Port of teensy 3 FastCRC library, uses hardware CRC
Embed:
(wiki syntax)
Show/hide line numbers
FastCRC_cpu.h
00001 /* FastCRC library code is placed under the MIT license 00002 * Copyright (c) 2014,2015 Frank Bösing 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining 00005 * a copy of this software and associated documentation files (the 00006 * "Software"), to deal in the Software without restriction, including 00007 * without limitation the rights to use, copy, modify, merge, publish, 00008 * distribute, sublicense, and/or sell copies of the Software, and to 00009 * permit persons to whom the Software is furnished to do so, subject to 00010 * the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be 00013 * included in all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00016 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00017 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00018 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS 00019 * BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN 00020 * ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN 00021 * CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00022 * SOFTWARE. 00023 */ 00024 00025 00026 // CPU-specific implementations of helper functions 00027 00028 #if !defined(__CORTEX_M4) 00029 #if !defined(FastCRC_cpu) 00030 #define FastCRC_cpu 00031 00032 //Reverse byte order (16 bit) 00033 #if defined(__thumb__) 00034 static __attribute__((section(".rev16_text"))) __INLINE __ASM uint32_t REV16(uint32_t value) 00035 { 00036 rev16 r0, r0 00037 bx lr 00038 } 00039 #else 00040 static inline __attribute__((always_inline)) 00041 unsigned int REV16( unsigned int value) //generic 00042 { 00043 return (value >> 8) | ((value & 0xff) << 8); 00044 } 00045 #endif 00046 00047 00048 00049 00050 00051 //Reverse byte order (32 bit) 00052 #if defined(__thumb__) 00053 static __attribute__((section(".rev16_text"))) __INLINE __ASM uint32_t REV32(uint32_t value) 00054 { 00055 rev r0, r0 00056 bx lr 00057 } 00058 #else 00059 static inline __attribute__((always_inline)) 00060 unsigned int REV32( unsigned int value) //generic 00061 { 00062 value = (value >> 16) | ((value & 0xffff) << 16); 00063 return ((value >> 8) & 0xff00ff) | ((value & 0xff00ff) << 8); 00064 } 00065 #endif 00066 00067 00068 #endif 00069 #endif
Generated on Mon Jul 25 2022 09:04:30 by
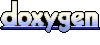