
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
File.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef FILE_H 00018 #define FILE_H 00019 00020 #include "filesystem/FileSystem.h" 00021 #include "platform/FileHandle.h" 00022 00023 namespace mbed { 00024 /** \addtogroup filesystem */ 00025 /** @{*/ 00026 00027 00028 /** File class 00029 */ 00030 class File : public FileHandle { 00031 public: 00032 /** Create an uninitialized file 00033 * 00034 * Must call open to initialize the file on a file system 00035 */ 00036 File(); 00037 00038 /** Create a file on a filesystem 00039 * 00040 * Creates and opens a file on a filesystem 00041 * 00042 * @param fs Filesystem as target for the file 00043 * @param path The name of the file to open 00044 * @param flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, 00045 * bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND 00046 */ 00047 File(FileSystem *fs, const char *path, int flags = O_RDONLY); 00048 00049 /** Destroy a file 00050 * 00051 * Closes file if the file is still open 00052 */ 00053 virtual ~File(); 00054 00055 /** Open a file on the filesystem 00056 * 00057 * @param fs Filesystem as target for the file 00058 * @param path The name of the file to open 00059 * @param flags The flags to open the file in, one of O_RDONLY, O_WRONLY, O_RDWR, 00060 * bitwise or'd with one of O_CREAT, O_TRUNC, O_APPEND 00061 * @return 0 on success, negative error code on failure 00062 */ 00063 virtual int open(FileSystem *fs, const char *path, int flags=O_RDONLY); 00064 00065 /** Close a file 00066 * 00067 * @return 0 on success, negative error code on failure 00068 */ 00069 virtual int close(); 00070 00071 /** Read the contents of a file into a buffer 00072 * 00073 * @param buffer The buffer to read in to 00074 * @param size The number of bytes to read 00075 * @return The number of bytes read, 0 at end of file, negative error on failure 00076 */ 00077 00078 virtual ssize_t read(void *buffer, size_t size); 00079 00080 /** Write the contents of a buffer to a file 00081 * 00082 * @param buffer The buffer to write from 00083 * @param size The number of bytes to write 00084 * @return The number of bytes written, negative error on failure 00085 */ 00086 virtual ssize_t write(const void *buffer, size_t size); 00087 00088 /** Flush any buffers associated with the file 00089 * 00090 * @return 0 on success, negative error code on failure 00091 */ 00092 virtual int sync(); 00093 00094 /** Check if the file in an interactive terminal device 00095 * 00096 * @return True if the file is a terminal 00097 */ 00098 virtual int isatty(); 00099 00100 /** Move the file position to a given offset from from a given location 00101 * 00102 * @param offset The offset from whence to move to 00103 * @param whence The start of where to seek 00104 * SEEK_SET to start from beginning of file, 00105 * SEEK_CUR to start from current position in file, 00106 * SEEK_END to start from end of file 00107 * @return The new offset of the file 00108 */ 00109 virtual off_t seek(off_t offset, int whence = SEEK_SET); 00110 00111 /** Get the file position of the file 00112 * 00113 * @return The current offset in the file 00114 */ 00115 virtual off_t tell(); 00116 00117 /** Rewind the file position to the beginning of the file 00118 * 00119 * @note This is equivalent to file_seek(file, 0, FS_SEEK_SET) 00120 */ 00121 virtual void rewind(); 00122 00123 /** Get the size of the file 00124 * 00125 * @return Size of the file in bytes 00126 */ 00127 virtual off_t size(); 00128 00129 private: 00130 FileSystem *_fs; 00131 fs_file_t _file; 00132 }; 00133 00134 00135 /** @}*/ 00136 } // namespace mbed 00137 00138 #endif
Generated on Sun Jul 17 2022 08:25:23 by
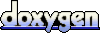