01648 /** 01649 * The characteristic's properties. Refer to 01650 * GattCharacteristic::Properties_t. 01651 */ 01652 uint8_t _properties; 01653 01654 /** 01655 * The characteristic's required security. 01656 */ 01657 SecurityManager::SecurityMode_t _requiredSecurity; 01658 01659 /** 01660 * The characteristic's descriptor attributes. 01661 */ 01662 GattAttribute **_descriptors; 01663 01664 /** 01665 * The number of descriptors in this characteristic. 01666 */ 01667 uint8_t _descriptorCount; 01668 01669 /** 01670 * Whether read authorization is enabled. 01671 */ 01672 bool enabledReadAuthorization; 01673 01674 /** 01675 * Whether write authorization is enabled. 01676 */ 01677 bool enabledWriteAuthorization; 01678 01679 /** 01680 * The registered callback handler for read authorization reply. 01681 */ 01682 FunctionPointerWithContext<GattReadAuthCallbackParams *> 01683 readAuthorizationCallback; 01684 01685 /** 01686 * The registered callback handler for write authorization reply. 01687 */ 01688 FunctionPointerWithContext<GattWriteAuthCallbackParams *> 01689 writeAuthorizationCallback; 01690 01691 private: 01692 /* Disallow copy and assignment. */ 01693 GattCharacteristic(const GattCharacteristic &); 01694 GattCharacteristic& operator=(const GattCharacteristic &); 01695 }; 01696 01697 /** 01698 * Helper class that represents a read only GattCharacteristic. 01699 */ 01700 template <typename T> 01701 class ReadOnlyGattCharacteristic : public GattCharacteristic { 01702 public: 01703 /** 01704 * Construct a ReadOnlyGattCharacteristic. 01705 * 01706 * @param[in] uuid The characteristic's UUID. 01707 * @param[in] valuePtr Pointer to the characteristic's initial value. The 01708 * pointer is reinterpreted as a pointer to an uint8_t buffer. 01709 * @param[in] additionalProperties Additional characteristic properties. By 01710 * default, the properties are set to 01711 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 01712 * @param[in] descriptors An array of pointers to descriptors to be added 01713 * to the new characteristic. 01714 * @param[in] numDescriptors The total number of descriptors in @p 01715 * descriptors. 01716 * 01717 * @note Instances of ReadOnlyGattCharacteristic have a fixed length 01718 * attribute value that equals sizeof(T). For a variable length alternative, 01719 * use GattCharacteristic directly. 01720 */ 01721 ReadOnlyGattCharacteristic<T>( 01722 const UUID &uuid, 01723 T *valuePtr, 01724 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01725 GattAttribute *descriptors[] = NULL, 01726 unsigned numDescriptors = 0 01727 ) : GattCharacteristic( 01728 uuid, 01729 reinterpret_cast<uint8_t *>(valuePtr), 01730 sizeof(T), 01731 sizeof(T), 01732 BLE_GATT_CHAR_PROPERTIES_READ | additionalProperties, 01733 descriptors, 01734 numDescriptors, 01735 false 01736 ) { 01737 } 01738 }; 01739 01740 /** 01741 * Helper class that represents a write only GattCharacteristic. 01742 */ 01743 template <typename T> 01744 class WriteOnlyGattCharacteristic : public GattCharacteristic { 01745 public: 01746 /** 01747 * Construct a WriteOnlyGattCharacteristic. 01748 * 01749 * @param[in] uuid The characteristic's UUID. 01750 * @param[in] valuePtr Pointer to the characteristic's initial value. The 01751 * pointer is reinterpreted as a pointer to an uint8_t buffer. 01752 * @param[in] additionalProperties Additional characteristic properties. By 01753 * default, the properties are set to 01754 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE. 01755 * @param[in] descriptors An array of pointers to descriptors to be added to 01756 * the new characteristic. 01757 * @param[in] numDescriptors The total number of descriptors in @p 01758 * descriptors. 01759 * 01760 * @note Instances of WriteOnlyGattCharacteristic have variable length 01761 * attribute value with maximum size equal to sizeof(T). For a fixed length 01762 * alternative, use GattCharacteristic directly. 01763 */ 01764 WriteOnlyGattCharacteristic<T>( 01765 const UUID &uuid, 01766 T *valuePtr, 01767 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01768 GattAttribute *descriptors[] = NULL, 01769 unsigned numDescriptors = 0 01770 ) : GattCharacteristic( 01771 uuid, 01772 reinterpret_cast<uint8_t *>(valuePtr), 01773 sizeof(T), 01774 sizeof(T), 01775 BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, 01776 descriptors, 01777 numDescriptors 01778 ) { 01779 } 01780 }; 01781 01782 /** 01783 * Helper class that represents a readable and writable GattCharacteristic. 01784 */ 01785 template <typename T> 01786 class ReadWriteGattCharacteristic : public GattCharacteristic { 01787 public: 01788 /** 01789 * Construct a ReadWriteGattCharacteristic. 01790 * 01791 * @param[in] uuid The characteristic's UUID. 01792 * @param[in] valuePtr Pointer to the characteristic's initial value. The 01793 * pointer is reinterpreted as a pointer to an uint8_t buffer. 01794 * @param[in] additionalProperties Additional characteristic properties. By 01795 * default, the properties are set to 01796 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE and 01797 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 01798 * @param[in] descriptors An array of pointers to descriptors to be added to 01799 * the new characteristic. 01800 * @param[in] numDescriptors The total number of descriptors in @p descriptors. 01801 * 01802 * @note Instances of ReadWriteGattCharacteristic have variable length 01803 * attribute value with maximum size equal to sizeof(T). For a fixed length 01804 * alternative, use GattCharacteristic directly. 01805 */ 01806 ReadWriteGattCharacteristic<T>( 01807 const UUID &uuid, 01808 T *valuePtr, 01809 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01810 GattAttribute *descriptors[] = NULL, 01811 unsigned numDescriptors = 0 01812 ) : GattCharacteristic( 01813 uuid, 01814 reinterpret_cast<uint8_t *>(valuePtr), 01815 sizeof(T), 01816 sizeof(T), 01817 BLE_GATT_CHAR_PROPERTIES_READ | BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, 01818 descriptors, 01819 numDescriptors 01820 ) { 01821 } 01822 }; 01823 01824 /** 01825 * Helper class that represents a write-only GattCharacteristic with an array 01826 * value. 01827 */ 01828 template <typename T, unsigned NUM_ELEMENTS> 01829 class WriteOnlyArrayGattCharacteristic : public GattCharacteristic { 01830 public: 01831 /** 01832 * Construct a WriteOnlyGattCharacteristic. 01833 * 01834 * @param[in] uuid The characteristic's UUID. 01835 * @param[in] valuePtr Pointer to an array of length NUM_ELEMENTS containing 01836 * the characteristic's initial value. The pointer is reinterpreted as a 01837 * pointer to an uint8_t buffer. 01838 * @param[in] additionalProperties Additional characteristic properties. By 01839 * default, the properties are set to 01840 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE. 01841 * @param[in] descriptors An array of pointers to descriptors to be added to 01842 * the new characteristic. 01843 * @param[in] numDescriptors The total number of descriptors in @p descriptors. 01844 * 01845 * @note Instances of WriteOnlyGattCharacteristic have variable length 01846 * attribute value with maximum size equal to sizeof(T) * NUM_ELEMENTS. 01847 * For a fixed length alternative, use GattCharacteristic directly. 01848 */ 01849 WriteOnlyArrayGattCharacteristic<T, NUM_ELEMENTS>( 01850 const UUID &uuid, 01851 T valuePtr[NUM_ELEMENTS], 01852 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01853 GattAttribute *descriptors[] = NULL, 01854 unsigned numDescriptors = 0 01855 ) : GattCharacteristic( 01856 uuid, 01857 reinterpret_cast<uint8_t *>(valuePtr), 01858 sizeof(T) * NUM_ELEMENTS, 01859 sizeof(T) * NUM_ELEMENTS, 01860 BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, 01861 descriptors, 01862 numDescriptors 01863 ) { 01864 } 01865 }; 01866 01867 /** 01868 * Helper class that represents a read-only GattCharacteristic with an array 01869 * value. 01870 */ 01871 template <typename T, unsigned NUM_ELEMENTS> 01872 class ReadOnlyArrayGattCharacteristic : public GattCharacteristic { 01873 public: 01874 /** 01875 * Construct a ReadOnlyGattCharacteristic. 01876 * 01877 * @param[in] uuid The characteristic's UUID. 01878 * @param[in] valuePtr Pointer to an array of length NUM_ELEMENTS containing 01879 * the characteristic's initial value. The pointer is reinterpreted as a 01880 * pointer to an uint8_t buffer. 01881 * @param[in] additionalProperties Additional characteristic properties. By 01882 * default, the properties are set to 01883 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 01884 * @param[in] descriptors An array of pointers to descriptors to be added to 01885 * the new characteristic. 01886 * @param[in] numDescriptors The total number of descriptors in @p 01887 * descriptors. 01888 * 01889 * @note Instances of ReadOnlyGattCharacteristic have fixed length 01890 * attribute value that equals sizeof(T) * NUM_ELEMENTS. For a variable 01891 * length alternative, use GattCharacteristic directly. 01892 */ 01893 ReadOnlyArrayGattCharacteristic<T, NUM_ELEMENTS>( 01894 const UUID &uuid, 01895 T valuePtr[NUM_ELEMENTS], 01896 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01897 GattAttribute *descriptors[] = NULL, 01898 unsigned numDescriptors = 0 01899 ) : GattCharacteristic( 01900 uuid, 01901 reinterpret_cast<uint8_t *>(valuePtr), 01902 sizeof(T) * NUM_ELEMENTS, 01903 sizeof(T) * NUM_ELEMENTS, 01904 BLE_GATT_CHAR_PROPERTIES_READ | additionalProperties, 01905 descriptors, 01906 numDescriptors, 01907 false 01908 ) { 01909 } 01910 }; 01911 01912 /** 01913 * Helper class that represents a readable and writable GattCharacteristic with 01914 * an array value. 01915 */ 01916 template <typename T, unsigned NUM_ELEMENTS> 01917 class ReadWriteArrayGattCharacteristic : public GattCharacteristic { 01918 public: 01919 /** 01920 * Construct a ReadWriteGattCharacteristic. 01921 * 01922 * @param[in] uuid The characteristic's UUID. 01923 * @param[in] valuePtr Pointer to an array of length NUM_ELEMENTS containing 01924 * the characteristic's initial value. The pointer is reinterpreted as a 01925 * pointer to an uint8_t buffer. 01926 * @param[in] additionalProperties Additional characteristic properties. By 01927 * default, the properties are set to 01928 * Properties_t::BLE_GATT_CHAR_PROPERTIES_WRITE | 01929 * Properties_t::BLE_GATT_CHAR_PROPERTIES_READ. 01930 * @param[in] descriptors An array of pointers to descriptors to be added to 01931 * the new characteristic. 01932 * @param[in] numDescriptors The total number of descriptors in @p descriptors. 01933 * 01934 * @note Instances of ReadWriteGattCharacteristic have variable length 01935 * attribute value with maximum size equal to sizeof(T) * NUM_ELEMENTS. 01936 * For a fixed length alternative, use GattCharacteristic directly. 01937 */ 01938 ReadWriteArrayGattCharacteristic<T, NUM_ELEMENTS>( 01939 const UUID &uuid, 01940 T valuePtr[NUM_ELEMENTS], 01941 uint8_t additionalProperties = BLE_GATT_CHAR_PROPERTIES_NONE, 01942 GattAttribute *descriptors[] = NULL, 01943 unsigned numDescriptors = 0 01944 ) : GattCharacteristic( 01945 uuid, 01946 reinterpret_cast<uint8_t *>(valuePtr), 01947 sizeof(T) * NUM_ELEMENTS, 01948 sizeof(T) * NUM_ELEMENTS, 01949 BLE_GATT_CHAR_PROPERTIES_READ | BLE_GATT_CHAR_PROPERTIES_WRITE | additionalProperties, 01950 descriptors, 01951 numDescriptors 01952 ) { 01953 } 01954 }; 01955 01956 /** 01957 * @} 01958 * @} 01959 * @} 01960 */ 01961 01962 #endif /* ifndef __GATT_CHARACTERISTIC_H__ */
Generated on Sun Jul 17 2022 08:25:23 by
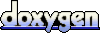