最新revisionだとなんかerrorになるので、暫定的に rev 111にrevert。ごめんなさい。こういうときどういうふうにcommitすればいいのか分からなかったので。
Fork of BLE_API by
BLEDevice Class Reference
The base class used to abstract away BLE capable radio transceivers or SOCs, to enable this BLE API to work with any radio transparently. More...
#include <BLEDevice.h>
Public Member Functions | |
ble_error_t | init () |
Initialize the BLE controller. | |
ble_error_t | setAddress (Gap::addr_type_t type, const uint8_t address[6]) |
Set the BTLE MAC address and type. | |
void | setAdvertisingType (GapAdvertisingParams::AdvertisingType) |
void | setAdvertisingInterval (uint16_t interval) |
void | setAdvertisingTimeout (uint16_t timeout) |
void | setAdvertisingParams (const GapAdvertisingParams &advParams) |
Please refer to the APIs above. | |
ble_error_t | setAdvertisingPayload (void) |
This API is typically used as an internal helper to udpate the transport backend with advertising data before starting to advertise. | |
void | clearAdvertisingPayload (void) |
Reset any advertising payload prepared from prior calls to accumulateAdvertisingPayload(). | |
ble_error_t | accumulateAdvertisingPayload (uint8_t flags) |
Accumulate an AD structure in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayload (GapAdvertisingData::Appearance app) |
Accumulate an AD structure in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayloadTxPower (int8_t power) |
Accumulate an AD structure in the advertising payload. | |
ble_error_t | accumulateAdvertisingPayload (GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) |
Accumulate a variable length byte-stream as an AD structure in the advertising payload. | |
ble_error_t | accumulateScanResponse (GapAdvertisingData::DataType type, const uint8_t *data, uint8_t len) |
Accumulate a variable length byte-stream as an AD structure in the scanResponse payload. | |
ble_error_t | startAdvertising (void) |
Start advertising (GAP Discoverable, Connectable modes, Broadcast Procedure). | |
ble_error_t | stopAdvertising (void) |
Stop advertising (GAP Discoverable, Connectable modes, Broadcast Procedure). | |
void | onDisconnection (Gap::HandleSpecificEventCallback_t disconnectionCallback) |
Used to setup a callback for GAP disconnection. | |
void | onDataSent (GattServer::ServerEventCallback_t callback) |
Setup a callback for the GATT event DATA_SENT. | |
void | onDataWritten (GattServer::EventCallback_t callback) |
Setup a callback for when a characteristic has its value updated by a client. | |
ble_error_t | addService (GattService &service) |
Add a service declaration to the local server ATT table. | |
void | waitForEvent (void) |
Yield control to the BLE stack or to other tasks waiting for events. | |
const char * | getVersion (void) |
This call allows the application to get the BLE stack version information. | |
ble_error_t | setDeviceName (const uint8_t *deviceName) |
Set the device name characteristic in the GAP service. | |
ble_error_t | getDeviceName (uint8_t *deviceName, unsigned *lengthP) |
Get the value of the device name characteristic in the GAP service. | |
ble_error_t | setAppearance (uint16_t appearance) |
Set the appearance characteristic in the GAP service. | |
ble_error_t | getAppearance (uint16_t *appearanceP) |
Set the appearance characteristic in the GAP service. | |
ble_error_t | setTxPower (int8_t txPower) |
Set the radio's transmit power. | |
ble_error_t | setAdvertisingData (const GapAdvertisingData &ADStructures, const GapAdvertisingData &scanResponse) |
DEPRECATED. |
Detailed Description
The base class used to abstract away BLE capable radio transceivers or SOCs, to enable this BLE API to work with any radio transparently.
Definition at line 30 of file BLEDevice.h.
Member Function Documentation
ble_error_t accumulateAdvertisingPayload | ( | uint8_t | flags ) |
Accumulate an AD structure in the advertising payload.
Please note that the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used as an additional 31 bytes if the advertising payload proves to be too small.
- Parameters:
-
flags The flags to be added. Multiple flags may be specified in combination.
Definition at line 386 of file BLEDevice.h.
ble_error_t accumulateAdvertisingPayload | ( | GapAdvertisingData::Appearance | app ) |
Accumulate an AD structure in the advertising payload.
Please note that the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used as an additional 31 bytes if the advertising payload proves to be too small.
- Parameters:
-
app The appearance of the peripheral.
Definition at line 393 of file BLEDevice.h.
ble_error_t accumulateAdvertisingPayload | ( | GapAdvertisingData::DataType | type, |
const uint8_t * | data, | ||
uint8_t | len | ||
) |
Accumulate a variable length byte-stream as an AD structure in the advertising payload.
Please note that the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used as an additional 31 bytes if the advertising payload proves to be too small.
- Parameters:
-
type The type which describes the variable length data. data data bytes. len length of data.
Definition at line 407 of file BLEDevice.h.
ble_error_t accumulateAdvertisingPayloadTxPower | ( | int8_t | power ) |
Accumulate an AD structure in the advertising payload.
Please note that the payload is limited to 31 bytes. The SCAN_RESPONSE message may be used as an additional 31 bytes if the advertising payload proves to be too small.
- Parameters:
-
app The max transmit power to be used by the controller. This is only a hint.
Definition at line 400 of file BLEDevice.h.
ble_error_t accumulateScanResponse | ( | GapAdvertisingData::DataType | type, |
const uint8_t * | data, | ||
uint8_t | len | ||
) |
Accumulate a variable length byte-stream as an AD structure in the scanResponse payload.
- Parameters:
-
type The type which describes the variable length data. data data bytes. len length of data.
Definition at line 414 of file BLEDevice.h.
ble_error_t addService | ( | GattService & | service ) |
Add a service declaration to the local server ATT table.
Also add the characteristics contained within.
Definition at line 500 of file BLEDevice.h.
void clearAdvertisingPayload | ( | void | ) |
Reset any advertising payload prepared from prior calls to accumulateAdvertisingPayload().
Definition at line 379 of file BLEDevice.h.
ble_error_t getAppearance | ( | uint16_t * | appearanceP ) |
Set the appearance characteristic in the GAP service.
- Parameters:
-
[out] appearance The new value for the device-appearance.
Definition at line 570 of file BLEDevice.h.
ble_error_t getDeviceName | ( | uint8_t * | deviceName, |
unsigned * | lengthP | ||
) |
Get the value of the device name characteristic in the GAP service.
- Parameters:
-
[out] deviceName Pointer to an empty buffer where the UTF-8 *non NULL- terminated* string will be placed. Set this value to NULL in order to obtain the deviceName-length from the 'length' parameter. in/out] lengthP (on input) Length of the buffer pointed to by deviceName; (on output) the complete device name length (without the null terminator).
- Note:
- If the device name is longer than the size of the supplied buffer, length will return the complete device name length, and not the number of bytes actually returned in deviceName. The application may use this information to retry with a suitable buffer size.
Sample use: uint8_t deviceName[20]; unsigned length = sizeof(deviceName); ble.getDeviceName(deviceName, &length); if (length < sizeof(deviceName)) { deviceName[length] = 0; } DEBUG("length: %u, deviceName: %s\r\n", length, deviceName);
Definition at line 558 of file BLEDevice.h.
const char * getVersion | ( | void | ) |
This call allows the application to get the BLE stack version information.
- Returns:
- A pointer to a const string representing the version. Note: The string is owned by the BLE_API.
Definition at line 546 of file BLEDevice.h.
ble_error_t init | ( | void | ) |
Initialize the BLE controller.
This should be called before using anything else in the BLE_API.
Definition at line 337 of file BLEDevice.h.
void onDataSent | ( | GattServer::ServerEventCallback_t | callback ) |
Setup a callback for the GATT event DATA_SENT.
Definition at line 470 of file BLEDevice.h.
void onDataWritten | ( | GattServer::EventCallback_t | callback ) |
Setup a callback for when a characteristic has its value updated by a client.
Definition at line 476 of file BLEDevice.h.
void onDisconnection | ( | Gap::HandleSpecificEventCallback_t | disconnectionCallback ) |
Used to setup a callback for GAP disconnection.
Definition at line 464 of file BLEDevice.h.
ble_error_t setAddress | ( | Gap::addr_type_t | type, |
const uint8_t | address[6] | ||
) |
ble_error_t setAdvertisingData | ( | const GapAdvertisingData & | ADStructures, |
const GapAdvertisingData & | scanResponse | ||
) |
DEPRECATED.
Definition at line 586 of file BLEDevice.h.
void setAdvertisingInterval | ( | uint16_t | interval ) |
- Parameters:
-
[in] interval Advertising interval between 0x0020 and 0x4000 in 0.625ms units (20ms to 10.24s). If using non-connectable mode (ADV_NON_CONNECTABLE_UNDIRECTED) this min value is 0x00A0 (100ms). To reduce the likelihood of collisions, the link layer perturbs this interval by a pseudo-random delay with a range of 0 ms to 10 ms for each advertising event.
- Decreasing this value will allow central devices to detect your peripheral faster at the expense of more power being used by the radio due to the higher data transmit rate.
- This field must be set to 0 if connectionMode is equal to ADV_CONNECTABLE_DIRECTED
- See Bluetooth Core Specification, Vol 3., Part C, Appendix A for suggested advertising intervals.
Definition at line 361 of file BLEDevice.h.
void setAdvertisingParams | ( | const GapAdvertisingParams & | advParams ) |
Please refer to the APIs above.
Definition at line 373 of file BLEDevice.h.
ble_error_t setAdvertisingPayload | ( | void | ) |
This API is typically used as an internal helper to udpate the transport backend with advertising data before starting to advertise.
It may also be explicity used to dynamically reset the accumulated advertising payload and scanResponse; to do this, the application can clear and re- accumulate a new advertising payload (and scanResponse) before using this API.
Definition at line 421 of file BLEDevice.h.
void setAdvertisingTimeout | ( | uint16_t | timeout ) |
- Parameters:
-
[in] timeout Advertising timeout between 0x1 and 0x3FFF (1 and 16383) in seconds. Enter 0 to disable the advertising timeout.
Definition at line 367 of file BLEDevice.h.
void setAdvertisingType | ( | GapAdvertisingParams::AdvertisingType | advType ) |
- Parameters:
-
[in] advType The GAP advertising mode to use for this device. Valid values are defined in AdvertisingType:
- ADV_NON_CONNECTABLE_UNDIRECTED
- All connections to the peripheral device will be refused.
- ADV_CONNECTABLE_DIRECTED
- Only connections from a pre-defined central device will be accepted.
- ADV_CONNECTABLE_UNDIRECTED
- Any central device can connect to this peripheral.
- ADV_SCANNABLE_UNDIRECTED
- Any central device can connect to this peripheral, and the secondary Scan Response payload will be included or available to central devices.
- See Bluetooth Core Specification 4.0 (Vol. 3), Part C, Section 9.3 and Core Specification 4.0 (Vol. 6), Part B, Section 2.3.1 for further information on GAP connection modes
Definition at line 355 of file BLEDevice.h.
ble_error_t setAppearance | ( | uint16_t | appearance ) |
Set the appearance characteristic in the GAP service.
- Parameters:
-
[in] appearance The new value for the device-appearance.
Definition at line 564 of file BLEDevice.h.
ble_error_t setDeviceName | ( | const uint8_t * | deviceName ) |
Set the device name characteristic in the GAP service.
- Parameters:
-
deviceName The new value for the device-name. This is a UTF-8 encoded, NULL-terminated string.
Definition at line 552 of file BLEDevice.h.
ble_error_t setTxPower | ( | int8_t | txPower ) |
Set the radio's transmit power.
- Parameters:
-
[in] txPower Radio transmit power in dBm.
Definition at line 576 of file BLEDevice.h.
ble_error_t startAdvertising | ( | void | ) |
Start advertising (GAP Discoverable, Connectable modes, Broadcast Procedure).
Definition at line 427 of file BLEDevice.h.
ble_error_t stopAdvertising | ( | void | ) |
Stop advertising (GAP Discoverable, Connectable modes, Broadcast Procedure).
Definition at line 440 of file BLEDevice.h.
void waitForEvent | ( | void | ) |
Yield control to the BLE stack or to other tasks waiting for events.
This is a sleep function which will return when there is an application specific interrupt, but the MCU might wake up several times before returning (to service the stack). This is not always interchangeable with WFE().
Definition at line 523 of file BLEDevice.h.
Generated on Tue Jul 12 2022 20:47:07 by
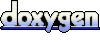