
Successful acro and level mode now! Relying on MPU9250 as base sensor. I'm working continuously on tuning and features :) NEWEST VERSION ON: https://github.com/MaEtUgR/FlyBed (CODE 100% compatible/copyable)
PID.h
00001 // by MaEtUgR 00002 00003 #ifndef PID_H 00004 #define PID_H 00005 00006 #include "mbed.h" 00007 00008 #define BUFFERSIZE 5 00009 00010 class PID { 00011 public: 00012 PID(float P, float I, float D, float Integral_Max); 00013 void compute(float SetPoint, float ProcessValue); 00014 void setIntegrate(bool Integrate); 00015 void setPID(float P, float I, float D); 00016 00017 float Value; 00018 00019 private: 00020 float P, I, D; // PID Values and limits 00021 00022 Timer dtTimer; // Timer to measure time between every compute 00023 float LastTime; // Time when last loop was 00024 00025 float Integral; // the sum of all errors (constaind so it doesn't get infinite) 00026 float Integral_Max; // maximum that the sum of all errors can get (not important: last error not counted) 00027 bool Integrate; // if the integral is used / the controller is in use 00028 00029 float PreviousError; // the Error of the last computation to get derivative 00030 float RollBuffer[BUFFERSIZE]; // Rollingbufferarray for derivative to filter noise 00031 int RollBufferIndex; 00032 }; 00033 00034 #endif
Generated on Tue Jul 12 2022 20:19:36 by
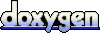