Implementation of the WifiPlusClick hardware module.
Dependents: WifiPlusKlickExample
UDPSocket Class Reference
Class UDPSocket, inherits from class Socket and implements the functionality of a UDP Socket when using a WifiPlusClick module. More...
#include <UDPSocket.h>
Inherits Socket.
Public Member Functions | |
UDPSocket () | |
UDPSocket constructor to instantiate a UDPSocket object. | |
int | init (void) |
Function init provides initialization of the UDPSocket object. | |
int | bind (unsigned int port=0) |
Function bind will bind a UDPSocket to a local port. | |
int | join_multicast_group (const char *address) |
Function join_multicast_group. | |
int | set_broadcasting (bool broadcast=true) |
Function set_broadcasting. | |
int | sendTo (Endpoint &remote, char *packet, int length) |
Function sendTo implements sending of a packet to a specific endpoint. | |
int | receiveFrom (Endpoint &remote, char *packet, int length) |
Function receiveFrom implements receiving a packet of data on a socket. | |
void | set_blocking (bool blocking, unsigned int timeout=1500) |
Function set_blocking sets the socket into a blocking or non-blocking status. | |
int | set_option (int level, int optname, const void *optval, int socklen) |
Function set_option is not working as expected, because there this functionality is not supported by the WifiPlusClick module. | |
int | close (bool shutdown=true) |
Function close will close the socket. |
Detailed Description
Class UDPSocket, inherits from class Socket and implements the functionality of a UDP Socket when using a WifiPlusClick module.
- Note:
- Please note that the functionality provided by a WifiPlusClick module is limited. Neither broadcasting nor multicasting functionality is possible.
Definition at line 27 of file UDPSocket.h.
Constructor & Destructor Documentation
UDPSocket | ( | ) |
UDPSocket constructor to instantiate a UDPSocket object.
Definition at line 23 of file UDPSocket.cpp.
Member Function Documentation
int bind | ( | unsigned int | port = 0 ) |
Function bind will bind a UDPSocket to a local port.
The socket object should not already have been used for other purposes before.
- Note:
- Please note that there is currently no check implemented as to wheather or not the socket has already been used before. port : The port to which to bind the UDPSocket to. If the port is specified as zero, a non-zero value beginning with 1024 will be selected.
- Returns:
- : 0 if successfull, or -1 otherwise.
Definition at line 37 of file UDPSocket.cpp.
int close | ( | bool | shutdown = true ) |
[inherited] |
Function close will close the socket.
- Parameters:
-
shutdown : This parameter will actually be ignored by the implementation.
- Returns:
- : -1 if not successfull >=0 if successfull
Definition at line 38 of file Socket.cpp.
int init | ( | void | ) |
Function init provides initialization of the UDPSocket object.
- Returns:
- : true if successfull, or false otherwise.
Definition at line 27 of file UDPSocket.cpp.
int join_multicast_group | ( | const char * | address ) |
Function join_multicast_group.
- Note:
- This function is not implemented as the WifiPlusClick module does not support this functionality.
int receiveFrom | ( | Endpoint & | remote, |
char * | packet, | ||
int | length | ||
) |
Function receiveFrom implements receiving a packet of data on a socket.
The remote address will be provided on return. Make sure to use it on an initialized or bound socket.
- Parameters:
-
remote : a reference to an endpoint, which will receive the remote ip-address and the remote port from where data was returned. packet : a data buffer which will receive the received data. The buffer must be at least as larget as length bytes. length : The maximum number of bytes to receive. Please make sure that the buffer packet is large enough to hold this data completely.
- Returns:
- : the number of databytes actually received or -1 on failure.
- Note:
- there may be more data received than what the buffer can store. Any data that does not fit in the buffer is discarded !
Definition at line 62 of file UDPSocket.cpp.
int sendTo | ( | Endpoint & | remote, |
char * | packet, | ||
int | length | ||
) |
Function sendTo implements sending of a packet to a specific endpoint.
Make sure to use it on an initialized or bound socket.
- Parameters:
-
remote : a reference to a valid endpoint specifying the remote ip-address and the remote port where to send the packet. packet : a pointer to a valid buffer containing the packet data to send. length : Specifies the number of data bytes to send.
- Returns:
- : the number of databytes actually sent or -1 on failure.
Definition at line 52 of file UDPSocket.cpp.
void set_blocking | ( | bool | blocking, |
unsigned int | timeout = 1500 |
||
) | [inherited] |
Function set_blocking sets the socket into a blocking or non-blocking status.
In case of a blocking socket a timeout can be specified.
- Parameters:
-
blocking : set to true if time consuming socket operations shall block execution until they are finished or until a timeout occurs. If set to false, socket operations will terminated immediately. timeout : a timeout value in milliseconds for use in blocking operations.
Definition at line 31 of file Socket.cpp.
int set_broadcasting | ( | bool | broadcast = true ) |
Function set_broadcasting.
- Note:
- This fnction is not implemented as the WifiPlusClick module does not support this functionality.
int set_option | ( | int | level, |
int | optname, | ||
const void * | optval, | ||
int | socklen | ||
) | [inherited] |
Function set_option is not working as expected, because there this functionality is not supported by the WifiPlusClick module.
- Note:
- This functionality is just kept for compatibility reasons.
Generated on Tue Jul 12 2022 23:18:35 by
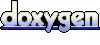