TCP Server that handles multiple client requests at the same time by using multiple threads
Dependents: ThreadServer Server_Multi_Client
Server.cpp
00001 /* 00002 ** File name: Server.cpp 00003 ** Descriptions: TCP server that handles multiple client requests in separate threads 00004 ** 00005 **------------------------------------------------------------------------------------------------------ 00006 ** Created by: Ivan Shindev 00007 ** Created date: 06/11/2013 00008 ** Version: 1.0 00009 ** Descriptions: The original version 00010 ** 00011 **------------------------------------------------------------------------------------------------------ 00012 ** Modified by: Ivan Shindev 00013 ** Modified date: 06/29/2013 00014 ** Version: 00015 ** Descriptions: Added functionality of running multiple thread instances of the same function 00016 ********************************************************************************************************/ 00017 #include "Server.h" 00018 00019 #include "mbed.h" 00020 #include "Threads.h" 00021 /*Handle_client handles the client request 00022 * 00023 * Default: Echo the clients request 00024 */ 00025 00026 void Handle_client(void const *socket_data) { 00027 00028 int socket; 00029 char buffer[256]; 00030 socket = (int)socket_data; 00031 00032 int n = lwip_recv(socket ,buffer,sizeof(buffer),0); //read from the client 00033 00034 if (lwip_send(socket ,buffer,n,0)!=n) //send the content back to the socket 00035 { 00036 00037 00038 } 00039 00040 00041 } 00042 00043 00044 Server::Server(int port, int max_number_of_clients): 00045 _port(port), _max_number_of_clients(max_number_of_clients){ 00046 00047 00048 } 00049 00050 int Server::Start() { 00051 00052 EthernetInterface eth; 00053 eth.init(); //Use DHCP 00054 eth.connect(); 00055 00056 int socket_server; 00057 struct sockaddr_in localHost; 00058 memset(&localHost, 0, sizeof(localHost)); 00059 int new_socket; 00060 00061 if( (socket_server= lwip_socket(AF_INET, SOCK_STREAM, 0))<0) 00062 { 00063 return -1; 00064 } 00065 localHost.sin_family = AF_INET; 00066 localHost.sin_port = htons(_port); //port 00067 localHost.sin_addr.s_addr = INADDR_ANY; //localhost address 00068 00069 if (lwip_bind(socket_server, (const struct sockaddr *) &localHost, sizeof(localHost)) < 0) { 00070 00071 return -1; 00072 } 00073 if (lwip_listen(socket_server,3)<0) 00074 { 00075 00076 perror("listen"); 00077 exit(EXIT_FAILURE); 00078 } 00079 00080 socklen_t newSockRemoteHostLen = sizeof(localHost); 00081 00082 ThreadList* my_threads=NULL; //List of all Initialized threads 00083 ThreadList* thread; //pointer to the last created ThreadList element 00084 int max=_max_number_of_clients; 00085 while(1) 00086 { 00087 new_socket = lwip_accept(socket_server, (struct sockaddr*) &localHost, &newSockRemoteHostLen); 00088 if (new_socket < 0) 00089 { 00090 00091 } 00092 else 00093 { 00094 00095 if(initThread(&my_threads,Handle_client,&thread,max)==0) 00096 { 00097 lwip_close(new_socket); 00098 00099 } 00100 else 00101 { 00102 00103 thread->id=osThreadCreate(thread->thread,(void *) new_socket); 00104 00105 } 00106 } 00107 00108 00109 } 00110 } 00111 00112
Generated on Fri Jul 15 2022 21:23:43 by
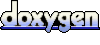