
Decode routine for weather station WT440H or WT450H
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 // This piece of software decode Weahter station temperature and humidity 00003 // freeware, by Lotfi BAGHLI, March, 2013 00004 // I hacked the TX WT440H, got 2 wires out (GND and DATA) 00005 // I still want to get the data from a RX one (WS738) or a simple 433 MHz RX but signal pbs not yet solved 00006 // 00007 // thanks to Jaakko Ala-Paavola, http://ala-paavola.fi/jaakko/doku.php?id=wt450h 00008 // for the protocol and decode routine 00009 // 00010 // Connect 2 wires to the MBED : GND and Data Signal to p18 00011 // Temperature and Humidity are displayed on the Serial via USB of the MBED 00012 00013 DigitalOut myled(LED1); 00014 DigitalOut led2(LED2); 00015 DigitalIn Rx433(p18); 00016 InterruptIn Rx433NotifyChange(p18); 00017 Serial pc(USBTX, USBRX); 00018 Timer T3; 00019 00020 #define TIMEOUT 1000 00021 #define BIT0_LENGTH 2000 00022 #define BIT1_LENGTH 1000 00023 #define VARIATION 500 00024 #define DATA_LENGTH 5 00025 #define SENSOR_COUNT 4 00026 #define NETWORK_COUNT 2 00027 #define MSGLENGTH 36 00028 00029 //#define DEBUG 00030 struct sensor { 00031 unsigned char humidity; 00032 signed char temp_int; 00033 unsigned char temp_dec; 00034 signed char min_int; 00035 unsigned char min_dec; 00036 signed char max_int; 00037 unsigned char max_dec; 00038 time_t timestamp; 00039 }; 00040 unsigned long T3saved_us; 00041 unsigned char bitcount = 0; 00042 unsigned char bytecount = 0; 00043 unsigned char second_half = 0; 00044 unsigned char data[DATA_LENGTH]; 00045 struct sensor measurement[NETWORK_COUNT][SENSOR_COUNT]; 00046 unsigned char minmaxday = 0; 00047 // make var global 00048 time_t t; 00049 unsigned char net, id; 00050 int t_int; 00051 unsigned char rh, t_dec; 00052 00053 unsigned int i,j, oldj; 00054 00055 void NCInterrupt() 00056 { 00057 unsigned char i; 00058 unsigned char bit; 00059 unsigned long current = T3.read_us(); 00060 unsigned long diff = current-T3saved_us; 00061 myled = ! myled; 00062 j++; 00063 T3saved_us=current; 00064 00065 00066 if ( diff < BIT0_LENGTH + VARIATION && diff > BIT0_LENGTH - VARIATION ) { 00067 bit = 0; 00068 second_half = 0; 00069 } else if ( diff < BIT1_LENGTH + VARIATION && diff > BIT1_LENGTH - VARIATION ) { 00070 if (second_half) { 00071 bit = 1; 00072 second_half = 0; 00073 } else { 00074 second_half = 1; 00075 return; 00076 } 00077 } else { 00078 goto reset; 00079 } 00080 00081 data[bitcount/8] = data[bitcount/8]<<1; 00082 data[bitcount/8] |= bit; 00083 bitcount++; 00084 00085 if ( bitcount == 4 ) { 00086 if ( data[0] != 0x0c ) 00087 goto reset; 00088 bitcount = 8; 00089 #ifdef DEBUG 00090 pc.print('#'); 00091 #endif 00092 } 00093 00094 if ( bitcount >= MSGLENGTH ) { 00095 00096 #ifdef DEBUG 00097 for (i=0; i<DATA_LENGTH; i++) { 00098 pc.print(data[i], HEX); 00099 pc.print(' '); 00100 } 00101 #endif 00102 // pc.print("Data: NET:"); 00103 net = 0x07 & (data[1]>>4)-1; 00104 // pc.print(net,DEC); 00105 // pc.print(" ID:"); 00106 id = 0x03 & (data[1]>>2); 00107 // pc.print(id, DEC); 00108 // pc.print(" RH:"); 00109 rh = data[2]; 00110 // pc.print(rh, DEC); 00111 // pc.print(" T:"); 00112 t_int = data[3]-50; 00113 // pc.print(t_int, DEC); 00114 t_dec = data[4]>>1; 00115 // pc.print('.'); 00116 // pc.print(t_dec,DEC); 00117 00118 t = time(NULL); 00119 00120 /* Do not store all sensors */ 00121 00122 if ( net <= NETWORK_COUNT ) { 00123 measurement[net][id].temp_int = t_int; 00124 measurement[net][id].temp_dec = t_dec; 00125 measurement[net][id].humidity = rh; 00126 /* 00127 if ( !measurement[net][id].timestamp || 00128 day(t) != minmaxday ) { 00129 minmaxday = day(t); 00130 measurement[net][id].max_int = t_int; 00131 measurement[net][id].max_dec = t_dec; 00132 measurement[net][id].min_int = t_int; 00133 measurement[net][id].min_dec = t_dec; 00134 } 00135 00136 if ( t_int > measurement[net][id].max_int || 00137 ( t_int == measurement[net][id].max_int && t_dec > measurement[net][id].max_dec ) ) { 00138 measurement[net][id].max_int = t_int; 00139 measurement[net][id].max_dec = t_dec; 00140 } 00141 00142 if ( t_int < measurement[net][id].min_int || 00143 ( t_int == measurement[net][id].min_int && t_dec < measurement[net][id].min_dec ) ) { 00144 measurement[net][id].min_int = t_int; 00145 measurement[net][id].min_dec = t_dec; 00146 } 00147 */ 00148 00149 measurement[net][id].timestamp = t; 00150 } 00151 00152 goto reset; 00153 } 00154 return; 00155 00156 reset: 00157 for (i=0; i<DATA_LENGTH; i++) 00158 data[i] = 0; 00159 00160 bytecount = 0; 00161 bitcount = 0; 00162 second_half = 0; 00163 return; 00164 } 00165 00166 int main() 00167 { 00168 j=0; 00169 T3.start(); 00170 pc.baud(115200); 00171 Rx433NotifyChange.mode(PullNone); 00172 Rx433NotifyChange.rise(&NCInterrupt); 00173 Rx433NotifyChange.fall(&NCInterrupt); 00174 while(1) { 00175 led2 = !led2; 00176 wait(1); 00177 if (oldj != j) 00178 { 00179 pc.printf("j:%d, Data: NET:%d ID:%d RH:%d T:%d.%d TIME:%s\r", j, net, id, rh, t_int, t_dec, ctime(&t)); 00180 oldj=j; 00181 } 00182 00183 } 00184 }
Generated on Wed Jul 27 2022 14:23:39 by
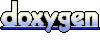