TSL2561 light sensor lib, tested with Multitech xDot-DK.
Fork of TSL2561 by
TSL2561.cpp
00001 00002 #include "TSL2561.h" 00003 #include "mbed.h" 00004 00005 Serial DEBUG(USBTX, USBRX); 00006 00007 #define DEBUG_PRINTX(z,x) if(z==1) DEBUG.printf(x); 00008 #define DEBUG_PRINTLNX(z,x) if(z==1) {DEBUG.printf(x); DEBUG.printf("\r\n");} 00009 #define DEBUG_PRINTXY(z,x, y) if(z==1) DEBUG.printf(x, y); 00010 #define DEBUG_PRINTLNXY(z,x, y) if(z==1) {DEBUG.printf(x, y); DEBUG.printf("\r\n");} 00011 00012 TSL2561::TSL2561():i2c(TSL2561_I2C_PINNAME_SDA,TSL2561_I2C_PINNAME_SCL){ 00013 i2c.frequency (300); 00014 _addr = TSL2561_ADDR_FLOAT; 00015 _initialized = false; 00016 _integration = TSL2561_INTEGRATIONTIME_13MS; 00017 _gain = TSL2561_GAIN_16X; 00018 } 00019 00020 TSL2561::TSL2561(uint8_t addr):i2c(TSL2561_I2C_PINNAME_SDA,TSL2561_I2C_PINNAME_SCL) { 00021 00022 _addr = addr; 00023 _initialized = false; 00024 _integration = TSL2561_INTEGRATIONTIME_13MS; 00025 _gain = TSL2561_GAIN_16X; 00026 // we cant do wire initialization till later, because we havent loaded Wire yet 00027 } 00028 00029 TSL2561::TSL2561(PinName sda, PinName scl):i2c(sda, scl) { 00030 00031 _addr = TSL2561_ADDR_FLOAT; 00032 _initialized = false; 00033 _integration = TSL2561_INTEGRATIONTIME_13MS; 00034 _gain = TSL2561_GAIN_16X; 00035 // we cant do wire initialization till later, because we havent loaded Wire yet 00036 } 00037 00038 TSL2561::TSL2561(PinName sda, PinName scl, uint8_t addr):i2c(sda, scl) { 00039 00040 _addr = addr; 00041 _initialized = false; 00042 _integration = TSL2561_INTEGRATIONTIME_13MS; 00043 _gain = TSL2561_GAIN_16X; 00044 // we cant do wire initialization till later, because we havent loaded Wire yet 00045 } 00046 00047 uint16_t TSL2561::getLuminosity (uint8_t channel) { 00048 00049 uint32_t x = getFullLuminosity(); 00050 00051 if (channel == 0) { 00052 // Reads two byte value from channel 0 (visible + infrared) 00053 00054 return (x & 0xFFFF); 00055 } else if (channel == 1) { 00056 // Reads two byte value from channel 1 (infrared) 00057 00058 return (x >> 16); 00059 } else if (channel == 2) { 00060 // Reads all and subtracts out just the visible! 00061 00062 return ( (x & 0xFFFF) - (x >> 16) ); 00063 } 00064 00065 // unknown channel! 00066 return 0; 00067 } 00068 00069 uint32_t TSL2561::getFullLuminosity (void) 00070 { 00071 if (!_initialized) begin(); 00072 00073 // Enable the device by setting the control bit to 0x03 00074 enable(); 00075 00076 // Wait x ms for ADC to complete 00077 switch (_integration) 00078 { 00079 case TSL2561_INTEGRATIONTIME_13MS: 00080 wait_ms(14); 00081 break; 00082 case TSL2561_INTEGRATIONTIME_101MS: 00083 wait_ms(102); 00084 break; 00085 default: 00086 wait_ms(403); 00087 break; 00088 } 00089 00090 //DEBUG_PRINTLNXY(0," Integration:= %d",_integration); 00091 00092 uint32_t x; 00093 x = read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN1_LOW); 00094 00095 // DEBUG_PRINTLNXY(0," x:= %d",x); 00096 00097 x <<= 16; 00098 x |= read16(TSL2561_COMMAND_BIT | TSL2561_WORD_BIT | TSL2561_REGISTER_CHAN0_LOW); 00099 00100 //DEBUG_PRINTLNXY(0," x:= %d",x); 00101 00102 //wait(3); 00103 disable(); 00104 00105 return x; 00106 } 00107 00108 00109 bool TSL2561::begin(void) { 00110 00111 char reg[1]; 00112 reg[0] = TSL2561_REGISTER_ID; 00113 char receivedata[1]; 00114 char read; 00115 i2c.write(_addr<<1, reg, 1); 00116 i2c.read(_addr<<1, receivedata, 1); 00117 read=receivedata[0]; 00118 00119 if (read & 0x0A ) { 00120 DEBUG_PRINTLNXY(0,"Read 0x%x => Found TSL2561",read); 00121 } else { 00122 return false; 00123 } 00124 _initialized = true; 00125 00126 // Set default integration time and gain 00127 setTiming(_integration); 00128 setGain(_gain); 00129 00130 // Note: by default, the device is in power down mode on bootup 00131 disable(); 00132 00133 return true; 00134 } 00135 00136 uint16_t TSL2561::read16(uint8_t reg) 00137 { 00138 uint16_t x; 00139 uint16_t t; 00140 char _x; 00141 char _t; 00142 char r[1]; 00143 r[0] = reg; 00144 char receivedata[2]; 00145 00146 i2c.write(_addr<<1, r, 1); 00147 i2c.read(_addr<<1, receivedata, 2); 00148 00149 _t=receivedata[0]; 00150 _x=receivedata[1]; 00151 00152 DEBUG_PRINTLNXY(0,"_t:=0x%x",_t); 00153 DEBUG_PRINTLNXY(0,"_x:=0x%x",_x); 00154 00155 t=(uint16_t)_t; 00156 x=(uint16_t)_x; 00157 x <<= 8; 00158 x |= t; 00159 00160 DEBUG_PRINTLNXY(0,"x:= %d",x); 00161 00162 return x; 00163 } 00164 00165 void TSL2561::write8 (uint8_t reg, uint8_t value) 00166 { 00167 i2c.start(); 00168 i2c.write(_addr<<1); 00169 i2c.write(reg); 00170 i2c.write(value); 00171 i2c.stop(); 00172 } 00173 00174 void TSL2561::setTiming(tsl2561IntegrationTime_t integration){ 00175 00176 if (!_initialized) begin(); 00177 00178 else DEBUG_PRINTLNX(0,"--------------Set Timing---------"); 00179 00180 enable(); 00181 00182 _integration = integration; 00183 00184 DEBUG_PRINTLNXY(0,"Integration: 0x%x",_integration); 00185 DEBUG_PRINTLNXY(0,"Gain: 0x%x",_gain); 00186 DEBUG_PRINTLNXY(0,"Integration | Gain: 0x%x",_integration | _gain); 00187 00188 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00189 00190 disable(); 00191 00192 DEBUG_PRINTLNX(0,"--------------Complete Set Timing-------------"); 00193 00194 //wait(1); 00195 00196 } 00197 00198 void TSL2561::setGain(tsl2561Gain_t gain) { 00199 00200 if (!_initialized) begin(); 00201 else DEBUG_PRINTLNX(0,"-------------Set Gain--------------"); 00202 00203 00204 enable(); 00205 00206 DEBUG_PRINTLNXY(0,"Intergration: 0x%x",_integration); 00207 DEBUG_PRINTLNXY(0,"Gain: 0x%x",_gain); 00208 DEBUG_PRINTLNXY(0,"Intergration | Gain: 0x%x",_integration | _gain); 00209 00210 _gain = gain; 00211 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _integration | _gain); 00212 //write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_TIMING, _gain); 00213 disable(); 00214 00215 DEBUG_PRINTLNX(0,"---------------Complete Set Gain----------------"); 00216 //wait(1); 00217 00218 } 00219 00220 void TSL2561::enable(void) 00221 { 00222 if (!_initialized) begin(); 00223 00224 // Enable the device by setting the control bit to 0x03 00225 DEBUG_PRINTLNX(0," Power On"); 00226 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWERON); 00227 } 00228 00229 void TSL2561::disable(void) 00230 { 00231 if (!_initialized) begin(); 00232 00233 // Disable the device by setting the control bit to 0x03 00234 DEBUG_PRINTLNX(0," Power Off"); 00235 write8(TSL2561_COMMAND_BIT | TSL2561_REGISTER_CONTROL, TSL2561_CONTROL_POWEROFF); 00236 }
Generated on Wed Jul 13 2022 15:41:39 by
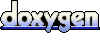