a
Embed:
(wiki syntax)
Show/hide line numbers
UARTSerial_mio.h
00001 00002 #ifndef MBED_UARTSERIAL_MIO_H 00003 #define MBED_UARTSERIAL_MIO_H 00004 00005 #include "platform/platform.h" 00006 00007 #if (DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00008 00009 #include "platform/FileHandle.h" 00010 #include "SerialBase.h" 00011 #include "InterruptIn.h" 00012 #include "platform/PlatformMutex.h" 00013 #include "hal/serial_api.h" 00014 #include "platform/CircularBuffer.h" 00015 #include "platform/NonCopyable.h" 00016 00017 #include "mbed.h" 00018 00019 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 00020 #define MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 256 00021 #endif 00022 00023 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 00024 #define MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 256 00025 #endif 00026 00027 namespace mbed { 00028 00029 /** \addtogroup drivers */ 00030 00031 /** Class providing buffered UART communication functionality using separate circular buffer for send and receive channels 00032 * 00033 * @ingroup drivers 00034 */ 00035 00036 class UARTSerial_mio : private SerialBase, public FileHandle, private NonCopyable<UARTSerial_mio> { 00037 00038 public: 00039 00040 /** Create a UARTSerial_mio port, connected to the specified transmit and receive pins, with a particular baud rate. 00041 * @param tx Transmit pin 00042 * @param rx Receive pin 00043 * @param baud The baud rate of the serial port (optional, defaults to MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE) 00044 */ 00045 UARTSerial_mio(PinName tx, PinName rx, int baud = MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE); 00046 virtual ~UARTSerial_mio(); 00047 00048 /** Equivalent to POSIX poll(). Derived from FileHandle. 00049 * Provides a mechanism to multiplex input/output over a set of file handles. 00050 */ 00051 virtual short poll(short events) const; 00052 00053 /* Resolve ambiguities versus our private SerialBase 00054 * (for writable, spelling differs, but just in case) 00055 */ 00056 using FileHandle::readable; 00057 using FileHandle::writable; 00058 00059 /** Write the contents of a buffer to a file 00060 * 00061 * Follows POSIX semantics: 00062 * 00063 * * if blocking, block until all data is written 00064 * * if no data can be written, and non-blocking set, return -EAGAIN 00065 * * if some data can be written, and non-blocking set, write partial 00066 * 00067 * @param buffer The buffer to write from 00068 * @param length The number of bytes to write 00069 * @return The number of bytes written, negative error on failure 00070 */ 00071 virtual ssize_t write(const void *buffer, size_t length); 00072 00073 /** Read the contents of a file into a buffer 00074 * 00075 * Follows POSIX semantics: 00076 * 00077 * * if no data is available, and non-blocking set return -EAGAIN 00078 * * if no data is available, and blocking set, wait until data is available 00079 * * If any data is available, call returns immediately 00080 * 00081 * @param buffer The buffer to read in to 00082 * @param length The number of bytes to read 00083 * @return The number of bytes read, 0 at end of file, negative error on failure 00084 */ 00085 virtual ssize_t read(void *buffer, size_t length); 00086 virtual ssize_t read_timeout(void *buffer, size_t length, double _timeOut); 00087 00088 /** Close a file 00089 * 00090 * @return 0 on success, negative error code on failure 00091 */ 00092 virtual int close(); 00093 00094 /** Check if the file in an interactive terminal device 00095 * 00096 * @return True if the file is a terminal 00097 * @return False if the file is not a terminal 00098 * @return Negative error code on failure 00099 */ 00100 virtual int isatty(); 00101 00102 /** Move the file position to a given offset from from a given location 00103 * 00104 * Not valid for a device type FileHandle like UARTSerial_mio. 00105 * In case of UARTSerial_mio, returns ESPIPE 00106 * 00107 * @param offset The offset from whence to move to 00108 * @param whence The start of where to seek 00109 * SEEK_SET to start from beginning of file, 00110 * SEEK_CUR to start from current position in file, 00111 * SEEK_END to start from end of file 00112 * @return The new offset of the file, negative error code on failure 00113 */ 00114 virtual off_t seek(off_t offset, int whence); 00115 00116 /** Flush any buffers associated with the file 00117 * 00118 * @return 0 on success, negative error code on failure 00119 */ 00120 virtual int sync(); 00121 virtual int flush(); 00122 /** Set blocking or non-blocking mode 00123 * The default is blocking. 00124 * 00125 * @param blocking true for blocking mode, false for non-blocking mode. 00126 */ 00127 virtual int set_blocking(bool blocking) 00128 { 00129 _blocking = blocking; 00130 return 0; 00131 } 00132 00133 /** Check current blocking or non-blocking mode for file operations. 00134 * 00135 * @return true for blocking mode, false for non-blocking mode. 00136 */ 00137 virtual bool is_blocking() const 00138 { 00139 return _blocking; 00140 } 00141 00142 /** Enable or disable input 00143 * 00144 * Control enabling of device for input. This is primarily intended 00145 * for temporary power-saving; the overall ability of the device to operate for 00146 * input and/or output may be fixed at creation time, but this call can 00147 * allow input to be temporarily disabled to permit power saving without 00148 * losing device state. 00149 * 00150 * @param enabled true to enable input, false to disable. 00151 * 00152 * @return 0 on success 00153 * @return Negative error code on failure 00154 */ 00155 virtual int enable_input(bool enabled); 00156 00157 /** Enable or disable output 00158 * 00159 * Control enabling of device for output. This is primarily intended 00160 * for temporary power-saving; the overall ability of the device to operate for 00161 * input and/or output may be fixed at creation time, but this call can 00162 * allow output to be temporarily disabled to permit power saving without 00163 * losing device state. 00164 * 00165 * @param enabled true to enable output, false to disable. 00166 * 00167 * @return 0 on success 00168 * @return Negative error code on failure 00169 */ 00170 virtual int enable_output(bool enabled); 00171 00172 /** Register a callback on state change of the file. 00173 * 00174 * The specified callback will be called on state changes such as when 00175 * the file can be written to or read from. 00176 * 00177 * The callback may be called in an interrupt context and should not 00178 * perform expensive operations. 00179 * 00180 * Note! This is not intended as an attach-like asynchronous api, but rather 00181 * as a building block for constructing such functionality. 00182 * 00183 * The exact timing of when the registered function 00184 * is called is not guaranteed and susceptible to change. It should be used 00185 * as a cue to make read/write/poll calls to find the current state. 00186 * 00187 * @param func Function to call on state change 00188 */ 00189 virtual void sigio(Callback<void()> func); 00190 00191 /** Setup interrupt handler for DCD line 00192 * 00193 * If DCD line is connected, an IRQ handler will be setup. 00194 * Does nothing if DCD is NC, i.e., not connected. 00195 * 00196 * @param dcd_pin Pin-name for DCD 00197 * @param active_high a boolean set to true if DCD polarity is active low 00198 */ 00199 void set_data_carrier_detect(PinName dcd_pin, bool active_high = false); 00200 00201 /** Set the baud rate 00202 * 00203 * @param baud The baud rate 00204 */ 00205 void set_baud(int baud); 00206 00207 // Expose private SerialBase::Parity as UARTSerial_mio::Parity 00208 using SerialBase::Parity; 00209 // In C++11, we wouldn't need to also have using directives for each value 00210 using SerialBase::None; 00211 using SerialBase::Odd; 00212 using SerialBase::Even; 00213 using SerialBase::Forced1; 00214 using SerialBase::Forced0; 00215 00216 /** Set the transmission format used by the serial port 00217 * 00218 * @param bits The number of bits in a word (5-8; default = 8) 00219 * @param parity The parity used (None, Odd, Even, Forced1, Forced0; default = None) 00220 * @param stop_bits The number of stop bits (1 or 2; default = 1) 00221 */ 00222 void set_format(int bits = 8, Parity parity = UARTSerial_mio::None, int stop_bits = 1); 00223 00224 #if DEVICE_SERIAL_FC 00225 // For now use the base enum - but in future we may have extra options 00226 // such as XON/XOFF or manual GPIO RTSCTS. 00227 using SerialBase::Flow; 00228 // In C++11, we wouldn't need to also have using directives for each value 00229 using SerialBase::Disabled; 00230 using SerialBase::RTS; 00231 using SerialBase::CTS; 00232 using SerialBase::RTSCTS; 00233 00234 /** Set the flow control type on the serial port 00235 * 00236 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00237 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00238 * @param flow2 the second flow control pin (CTS for RTSCTS) 00239 */ 00240 void set_flow_control(Flow type, PinName flow1 = NC, PinName flow2 = NC); 00241 #endif 00242 00243 private: 00244 00245 void wait_ms(uint32_t millisec); 00246 void wait_us(uint32_t microsec); 00247 00248 /** SerialBase lock override */ 00249 virtual void lock(void); 00250 00251 /** SerialBase unlock override */ 00252 virtual void unlock(void); 00253 00254 /** Acquire mutex */ 00255 virtual void api_lock(void); 00256 00257 /** Release mutex */ 00258 virtual void api_unlock(void); 00259 00260 /** Unbuffered write - invoked when write called from critical section */ 00261 ssize_t write_unbuffered(const char *buf_ptr, size_t length); 00262 00263 void enable_rx_irq(); 00264 void disable_rx_irq(); 00265 void enable_tx_irq(); 00266 void disable_tx_irq(); 00267 00268 /** Software serial buffers 00269 * By default buffer size is 256 for TX and 256 for RX. Configurable through mbed_app.json 00270 */ 00271 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE> _rxbuf; 00272 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE> _txbuf; 00273 00274 PlatformMutex _mutex; 00275 00276 Callback<void()> _sigio_cb; 00277 00278 bool _blocking; 00279 bool _tx_irq_enabled; 00280 bool _rx_irq_enabled; 00281 bool _tx_enabled; 00282 bool _rx_enabled; 00283 InterruptIn *_dcd_irq; 00284 00285 /** Device Hanged up 00286 * Determines if the device hanged up on us. 00287 * 00288 * @return True, if hanged up 00289 */ 00290 bool hup() const; 00291 00292 /** ISRs for serial 00293 * Routines to handle interrupts on serial pins. 00294 * Copies data into Circular Buffer. 00295 * Reports the state change to File handle. 00296 */ 00297 void tx_irq(void); 00298 void rx_irq(void); 00299 00300 void wake(void); 00301 00302 void dcd_irq(void); 00303 00304 }; 00305 } //namespace mbed 00306 00307 #endif //(DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00308 #endif //MBED_UARTSERIAL_H
Generated on Tue Aug 16 2022 09:44:47 by
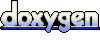