a
Embed:
(wiki syntax)
Show/hide line numbers
RoboClaw.h
00001 #ifndef ROBOCLAW_H 00002 #define ROBOCLAW_H 00003 #include "mbed.h" 00004 #include "registers.h" 00005 #include "UARTSerial_mio.h" 00006 /** The RoboClaw class, yo ucan use the library with : http://www.ionmc.com/RoboClaw-2x15A-Motor-Controller_p_10.html 00007 * Used to control one or two motors with (or not) encoders 00008 * @code 00009 * #include "mbed.h" 00010 * #include "RoboClaw.h" 00011 * 00012 * RoboClaw roboclaw(115200, PA_11, PA_12); 00013 * 00014 * int main() { 00015 * roboclaw.ForwardM1(ADR, 127); 00016 * while(1); 00017 * } 00018 * @endcode 00019 */ 00020 00021 class RoboClaw 00022 { 00023 public: 00024 /** Create RoboClaw instance 00025 */ 00026 RoboClaw(uint8_t adr, int baudrate, PinName rx, PinName tx, double _read_timeout); 00027 00028 /** Forward and Backward functions 00029 * @param address address of the device 00030 * @param speed speed of the motor (between 0 and 127) 00031 * @note Forward and Backward functions 00032 */ 00033 void ForwardM1(int speed); 00034 void BackwardM1(int speed); 00035 void ForwardM2(int speed); 00036 void BackwardM2(int speed); 00037 00038 /** Forward and Backward functions 00039 * @param address address of the device 00040 * @param speed speed of the motor (between 0 and 127) 00041 * @note Forward and Backward functions, it turns the two motors 00042 */ 00043 void Forward(int speed); 00044 void Backward(int speed); 00045 00046 /** Read the Firmware 00047 * @param address address of the device 00048 */ 00049 void ReadFirm(); 00050 00051 /** Read encoder and speed of M1 or M2 00052 * @param address address of the device 00053 * @note Read encoder in ticks 00054 * @note Read speed in ticks per second 00055 */ 00056 bool ReadEncM1(int32_t &encPulse); 00057 bool ReadEncM2(int32_t &encPulse); 00058 bool ReadSpeedM1(int32_t &speed); 00059 bool ReadSpeedM2(int32_t &speed); 00060 bool ReadCurrentM1M2(int32_t ¤tM1, int32_t ¤tM2); 00061 00062 00063 /** Set both encoders to zero 00064 * @param address address of the device 00065 */ 00066 void ResetEnc(); 00067 00068 /** Set speed of Motor with different parameter (only in ticks) 00069 * @param address address of the device 00070 * @note Set the Speed 00071 * @note Set the Speed and Accel 00072 * @note Set the Speed and Distance 00073 * @note Set the Speed, Accel and Distance 00074 * @note Set the Speed, Accel, Decceleration and Position 00075 */ 00076 void SpeedM1(int32_t speed); 00077 void SpeedM2(int32_t speed); 00078 void SpeedAccelM1(int32_t accel, int32_t speed); 00079 void SpeedAccelM2(int32_t accel, int32_t speed); 00080 void SpeedAccelM1M2(int32_t accel, int32_t speed1, int32_t speed2); 00081 void SpeedDistanceM1(int32_t speed, uint32_t distance, uint8_t buffer); 00082 void SpeedDistanceM2(int32_t speed, uint32_t distance, uint8_t buffer); 00083 void SpeedAccelDistanceM1(int32_t accel, int32_t speed, uint32_t distance, uint8_t buffer); 00084 void SpeedAccelDistanceM2(int32_t accel, int32_t speed, uint32_t distance, uint8_t buffer); 00085 void SpeedAccelDeccelPositionM1(uint32_t accel, int32_t speed, uint32_t deccel, int32_t position, uint8_t flag); 00086 void SpeedAccelDeccelPositionM2(uint32_t accel, int32_t speed, uint32_t deccel, int32_t position, uint8_t flag); 00087 void SpeedAccelDeccelPositionM1M2(uint32_t accel1,uint32_t speed1,uint32_t deccel1, int32_t position1,uint32_t accel2,uint32_t speed2,uint32_t deccel2, int32_t position2,uint8_t flag); 00088 00089 private: 00090 UARTSerial_mio _roboclaw; 00091 uint16_t crc; 00092 uint8_t address; 00093 void crc_clear(); 00094 void crc_update(uint8_t data); 00095 uint16_t crc_get(); 00096 00097 void write_n(uint8_t cnt, ...); 00098 void write_(uint8_t command, uint8_t data, bool reading, bool crcon); 00099 00100 uint16_t crc16(uint8_t *packet, int nBytes); 00101 int16_t read_(void); 00102 bool read_n(uint8_t address, uint8_t cmd, int n_byte, int32_t &value); 00103 void flushSerialBuffer(void); 00104 void Rx_interrupt(); 00105 // Circular buffers for serial TX and RX data - used by interrupt routines 00106 const int buffer_size = 7; 00107 // might need to increase buffer size for high baud rates 00108 char rx_buffer[7]; 00109 // Circular buffer pointers 00110 // volatile makes read-modify-write atomic 00111 //Timer readTimer; 00112 //double readTime; 00113 //double readTimePrec; 00114 volatile int rx_in; 00115 double read_timeout; 00116 // Line buffers for sprintf and sscanf 00117 00118 char rx_line[80]; 00119 }; 00120 00121 #endif
Generated on Tue Aug 16 2022 09:44:47 by
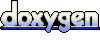