
Rtos API example
Embed:
(wiki syntax)
Show/hide line numbers
CallChain.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_CALLCHAIN_H 00017 #define MBED_CALLCHAIN_H 00018 00019 #include "platform/Callback.h" 00020 #include "platform/mbed_toolchain.h" 00021 #include "platform/NonCopyable.h" 00022 #include <string.h> 00023 00024 namespace mbed { 00025 00026 00027 typedef Callback<void()> *pFunctionPointer_t; 00028 class CallChainLink; 00029 00030 /** \addtogroup platform */ 00031 /** @{*/ 00032 /** 00033 * \defgroup platform_CallChain CallChain class 00034 * @{ 00035 */ 00036 00037 /** Group one or more functions in an instance of a CallChain, then call them in 00038 * sequence using CallChain::call(). Used mostly by the interrupt chaining code, 00039 * but can be used for other purposes. 00040 * 00041 * @note Synchronization level: Not protected 00042 * 00043 * Example: 00044 * @code 00045 * #include "mbed.h" 00046 * 00047 * CallChain chain; 00048 * 00049 * void first(void) { 00050 * printf("'first' function.\n"); 00051 * } 00052 * 00053 * void second(void) { 00054 * printf("'second' function.\n"); 00055 * } 00056 * 00057 * class Test { 00058 * public: 00059 * void f(void) { 00060 * printf("A::f (class member).\n"); 00061 * } 00062 * }; 00063 * 00064 * int main() { 00065 * Test test; 00066 * 00067 * chain.add(second); 00068 * chain.add_front(first); 00069 * chain.add(&test, &Test::f); 00070 * chain.call(); 00071 * } 00072 * @endcode 00073 */ 00074 class CallChain : private NonCopyable<CallChain> { 00075 public: 00076 /** Create an empty chain 00077 * 00078 * @param size (optional) Initial size of the chain 00079 */ 00080 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00081 "public API of mbed-os and is being removed in the future.") 00082 CallChain(int size = 4); 00083 00084 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00085 "public API of mbed-os and is being removed in the future.") 00086 virtual ~CallChain(); 00087 00088 /** Add a function at the end of the chain 00089 * 00090 * @param func A pointer to a void function 00091 * 00092 * @returns 00093 * The function object created for 'func' 00094 */ 00095 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00096 "public API of mbed-os and is being removed in the future.") 00097 pFunctionPointer_t add(Callback<void()> func); 00098 00099 /** Add a function at the end of the chain 00100 * 00101 * @param obj pointer to the object to call the member function on 00102 * @param method pointer to the member function to be called 00103 * 00104 * @returns 00105 * The function object created for 'obj' and 'method' 00106 * 00107 * @deprecated 00108 * The add function does not support cv-qualifiers. Replaced by 00109 * add(callback(obj, method)). 00110 */ 00111 template<typename T, typename M> 00112 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00113 "The add function does not support cv-qualifiers. Replaced by " 00114 "add(callback(obj, method)).") 00115 pFunctionPointer_t add(T *obj, M method) { 00116 return add(callback(obj, method)); 00117 } 00118 00119 /** Add a function at the beginning of the chain 00120 * 00121 * @param func A pointer to a void function 00122 * 00123 * @returns 00124 * The function object created for 'func' 00125 */ 00126 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00127 "public API of mbed-os and is being removed in the future.") 00128 pFunctionPointer_t add_front(Callback<void()> func); 00129 00130 /** Add a function at the beginning of the chain 00131 * 00132 * @param obj pointer to the object to call the member function on 00133 * @param method pointer to the member function to be called 00134 * 00135 * @returns 00136 * The function object created for 'tptr' and 'mptr' 00137 * 00138 * @deprecated 00139 * The add_front function does not support cv-qualifiers. Replaced by 00140 * add_front(callback(obj, method)). 00141 */ 00142 template<typename T, typename M> 00143 MBED_DEPRECATED_SINCE("mbed-os-5.1", 00144 "The add_front function does not support cv-qualifiers. Replaced by " 00145 "add_front(callback(obj, method)).") 00146 pFunctionPointer_t add_front(T *obj, M method) { 00147 return add_front(callback(obj, method)); 00148 } 00149 00150 /** Get the number of functions in the chain 00151 */ 00152 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00153 "public API of mbed-os and is being removed in the future.") 00154 int size() const; 00155 00156 /** Get a function object from the chain 00157 * 00158 * @param i function object index 00159 * 00160 * @returns 00161 * The function object at position 'i' in the chain 00162 */ 00163 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00164 "public API of mbed-os and is being removed in the future.") 00165 pFunctionPointer_t get(int i) const; 00166 00167 /** Look for a function object in the call chain 00168 * 00169 * @param f the function object to search 00170 * 00171 * @returns 00172 * The index of the function object if found, -1 otherwise. 00173 */ 00174 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00175 "public API of mbed-os and is being removed in the future.") 00176 int find(pFunctionPointer_t f) const; 00177 00178 /** Clear the call chain (remove all functions in the chain). 00179 */ 00180 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00181 "public API of mbed-os and is being removed in the future.") 00182 void clear(); 00183 00184 /** Remove a function object from the chain 00185 * 00186 * @arg f the function object to remove 00187 * 00188 * @returns 00189 * true if the function object was found and removed, false otherwise. 00190 */ 00191 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00192 "public API of mbed-os and is being removed in the future.") 00193 bool remove(pFunctionPointer_t f); 00194 00195 /** Call all the functions in the chain in sequence 00196 */ 00197 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00198 "public API of mbed-os and is being removed in the future.") 00199 void call(); 00200 00201 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00202 "public API of mbed-os and is being removed in the future.") 00203 void operator ()(void) { 00204 call(); 00205 } 00206 00207 MBED_DEPRECATED_SINCE("mbed-os-5.6", "This class is not part of the " 00208 "public API of mbed-os and is being removed in the future.") 00209 pFunctionPointer_t operator [](int i) const { 00210 return get(i); 00211 } 00212 00213 private: 00214 CallChainLink *_chain; 00215 }; 00216 00217 /**@}*/ 00218 00219 /**@}*/ 00220 00221 } // namespace mbed 00222 00223 #endif 00224
Generated on Sun Jul 17 2022 08:25:20 by
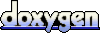