Watchdog Timer for the K64F. User can set timeout value from 1 to 356 seconds with a one second resolution.
Dependents: Telliskivi2_2014 mbed-IBooth-ETH TwitterReader
Watchdog.h
00001 #ifndef WATCHDOG_K64F_H 00002 #define WATCHDOG_K64F_H 00003 00004 #include "mbed.h" 00005 00006 /** Routines to set and kick the Watchdog timer for the K64F. 00007 * 00008 * User inputs a value from 1 to 356 seconds when initializing the Watchdog 00009 * timer. The user's input number is multiplied by a fixed value and then 00010 * placed into the countdown timer. 00011 * 00012 * The user is responsible for "kicking" the Watchdog before the timeout interval 00013 * expires, otherwise the K64F will automatically reboot. 00014 * 00015 * @code 00016 * #include "mbed.h" 00017 * #include "Watchdog.h" 00018 * 00019 * Watchdog wdt; 00020 * 00021 * int main() { 00022 * //initialization code.... 00023 * wdt.kick(20); //init the watchdog for a 20 second timeout 00024 * while(1) { 00025 * wait_ms(1000); //do some code 00026 * wdt.kick(); //kick the watchdog before 20 seconds is up 00027 * } 00028 * } 00029 * @endcode 00030 * 00031 */ 00032 /* Watchdog controller class 00033 */ 00034 class Watchdog { 00035 00036 public: 00037 /** Create a Watchdog object and initialize timeout in seconds 00038 * 00039 * @param int timeout in seconds. Range (1 - 356) 00040 * 00041 * Note: if value out of range, default value with be 356 00042 * 00043 * @return NONE 00044 */ 00045 void kick(int WDTseconds); 00046 00047 /** Keep alive by kicking the Watchdog occasionally 00048 * 00049 * @param NONE 00050 * 00051 * @return NONE 00052 */ 00053 void kick(); 00054 00055 private: 00056 /** Disable the watchdog Function 00057 * 00058 * @param NONE 00059 * 00060 * @return NONE 00061 */ 00062 void DisableWDOG(); 00063 00064 /** Enable the watchdog Function 00065 * 00066 * @param NONE 00067 * 00068 * @return NONE 00069 */ 00070 void EnableWDOG(); 00071 00072 }; 00073 00074 #endif
Generated on Wed Jul 13 2022 01:14:15 by
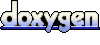