I2C device discovery and printout of known device names
Embed:
(wiki syntax)
Show/hide line numbers
i2cDevices.cpp
00001 #include "mbed.h" 00002 #include "i2cDevices.h" 00003 00004 extern RawSerial pc; 00005 00006 //--------------------------------------------------------------------------------------------------------------------------------------// 00007 //Constructor 00008 00009 i2c_discovery::i2c_discovery(PinName sda, PinName scl, const char* name) : _i2c(sda, scl) { 00010 _i2c.frequency(100000); 00011 } 00012 00013 //--------------------------------------------------------------------------------------------------------------------------------------// 00014 //Display detected i2c device name 00015 00016 int i2c_discovery::showI2C(i2cDeviceFlags& i2cFlags, char addr) { 00017 switch (addr) { 00018 #ifdef I2C_0x02 00019 case 0x02: 00020 pc.printf("%s\r\n", I2C_0x02); 00021 i2cFlags.PCA9955_GGG_ok = true; 00022 break; 00023 #endif 00024 #ifdef I2C_0x04 00025 case 0x04: 00026 pc.printf("%s\r\n", I2C_0x04); 00027 break; 00028 #endif 00029 #ifdef I2C_0x06 00030 case 0x06: 00031 pc.printf("%s\r\n", I2C_0x06); 00032 break; 00033 #endif 00034 #ifdef I2C_0x08 00035 case 0x08: 00036 pc.printf("%s\r\n", I2C_0x08); 00037 break; 00038 #endif 00039 #ifdef I2C_0x0A 00040 case 0x0A: 00041 pc.printf("%s\r\n", I2C_0x0A); 00042 break; 00043 #endif 00044 #ifdef I2C_0x0C 00045 case 0x0C: 00046 pc.printf("%s\r\n", I2C_0x0C); 00047 break; 00048 #endif 00049 #ifdef I2C_0x0E 00050 case 0x0E: 00051 pc.printf("%s\r\n", I2C_0x0E); 00052 break; 00053 #endif 00054 //---------------------------------------- 00055 #ifdef I2C_0x10 00056 case 0x10: 00057 pc.printf("%s\r\n", I2C_0x10); 00058 break; 00059 #endif 00060 #ifdef I2C_0x12 00061 case 0x12: 00062 pc.printf("%s\r\n", I2C_0x12); 00063 break; 00064 #endif 00065 #ifdef I2C_0x14 00066 case 0x14: 00067 pc.printf("%s\r\n", I2C_0x14); 00068 break; 00069 #endif 00070 #ifdef I2C_0x16 00071 case 0x16: 00072 pc.printf("%s\r\n", I2C_0x16); 00073 break; 00074 #endif 00075 #ifdef I2C_0x18 00076 case 0x18: 00077 pc.printf("%s\r\n", I2C_0x18); 00078 break; 00079 #endif 00080 #ifdef I2C_0x1A 00081 case 0x1A: 00082 pc.printf("%s\r\n", I2C_0x1A); 00083 break; 00084 #endif 00085 #ifdef I2C_0x1C 00086 case 0x1C: 00087 pc.printf("%s\r\n", I2C_0x1C); 00088 break; 00089 #endif 00090 #ifdef I2C_0x1E 00091 case 0x1E: 00092 pc.printf("%s\r\n", I2C_0x1E); 00093 break; 00094 #endif 00095 //---------------------------------------- 00096 #ifdef I2C_0x20 00097 case 0x20: 00098 pc.printf("%s\r\n", I2C_0x20); 00099 i2cFlags.VEML60xx_ok = true; 00100 break; 00101 #endif 00102 #ifdef I2C_0x22 00103 case 0x22: 00104 pc.printf("%s\r\n", I2C_0x22); 00105 break; 00106 #endif 00107 #ifdef I2C_0x24 00108 case 0x24: 00109 pc.printf("%s\r\n", I2C_0x24); 00110 break; 00111 #endif 00112 #ifdef I2C_0x26 00113 case 0x26: 00114 pc.printf("%s\r\n", I2C_0x26); 00115 break; 00116 #endif 00117 #ifdef I2C_0x28 00118 case 0x28: 00119 pc.printf("%s\r\n", I2C_0x28); 00120 break; 00121 #endif 00122 #ifdef I2C_0x2A 00123 case 0x2A: 00124 pc.printf("%s\r\n", I2C_0x2A); 00125 break; 00126 #endif 00127 #ifdef I2C_0x2C 00128 case 0x2C: 00129 pc.printf("%s\r\n", I2C_0x2C); 00130 break; 00131 #endif 00132 #ifdef I2C_0x2E 00133 case 0x2E: 00134 pc.printf("%s\r\n", I2C_0x2E); 00135 break; 00136 #endif 00137 //---------------------------------------- 00138 #ifdef I2C_0x30 00139 case 0x30: 00140 pc.printf("%s\r\n", I2C_0x30); 00141 break; 00142 #endif 00143 #ifdef I2C_0x32 00144 case 0x32: 00145 pc.printf("%s\r\n", I2C_0x32); 00146 break; 00147 #endif 00148 #ifdef I2C_0x34 00149 case 0x34: 00150 pc.printf("%s\r\n", I2C_0x34); 00151 break; 00152 #endif 00153 #ifdef I2C_0x36 00154 case 0x36: 00155 pc.printf("%s\r\n", I2C_0x36); 00156 break; 00157 #endif 00158 #ifdef I2C_0x38 00159 case 0x38: 00160 pc.printf("%s\r\n", I2C_0x38); 00161 i2cFlags.mma8451cs1_ok = true; 00162 //fxos8700_ok = true; 00163 break; 00164 #endif 00165 #ifdef I2C_0x3A 00166 case 0x3A: 00167 pc.printf("%s\r\n", I2C_0x3A); 00168 //fxos8700_ok = true; 00169 break; 00170 #endif 00171 #ifdef I2C_0x3C 00172 case 0x3C: 00173 pc.printf("%s\r\n", I2C_0x3C); 00174 //fxos8700_ok = true; 00175 break; 00176 #endif 00177 #ifdef I2C_0x3E 00178 case 0x3E: 00179 pc.printf("%s\r\n", I2C_0x3E); 00180 //fxos8700_ok = true; 00181 break; 00182 #endif 00183 //---------------------------------------- 00184 #ifdef I2C_0x40 00185 case 0x40: 00186 pc.printf("%s\r\n", I2C_0x40); 00187 break; 00188 #endif 00189 #ifdef I2C_0x42 00190 case 0x42: 00191 pc.printf("%s\r\n", I2C_0x42); 00192 break; 00193 #endif 00194 #ifdef I2C_0x44 00195 case 0x44: 00196 pc.printf("%s\r\n", I2C_0x44); 00197 break; 00198 #endif 00199 #ifdef I2C_0x46 00200 case 0x46: 00201 pc.printf("%s\r\n", I2C_0x46); 00202 break; 00203 #endif 00204 #ifdef I2C_0x48 00205 case 0x48: 00206 pc.printf("%s\r\n", I2C_0x48); 00207 break; 00208 #endif 00209 #ifdef I2C_0x4A 00210 case 0x4A: 00211 pc.printf("%s\r\n", I2C_0x4A); 00212 break; 00213 #endif 00214 #ifdef I2C_0x4C 00215 case 0x4C: 00216 pc.printf("%s\r\n", I2C_0x4C); 00217 break; 00218 #endif 00219 #ifdef I2C_0x4E 00220 case 0x4E: 00221 pc.printf("%s\r\n", I2C_0x4E); 00222 break; 00223 #endif 00224 //---------------------------------------- 00225 #ifdef I2C_0x50 00226 case 0x50: 00227 pc.printf("%s\r\n", I2C_0x50); 00228 break; 00229 #endif 00230 #ifdef I2C_0x52 00231 case 0x52: 00232 pc.printf("%s\r\n", I2C_0x52); 00233 break; 00234 #endif 00235 #ifdef I2C_0x54 00236 case 0x54: 00237 pc.printf("%s\r\n", I2C_0x54); 00238 break; 00239 #endif 00240 #ifdef I2C_0x56 00241 case 0x56: 00242 pc.printf("%s\r\n", I2C_0x56); 00243 break; 00244 #endif 00245 #ifdef I2C_0x58 00246 case 0x58: 00247 pc.printf("%s\r\n", I2C_0x58); 00248 break; 00249 #endif 00250 #ifdef I2C_0x5A 00251 case 0x5A: 00252 pc.printf("%s\r\n", I2C_0x5A); 00253 break; 00254 #endif 00255 #ifdef I2C_0x5C 00256 case 0x5C: 00257 pc.printf("%s\r\n", I2C_0x5C); 00258 break; 00259 #endif 00260 #ifdef I2C_0x5E 00261 case 0x5E: 00262 pc.printf("%s\r\n", I2C_0x5E); 00263 break; 00264 #endif 00265 //---------------------------------------- 00266 #ifdef I2C_0x60 00267 case 0x60: 00268 pc.printf("%s\r\n", I2C_0x60); 00269 break; 00270 #endif 00271 #ifdef I2C_0x62 00272 case 0x62: 00273 pc.printf("%s\r\n", I2C_0x62); 00274 break; 00275 #endif 00276 #ifdef I2C_0x64 00277 case 0x64: 00278 pc.printf("%s\r\n", I2C_0x64); 00279 break; 00280 #endif 00281 #ifdef I2C_0x66 00282 case 0x66: 00283 pc.printf("%s\r\n", I2C_0x66); 00284 break; 00285 #endif 00286 #ifdef I2C_0x68 00287 case 0x68: 00288 pc.printf("%s\r\n", I2C_0x68); 00289 break; 00290 #endif 00291 #ifdef I2C_0x6A 00292 case 0x6A: 00293 pc.printf("%s\r\n", I2C_0x6A); 00294 break; 00295 #endif 00296 #ifdef I2C_0x6C 00297 case 0x6C: 00298 pc.printf("%s\r\n", I2C_0x6C); 00299 break; 00300 #endif 00301 #ifdef I2C_0x6E 00302 case 0x6E: 00303 pc.printf("%s\r\n", I2C_0x6E); 00304 break; 00305 #endif 00306 //---------------------------------------- 00307 #ifdef I2C_0x70 00308 case 0x70: 00309 pc.printf("%s\r\n", I2C_0x70); 00310 i2cFlags.VEML6070_ok = true; 00311 break; 00312 #endif 00313 #ifdef I2C_0x72 00314 case 0x72: 00315 pc.printf("%s\r\n", I2C_0x72); 00316 break; 00317 #endif 00318 #ifdef I2C_0x74 00319 case 0x74: 00320 pc.printf("%s\r\n", I2C_0x74); 00321 break; 00322 #endif 00323 #ifdef I2C_0x76 00324 case 0x76: 00325 pc.printf("%s\r\n", I2C_0x76); 00326 break; 00327 #endif 00328 #ifdef I2C_0x78 00329 case 0x78: 00330 pc.printf("%s\r\n", I2C_0x78); 00331 break; 00332 #endif 00333 #ifdef I2C_0x7A 00334 case 0x7A: 00335 pc.printf("%s\r\n", I2C_0x7A); 00336 break; 00337 #endif 00338 #ifdef I2C_0x7C 00339 case 0x7C: 00340 pc.printf("%s\r\n", I2C_0x7C); 00341 break; 00342 #endif 00343 #ifdef I2C_0x7E 00344 case 0x7E: 00345 pc.printf("%s\r\n", I2C_0x7E); 00346 break; 00347 #endif 00348 //---------------------------------------- 00349 #ifdef I2C_0x80 00350 case 0x80: 00351 pc.printf("%s\r\n", I2C_0x80); 00352 i2cFlags.HTU21D_ok = true; 00353 i2cFlags.INA219_MAX7300_gg_ok = true; 00354 i2cFlags.INA230_MAX7300_gg_ok = true; 00355 break; 00356 #endif 00357 #ifdef I2C_0x82 00358 case 0x82: 00359 pc.printf("%s\r\n", I2C_0x82); 00360 break; 00361 #endif 00362 #ifdef I2C_0x84 00363 case 0x84: 00364 pc.printf("%s\r\n", I2C_0x84); 00365 break; 00366 #endif 00367 #ifdef I2C_0x86 00368 case 0x86: 00369 pc.printf("%s\r\n", I2C_0x86); 00370 break; 00371 #endif 00372 #ifdef I2C_0x88 00373 case 0x88: 00374 pc.printf("%s\r\n", I2C_0x88); 00375 break; 00376 #endif 00377 #ifdef I2C_0x8A 00378 case 0x8A: 00379 pc.printf("%s\r\n", I2C_0x8A); 00380 break; 00381 #endif 00382 #ifdef I2C_0x8C 00383 case 0x8C: 00384 pc.printf("%s\r\n", I2C_0x8C); 00385 break; 00386 #endif 00387 #ifdef I2C_0x8E 00388 case 0x8E: 00389 pc.printf("%s\r\n", I2C_0x8E); 00390 break; 00391 #endif 00392 //---------------------------------------- 00393 #ifdef I2C_0x90 00394 case 0x90: 00395 pc.printf("%s\r\n", I2C_0x90); 00396 break; 00397 #endif 00398 #ifdef I2C_0x92 00399 case 0x92: 00400 pc.printf("%s\r\n", I2C_0x92); 00401 break; 00402 #endif 00403 #ifdef I2C_0x94 00404 case 0x94: 00405 pc.printf("%s\r\n", I2C_0x94); 00406 break; 00407 #endif 00408 #ifdef I2C_0x96 00409 case 0x96: 00410 pc.printf("%s\r\n", I2C_0x96); 00411 break; 00412 #endif 00413 #ifdef I2C_0x98 00414 case 0x98: 00415 pc.printf("%s\r\n", I2C_0x98); 00416 break; 00417 #endif 00418 #ifdef I2C_0x9A 00419 case 0x9A: 00420 pc.printf("%s\r\n", I2C_0x9A); 00421 break; 00422 #endif 00423 #ifdef I2C_0x9C 00424 case 0x9C: 00425 pc.printf("%s\r\n", I2C_0x9C); 00426 break; 00427 #endif 00428 #ifdef I2C_0x9E 00429 case 0x9E: 00430 pc.printf("%s\r\n", I2C_0x9E); 00431 break; 00432 #endif 00433 //---------------------------------------- 00434 #ifdef I2C_0xA0 00435 case 0xA0: 00436 pc.printf("%s\r\n", I2C_0xA0); 00437 i2cFlags.eeprom000_ok = true; 00438 break; 00439 #endif 00440 #ifdef I2C_0xA2 00441 case 0xA2: 00442 pc.printf("%s\r\n", I2C_0xA2); 00443 break; 00444 #endif 00445 #ifdef I2C_0xA4 00446 case 0xA4: 00447 pc.printf("%s\r\n", I2C_0xA4); 00448 break; 00449 #endif 00450 #ifdef I2C_0xA6 00451 case 0xA6: 00452 pc.printf("%s\r\n", I2C_0xA6); 00453 break; 00454 #endif 00455 #ifdef I2C_0xA8 00456 case 0xA8: 00457 pc.printf("%s\r\n", I2C_0xA8); 00458 break; 00459 #endif 00460 #ifdef I2C_0xAA 00461 case 0xAA: 00462 pc.printf("%s\r\n", I2C_0xAA); 00463 i2cFlags.bq27210_ok = true; 00464 break; 00465 #endif 00466 #ifdef I2C_0xAC 00467 case 0xAC: 00468 pc.printf("%s\r\n", I2C_0xAC); 00469 break; 00470 #endif 00471 #ifdef I2C_0xAE 00472 case 0xAE: 00473 pc.printf("%s\r\n", I2C_0xAE); 00474 break; 00475 #endif 00476 //---------------------------------------- 00477 #ifdef I2C_0xB0 00478 case 0xB0: 00479 pc.printf("%s\r\n", I2C_0xB0); 00480 break; 00481 #endif 00482 #ifdef I2C_0xB2 00483 case 0xB2: 00484 pc.printf("%s\r\n", I2C_0xB2); 00485 break; 00486 #endif 00487 #ifdef I2C_0xB4 00488 case 0xB4: 00489 pc.printf("%s\r\n", I2C_0xB4); 00490 i2cFlags.ccs811_0_ok = true; 00491 break; 00492 #endif 00493 #ifdef I2C_0xB6 00494 case 0xB6: 00495 pc.printf("%s\r\n", I2C_0xB6); 00496 i2cFlags.ccs811_1_ok = true; 00497 break; 00498 #endif 00499 #ifdef I2C_0xB8 00500 case 0xB8: 00501 pc.printf("%s\r\n", I2C_0xB8); 00502 break; 00503 #endif 00504 #ifdef I2C_0xBA 00505 case 0xBA: 00506 pc.printf("%s\r\n", I2C_0xBA); 00507 break; 00508 #endif 00509 #ifdef I2C_0xBC 00510 case 0xBC: 00511 pc.printf("%s\r\n", I2C_0xBC); 00512 break; 00513 #endif 00514 #ifdef I2C_0xBE 00515 case 0xBE: 00516 pc.printf("%s\r\n", I2C_0xBE); 00517 break; 00518 #endif 00519 //---------------------------------------- 00520 #ifdef I2C_0xC0 00521 case 0xC0: 00522 pc.printf("%s\r\n", I2C_0xC0); 00523 i2cFlags.mlx90620_ok = true; 00524 //i2cFlags.PCA9955_GGG_ok = true; 00525 break; 00526 #endif 00527 #ifdef I2C_0xC2 00528 case 0xC2: 00529 pc.printf("%s\r\n", I2C_0xC2); 00530 i2cFlags.SCD30_ok = true; 00531 break; 00532 #endif 00533 #ifdef I2C_0xC4 00534 case 0xC4: 00535 pc.printf("%s\r\n", I2C_0xC4); 00536 break; 00537 #endif 00538 #ifdef I2C_0xC6 00539 case 0xC6: 00540 pc.printf("%s\r\n", I2C_0xC6); 00541 break; 00542 #endif 00543 #ifdef I2C_0xC8 00544 case 0xC8: 00545 pc.printf("%s\r\n", I2C_0xC8); 00546 break; 00547 #endif 00548 #ifdef I2C_0xCA 00549 case 0xCA: 00550 pc.printf("%s\r\n", I2C_0xCA); 00551 break; 00552 #endif 00553 #ifdef I2C_0xCC 00554 case 0xCC: 00555 pc.printf("%s\r\n", I2C_0xCC); 00556 break; 00557 #endif 00558 #ifdef I2C_0xCE 00559 case 0xCE: 00560 pc.printf("%s\r\n", I2C_0xCE); 00561 break; 00562 #endif 00563 //---------------------------------------- 00564 #ifdef I2C_0xD0 00565 case 0xD0: 00566 pc.printf("%s\r\n", I2C_0xD0); 00567 i2cFlags.rtcDS_ok = true; 00568 break; 00569 #endif 00570 #ifdef I2C_0xD2 00571 case 0xD2: 00572 pc.printf("%s\r\n", I2C_0xD2); 00573 break; 00574 #endif 00575 #ifdef I2C_0xD4 00576 case 0xD4: 00577 pc.printf("%s\r\n", I2C_0xD4); 00578 break; 00579 #endif 00580 #ifdef I2C_0xD6 00581 case 0xD6: 00582 pc.printf("%s\r\n", I2C_0xD6); 00583 break; 00584 #endif 00585 #ifdef I2C_0xD8 00586 case 0xD8: 00587 pc.printf("%s\r\n", I2C_0xD8); 00588 break; 00589 #endif 00590 #ifdef I2C_0xDA 00591 case 0xDA: 00592 pc.printf("%s\r\n", I2C_0xDA); 00593 break; 00594 #endif 00595 #ifdef I2C_0xDC 00596 case 0xDC: 00597 pc.printf("%s\r\n", I2C_0xDC); 00598 break; 00599 #endif 00600 #ifdef I2C_0xDE 00601 case 0xDE: 00602 pc.printf("%s\r\n", I2C_0xDE); 00603 i2cFlags.mcp7941x_ok = true; 00604 break; 00605 #endif 00606 //---------------------------------------- 00607 #ifdef I2C_0xE0 00608 case 0xE0: 00609 pc.printf("%s\r\n", I2C_0xE0); 00610 break; 00611 #endif 00612 #ifdef I2C_0xE2 00613 case 0xE2: 00614 pc.printf("%s\r\n", I2C_0xE2); 00615 break; 00616 #endif 00617 #ifdef I2C_0xE4 00618 case 0xE4: 00619 pc.printf("%s\r\n", I2C_0xE4); 00620 break; 00621 #endif 00622 #ifdef I2C_0xE6 00623 case 0xE6: 00624 pc.printf("%s\r\n", I2C_0xE6); 00625 break; 00626 #endif 00627 #ifdef I2C_0xE8 00628 case 0xE8: 00629 pc.printf("%s\r\n", I2C_0xE8); 00630 break; 00631 #endif 00632 #ifdef I2C_0xEA 00633 case 0xEA: 00634 pc.printf("%s\r\n", I2C_0xEA); 00635 break; 00636 #endif 00637 #ifdef I2C_0xEC 00638 case 0xEC: 00639 pc.printf("%s\r\n", I2C_0xEC); 00640 i2cFlags.bme280_cs0_ok = true; 00641 break; 00642 #endif 00643 #ifdef I2C_0xEE 00644 case 0xEE: 00645 pc.printf("%s\r\n", I2C_0xEE); 00646 i2cFlags.ms5611_ok = true; 00647 i2cFlags.bme280_cs1_ok = true; 00648 break; 00649 #endif 00650 //---------------------------------------- 00651 #ifdef I2C_0xF0 00652 case 0xF0: 00653 pc.printf("%s\r\n", I2C_0xF0); 00654 break; 00655 #endif 00656 #ifdef I2C_0xF2 00657 case 0xF2: 00658 pc.printf("%s\r\n", I2C_0xF2); 00659 break; 00660 #endif 00661 #ifdef I2C_0xF4 00662 case 0xF4: 00663 pc.printf("%s\r\n", I2C_0xF4); 00664 break; 00665 #endif 00666 #ifdef I2C_0xF6 00667 case 0xF6: 00668 pc.printf("%s\r\n", I2C_0xF6); 00669 break; 00670 #endif 00671 #ifdef I2C_0xF8 00672 case 0xF8: 00673 pc.printf("%s\r\n", I2C_0xF8); 00674 break; 00675 #endif 00676 #ifdef I2C_0xFA 00677 case 0xFA: 00678 pc.printf("%s\r\n", I2C_0xFA); 00679 break; 00680 #endif 00681 #ifdef I2C_0xFC 00682 case 0xFC: 00683 pc.printf("%s\r\n", I2C_0xFC); 00684 break; 00685 #endif 00686 #ifdef I2C_0xFE 00687 case 0xFE: 00688 pc.printf("%s\r\n", I2C_0xFE); 00689 break; 00690 #endif 00691 //---------------------------------------- 00692 default: 00693 pc.printf("%sunknown%s\r\n", DOMAGENTA, DONONE); 00694 break; 00695 } 00696 return(0); 00697 } 00698 00699 //------------------------------------------------------------------------------ 00700 // Clear I2C device flags 00701 00702 void i2c_discovery::clearFlags(i2cDeviceFlags& i2cFlags) { 00703 i2cFlags.rtcDS_ok = false; 00704 i2cFlags.ms5611_ok = false; 00705 i2cFlags.bme280_cs0_ok = false; 00706 i2cFlags.bme280_cs1_ok = false; 00707 i2cFlags.mma8451cs1_ok = false; 00708 i2cFlags.HTU21D_ok = false; 00709 i2cFlags.PCA9955_GGG_ok = false; 00710 i2cFlags.VEML60xx_ok = false; 00711 i2cFlags.VEML6070_ok = false; 00712 i2cFlags.eeprom000_ok = false; 00713 i2cFlags.INA219_MAX7300_gg_ok = false; 00714 i2cFlags.INA230_MAX7300_gg_ok = false; 00715 i2cFlags.bq27210_ok = false; 00716 i2cFlags.SCD30_ok = false; 00717 i2cFlags.ccs811_0_ok = false; 00718 i2cFlags.ccs811_1_ok = false; 00719 i2cFlags.mcp7941x_ok = false; 00720 } 00721 00722 //------------------------------------------------------------------------------ 00723 // Detect I2C device chain. If i2c chain > 1, return illegal number 255. Else, 00724 // return the total number of i2c devices detected 00725 00726 int i2c_discovery::dispI2C(i2cDeviceFlags& i2cFlags) { 00727 //i2c_discovery::clearFlags(i2cFlags); 00728 int count = 0; 00729 for (int address = 2; address < 256; address +=2) { 00730 char xBuf[2] = { 0, 0 }; 00731 int x2c = _i2c.write(address, xBuf, 1, false); 00732 if(x2c == 0) { 00733 pc.printf(" - i2c device found at address 0x%02X %s", address, DOBLUE); 00734 showI2C(i2cFlags, address); 00735 pc.printf("%s", DONONE); 00736 count++; 00737 } 00738 } 00739 return(count); 00740 } 00741 00742 //------------------------------------------------------------------------------ 00743 // Detect I2C device chain 00744 00745 int i2c_discovery::find_i2c(i2cDeviceFlags& i2cFlags) { 00746 pc.printf("Searching for devices on i2c bus...\n"); 00747 int count = dispI2C(i2cFlags); 00748 pc.printf(" - %s%2d%s device(s) found on i2c bus\n", DOBLUE, count, DONONE); 00749 return(count); 00750 } 00751
Generated on Sun Jul 17 2022 00:58:49 by
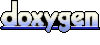