Dependents: Lab3Translator lab3_Radio_design Sync WeatherPlatform_20110408 ... more
HTTPText.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 HTTP Text data source/sink header file 00026 */ 00027 00028 #ifndef HTTP_TEXT_H 00029 #define HTTP_TEXT_H 00030 00031 #include "../HTTPData.h" 00032 #include "mbed.h" 00033 00034 #define DEFAULT_MAX_MEM_ALLOC 512 //Avoid out-of-memory problems 00035 00036 ///HTTP Client data container for text 00037 /** 00038 This is a simple "Text" data repository for HTTP requests. 00039 */ 00040 class HTTPText : public HTTPData //Simple Text I/O 00041 { 00042 public: 00043 ///Instantiates the object. 00044 /** 00045 @param encoding encoding of the data, it defaults to text/html. 00046 @param maxSize defines the maximum memory size that can be allocated by the object. It defaults to 512 bytes. 00047 */ 00048 HTTPText(const string& encoding = "text/html", int maxSize = DEFAULT_MAX_MEM_ALLOC); 00049 virtual ~HTTPText(); 00050 00051 ///Gets text 00052 /** 00053 Returns the text in the container as a zero-terminated char*. 00054 The array returned points to the internal buffer of the object and remains owned by the object. 00055 */ 00056 const char* gets() const; 00057 00058 //Puts text 00059 /** 00060 Sets the text in the container using a zero-terminated char*. 00061 */ 00062 void puts(const char* str); 00063 00064 ///Gets text 00065 /** 00066 Returns the text in the container as string. 00067 */ 00068 string& get(); 00069 00070 ///Puts text 00071 /** 00072 Sets the text in the container as string. 00073 */ 00074 void set(const string& str); 00075 00076 ///Clears the content. 00077 /** 00078 If this container is used as a data sink, it is cleared by the HTTP Client at the beginning of the request. 00079 */ 00080 virtual void clear(); 00081 00082 protected: 00083 virtual int read(char* buf, int len); 00084 virtual int write(const char* buf, int len); 00085 00086 virtual string getDataType(); //Internet media type for Content-Type header 00087 virtual void setDataType(const string& type); //Internet media type from Content-Type header 00088 00089 virtual bool getIsChunked(); //For Transfer-Encoding header 00090 virtual void setIsChunked(bool chunked); //From Transfer-Encoding header 00091 00092 virtual int getDataLen(); //For Content-Length header 00093 virtual void setDataLen(int len); //From Content-Length header, or if the transfer is chunked, next chunk length 00094 00095 private: 00096 string m_buf; 00097 string m_encoding; 00098 int m_maxSize; 00099 }; 00100 00101 #endif
Generated on Fri Jul 15 2022 06:20:13 by
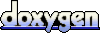