
This is the code used on my video series "Hybrid Supercapacitor Car Battery" for my own hardware monitoring system. THe videos can be found on madelectronengineering.com
Dependencies: BurstSPI Fonts INA219 mbed LPC1114_WakeInterruptIn
Fork of SharpMemoryLCD by
SharpLCD.h
00001 00002 #ifndef MBED_SharpLCD_H 00003 #define MBED_SharpLCD_H 00004 00005 #include "mbed.h" 00006 #include <stdarg.h> 00007 #include "BurstSPI.h" 00008 00009 #define incx() x++, dxt += d2xt, t += dxt 00010 #define incy() y--, dyt += d2yt, t += dyt 00011 00012 /// Set the display geometry depending on the resolution of the display used 00013 /// common types are 96x96, 128x128, 400x240 00014 /** MemoryLCD width in pixels */ 00015 #define DISPLAY_WIDTH (400) 00016 /** MemoryLCD height in pixels */ 00017 #define DISPLAY_HEIGHT (240) 00018 00019 /** Maximum length of a printf to the display */ 00020 #define MAX_PRINTF_CHARS 40 00021 /** Data type for storing buffer the pixel buffer */ 00022 #define DISPLAY_BUFFER_TYPE uint8_t 00023 #define DISPLAY_BUFFER_TYPE_SIZE (sizeof(DISPLAY_BUFFER_TYPE) * 8) 00024 #define DISPLAY_BUFFER_ELEMENTS ((DISPLAY_WIDTH*DISPLAY_HEIGHT)/DISPLAY_BUFFER_TYPE_SIZE) 00025 00026 /** Color definitions */ 00027 #define White 0xFFFF 00028 #define Black 0x0000 00029 #define foreground White 00030 #define background Black 00031 00032 /** Sharp Memory LCD. 00033 * Supports 96x96, 128x128, 400x240 displays. 00034 * Uses a Frame Buffer in RAM depending on resolution of display as follows: 00035 * 96 Display - 1.7k, 128 Display - 2.6k, 400 Display - 12.4k 00036 * 00037 * Example: 00038 * @code 00039 #include "mbed.h" 00040 #include "SharpLCD.h" 00041 00042 //KL25 00043 SharpLCD display(PTC6, NC, PTC5, PTC3, PTC4, NC); //mosi, miso(not used), sck, cs, enable, extcom 00044 00045 int main() 00046 { 00047 display.enableDisplay(); 00048 00049 while(1) 00050 { 00051 display.clearImmediate(); 00052 // draw ellipse (To draw circle, set the last two axis the same) 00053 for (int j=0; j<60; j++) { 00054 display.ellipse(64,64,j,60,Black); 00055 display.update(); 00056 //wait(.01); 00057 display.ellipse(64,64,j,50,White); // offset last axis here gives interesting effect! 00058 display.locate(5,6); 00059 display.printf("Sharp"); 00060 display.locate(2,8); 00061 display.printf("Draw Ellipse"); 00062 display.locate(4,10); 00063 display.printf("MemoryLCD"); 00064 display.update(); 00065 } 00066 // draw rectangle 00067 for (int j=0; j<128; j++) { 00068 display.rect(0,0,j,j,Black); 00069 display.update(); 00070 //wait(.01); 00071 display.rect(0,0,j,j,White); 00072 display.locate(5,6); 00073 display.printf("Sharp"); 00074 display.locate(1,8); 00075 display.printf("Draw Rectangle"); 00076 display.locate(4,10); 00077 display.printf("MemoryLCD"); 00078 display.update(); 00079 } 00080 for (int j=60; j>0; j--) { 00081 display.circle(64,64,j,Black); 00082 display.update(); 00083 wait(.01); 00084 display.circle(64,64,j,White); 00085 display.locate(5,6); 00086 display.printf("Sharp"); 00087 display.locate(2,8); 00088 display.printf("Draw Circle"); 00089 display.locate(4,10); 00090 display.printf("MemoryLCD"); 00091 display.update(); 00092 } 00093 } 00094 } 00095 * @endcode 00096 */ 00097 00098 00099 class SharpLCD { 00100 public: 00101 00102 SharpLCD(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName enable, PinName extcom); 00103 00104 /** 00105 * Sets external font usage, eg. dispaly.set_font(Arial12x12); 00106 * This uses pixel positioning. 00107 * display.set_font(NULL); returns to internal default font. 00108 * void set_font(const unsigned char * f); 00109 */ 00110 void set_font(const unsigned char * f); 00111 /** 00112 * Sets position of the next character or string print. 00113 * External font, set pixel x(column),y(row) position. 00114 * internal(default) font, set character column and row position 00115 */ 00116 void locate(int column, int row); 00117 /** 00118 * printf (from Stream). 00119 * Full printf funtionality only available with internal(default) font. 00120 * CR and LF have no effect with external fonts. 00121 */ 00122 void printf(const char* format, ...); 00123 /** 00124 * Enables (power) display. 00125 */ 00126 void enableDisplay(void); 00127 /** 00128 * Dissables (power) display. 00129 */ 00130 void disableDisplay(void); 00131 /** 00132 * Set a pixel in the pixel buffer. 00133 * 00134 * @param x X-Position. 00135 * @param y Y-Position. 00136 * @param colour Colour to set pixel to. White or Black. 00137 */ 00138 void pixel(int x, int y, int colour); 00139 /** 00140 * Draw rectangle one pixel wide (wire frame) in the pixel buffer. 00141 * 00142 * @param x0 X-Start position. 00143 * @param y0 Y-Start position. 00144 * @param x1 X-End position. 00145 * @param y1 Y-End position. 00146 * @param colour Colour to set pixel to. White or Black. 00147 */ 00148 void rect(int x0, int y0, int x1, int y1, int colour); 00149 /** 00150 * Draw filled rectangle in the pixel buffer. 00151 * 00152 * @param x0 X-Start position. 00153 * @param y0 Y-Start position. 00154 * @param w Width. 00155 * @param h Height. 00156 * @param colour Colour to fill rectangle to. White or Black. 00157 */ 00158 void fillrect(int x0, int y0, int w, int h, int colour); 00159 /** 00160 * Draw Circle one pixel wide in the pixel buffer. 00161 * 00162 * @param x0 X-Centre position. 00163 * @param y0 Y-Centre position. 00164 * @param r Radius. 00165 * @param colour Colour to set pixel to. White or Black. 00166 */ 00167 void circle(int x, int y, int r, int colour); 00168 /** 00169 * Draw Ellipse or Circle one pixel wide in the pixel buffer. 00170 * Set v & h to same values to produce a circle. 00171 00172 * @param xc X-Centre position. 00173 * @param yc Y-Centre position. 00174 * @param v Vertical radius. 00175 * @param h Horizontal radius. 00176 * @param colour Colour to set pixel to. White or Black. 00177 */ 00178 void ellipse(int xc, int yc, int v, int h, unsigned int colour); 00179 /** 00180 * Draw Filled Ellipse or Circle in the pixel buffer. 00181 * Set v & h to same values to produce a circle. 00182 00183 * @param xc X-Centre position. 00184 * @param yc Y-Centre position. 00185 * @param v Vertical radius. 00186 * @param h Horizontal radius. 00187 * @param colour Colour to set pixel to. White or Black. 00188 */ 00189 void fillellipse(int xc, int yc, int v, int h, unsigned int colour); 00190 /** 00191 * Draw a horizontal line one pixel wide in the pixel buffer. 00192 * 00193 * @param x0 X-Start position. 00194 * @param x1 X-End position. 00195 * @param y Y-position. 00196 * @param colour Colour to set pixel to. White or Black. 00197 */ 00198 void hline(int x0, int x1, int y, int colour); 00199 /** 00200 * Draw a Vertical line one pixel wide in the pixel buffer. 00201 * 00202 * @param x0 X-Position. 00203 * @param y0 Y-Start position. 00204 * @param y1 Y-End position. 00205 * @param colour Colour to set pixel to. White or Black. 00206 */ 00207 void vline(int x0, int y0, int y1, int colour); 00208 /** 00209 * Draw a Line in the pixel buffer. 00210 * 00211 * @param x0 X-Start position. 00212 * @param y0 Y-Start position. 00213 * @param x1 X-End position. 00214 * @param y1 Y-End position. 00215 * @param colour Colour to fill rectangle to. White or Black. 00216 */ 00217 void line(int x0, int y0, int x1, int y1, int colour); 00218 /** 00219 * Toggles the EXTCOM connection to the display if used. 00220 * Call this function at 55 ~ 65 Hz to keep the display from losing contrast. 00221 */ 00222 void toggle(); 00223 /** 00224 * Transfers pixel buffer to the display 00225 */ 00226 void update(); 00227 00228 /** 00229 * set pixel buffer to zero but do not blank screen. 00230 */ 00231 void clearspace(); 00232 00233 /** 00234 * Clear the display & set pixel buffer to zero. 00235 */ 00236 void clearImmediate(); 00237 /** 00238 * Move bitmap into pixel buffer 00239 * 00240 * @param bitmap pointer to uint8 array containing horizontal pixel data 00241 * @param bmpWidth width of the bitmap in pixels (must be byte multiple, eg 8,16,32,...) 00242 * @param bmpHeight height of the bitmap in pixels (must be byte multiple, eg 8,16,32,...) 00243 * @param startX starting position to apply bitmap in horizontal direction (0 = leftmost) (pixel resolution) 00244 * @param startY starting position to apply bitmap in vertical direction (0 = topmost) (pixel resolution) 00245 */ 00246 void showBMP(const uint8_t* bitmap, const uint32_t bmpWidth, const uint32_t bmpHeight, const uint32_t startX, const uint32_t startY); 00247 /** Output a character at the given position 00248 * 00249 * @param column column where charater must be written 00250 * @param row where character must be written 00251 * @param c the character to be written to the TextDisplay 00252 */ 00253 void character(int column, int row, int c); 00254 00255 /** return number if rows on TextDisplay 00256 * @result number of rows 00257 */ 00258 int rows(); 00259 /** return number if columns on TextDisplay 00260 * @result number of rows 00261 */ 00262 int columns(); 00263 00264 private: 00265 void window(int x, int y, int w, int h); 00266 void putp(int colour); 00267 void blit(int x, int y, int w, int h, const int *colour); 00268 void blitbit(int x, int y, int w, int h, const char* colour); 00269 protected: 00270 //SPI spi; // standard SPI 00271 BurstSPI spi; // Faster SPI pixel buffer transfer 00272 DigitalOut chipSelect; 00273 DigitalOut Enable; 00274 DigitalOut ExtCom; 00275 00276 uint8_t lcdPolarity; 00277 volatile uint32_t rowCount; 00278 uint8_t cmd[2 + (DISPLAY_WIDTH / DISPLAY_BUFFER_TYPE_SIZE * sizeof(DISPLAY_BUFFER_TYPE))]; 00279 volatile DISPLAY_BUFFER_TYPE pixelBuffer[DISPLAY_BUFFER_ELEMENTS]; // one full frame buffer 00280 volatile DISPLAY_BUFFER_TYPE RowState[DISPLAY_HEIGHT/DISPLAY_BUFFER_TYPE_SIZE]; // 1 bit per row to indicate row change status 00281 00282 // transfers pixel buffer to display 00283 void display_write(); 00284 00285 // pixel location 00286 short _x; 00287 short _y; 00288 00289 // window location 00290 short _x1; 00291 short _x2; 00292 short _y1; 00293 short _y2; 00294 00295 int _putc(int value); 00296 int _getc(); 00297 int width(); 00298 int height(); 00299 00300 // external font functions 00301 const unsigned char* font; 00302 int externalfont; 00303 00304 // character location 00305 int _column; 00306 int _row; 00307 unsigned int char_x; 00308 unsigned int char_y; 00309 00310 }; 00311 #endif
Generated on Wed Jul 13 2022 09:15:42 by
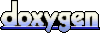