
Game for Project 2
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed wave_player
robot.cpp
00001 #include "mbed.h" 00002 #include "globals.h" 00003 #include "map_public.h " 00004 #include "robot.h" 00005 00006 // Following code will only draw the Robot on the screen. You are expected to modify most of the functions here. 00007 // All other necessary functions will be implemented by you. Ex: the movement of Pacman, calculate the score ... etc. 00008 00009 void robot_init(int grid_x, int grid_y){ 00010 00011 map_eat_candy(grid_x,grid_y); //clear the cookie on the grid. 00012 robot_draw(grid_x,grid_y); 00013 00014 } 00015 00016 void robot_draw(int grid_x, int grid_y){ 00017 00018 GRID grid_info = map_get_grid_status(grid_x,grid_y); 00019 // Calculate the actual position of the grid 00020 int frog_x = grid_info.x + GRID_RADIUS; 00021 int frog_y = grid_info.y + GRID_RADIUS; 00022 00023 // MAKE 00024 uLCD.filled_circle(frog_x, frog_y, 3, 0x909090); 00025 uLCD.filled_rectangle(frog_x-3,frog_y+1,frog_x+3,frog_y+3,0x33FF66); 00026 uLCD.line(frog_x-1, frog_y+4,frog_x-1, frog_y+5, 0xFF0000);//legs 00027 uLCD.line(frog_x+2, frog_y+4,frog_x+2, frog_y+5, 0xFF0000); 00028 uLCD.line(frog_x+4, frog_y+1,frog_x+5, frog_y+1, 0xFF0000);//hands 00029 uLCD.line(frog_x-4, frog_y+1,frog_x-5, frog_y+1, 0xFF0000); 00030 } 00031 00032 void robot_clear(int grid_x, int grid_y){ 00033 00034 GRID grid_info = map_get_grid_status(grid_x,grid_y); 00035 //Fill the grid (a rectangle) with BACKGROUND_COLOR to clear the pacman 00036 uLCD.filled_rectangle(grid_info.x, grid_info.y, grid_info.x+GRID_SIZE-1, grid_info.y+GRID_SIZE-1, BACKGROUND_COLOR); 00037 00038 }
Generated on Tue Aug 2 2022 11:28:12 by
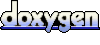