Implementation of the WifiPlusClick hardware module.
Dependents: WifiPlusKlickExample
TCPSocketConnection Class Reference
Class TCPSocketConnection inherits from Socket and Endpoint. More...
#include <TCPSocketConnection.h>
Inherits Socket, and Endpoint.
Public Member Functions | |
TCPSocketConnection () | |
Public constructor to instantiate an object of class TCPSocketConnection. | |
int | connect (const char *host, const int port) |
Function connect allows to create an unused socket object to a remote host on a remote port. | |
bool | is_connected (void) |
Function to query wheather the socket is already connected with a remote instance. | |
int | send (char *data, int length) |
Function send allows to send data to an already connected TCP socket. | |
int | send_all (char *data, int length) |
Function send_all allows to send data to an already connected TCP socket. | |
int | recv (char *data, int length) |
Function recv allows to receive data from an already connected TCP socket. | |
int | recv_all (char *data, int length) |
Function recv_all allows to receive data entirely from an already connected TCP socket. | |
void | set_blocking (bool blocking, unsigned int timeout=1500) |
Function set_blocking sets the socket into a blocking or non-blocking status. | |
int | set_option (int level, int optname, const void *optval, int socklen) |
Function set_option is not working as expected, because there this functionality is not supported by the WifiPlusClick module. | |
int | close (bool shutdown=true) |
Function close will close the socket. | |
void | reset_address (void) |
Reset the address of this endpoint. | |
int | set_address (const char *host, const int port) |
Set the address of this endpoint. | |
char * | get_address (void) |
Get the IP address of this endpoint. | |
int | get_port (void) |
Get the port of this endpoint. | |
Friends | |
class | TCPSocketServer |
Detailed Description
Class TCPSocketConnection inherits from Socket and Endpoint.
Implements the client TCP socket functionality.
Definition at line 26 of file TCPSocketConnection.h.
Constructor & Destructor Documentation
Public constructor to instantiate an object of class TCPSocketConnection.
Definition at line 21 of file TCPSocketConnection.cpp.
Member Function Documentation
int close | ( | bool | shutdown = true ) |
[inherited] |
Function close will close the socket.
- Parameters:
-
shutdown : This parameter will actually be ignored by the implementation.
- Returns:
- : -1 if not successfull >=0 if successfull
Definition at line 38 of file Socket.cpp.
int connect | ( | const char * | host, |
const int | port | ||
) |
Function connect allows to create an unused socket object to a remote host on a remote port.
- Parameters:
-
host : remote host to which to initiate a TCP connection. port : remote port to which to initiate a TCP connection.
- Returns:
- : 0 if successfull or -1 on failure.
Definition at line 26 of file TCPSocketConnection.cpp.
char * get_address | ( | void | ) | [inherited] |
Get the IP address of this endpoint.
- Returns:
- The IP address of this endpoint.
Definition at line 45 of file Endpoint.cpp.
int get_port | ( | void | ) | [inherited] |
Get the port of this endpoint.
- Returns:
- The port of this endpoint
Definition at line 49 of file Endpoint.cpp.
bool is_connected | ( | void | ) |
Function to query wheather the socket is already connected with a remote instance.
- Returns:
- : true if already connected or false otherwise.
Definition at line 44 of file TCPSocketConnection.cpp.
int recv | ( | char * | data, |
int | length | ||
) |
Function recv allows to receive data from an already connected TCP socket.
Please note that this function will return once a first bunch of data has been received. It will not wait until all the requested data is available.
- Parameters:
-
data : pointer to a valid data buffer which will receive the received data. The buffer must be big enough to hold all the requested data. length : Maximum numnber of bytes to receive.
- Returns:
- : number of bytes actually received and stored in data buffer or -1 on failure.
Definition at line 84 of file TCPSocketConnection.cpp.
int recv_all | ( | char * | data, |
int | length | ||
) |
Function recv_all allows to receive data entirely from an already connected TCP socket.
Please note that this function will only return if all the requested data was received or a timeout occured.
- Parameters:
-
data : pointer to a valid data buffer which will receive the received data. The buffer must be big enough to hold all the requested data. length : Number of bytes to receive.
- Returns:
- : number of bytes actually received and stored in data buffer or -1 on failure.
Definition at line 94 of file TCPSocketConnection.cpp.
void reset_address | ( | void | ) | [inherited] |
Reset the address of this endpoint.
Definition at line 33 of file Endpoint.cpp.
int send | ( | char * | data, |
int | length | ||
) |
Function send allows to send data to an already connected TCP socket.
- Parameters:
-
data : pointer to a valid data buffer. length : Number of bytes from data buffer to send.
- Returns:
- : number of bytes actually sent or -1 on failure.
Definition at line 49 of file TCPSocketConnection.cpp.
int send_all | ( | char * | data, |
int | length | ||
) |
Function send_all allows to send data to an already connected TCP socket.
The function will try to send all the data specified by length. If a timeout occurs in a blocking configuration the function returns the number of bytes actually sent.
- Parameters:
-
data : pointer to a valid data buffer. length : Number of bytes from data buffer to send.
- Returns:
- : number of bytes actually send or -1 on failure.
Definition at line 59 of file TCPSocketConnection.cpp.
int set_address | ( | const char * | host, |
const int | port | ||
) | [inherited] |
Set the address of this endpoint.
- Parameters:
-
host The endpoint address (it can either be an IP Address or a hostname that will be resolved with DNS). port The endpoint port
- Returns:
- 0 on success, -1 on failure (when an hostname cannot be resolved by DNS).
Definition at line 38 of file Endpoint.cpp.
void set_blocking | ( | bool | blocking, |
unsigned int | timeout = 1500 |
||
) | [inherited] |
Function set_blocking sets the socket into a blocking or non-blocking status.
In case of a blocking socket a timeout can be specified.
- Parameters:
-
blocking : set to true if time consuming socket operations shall block execution until they are finished or until a timeout occurs. If set to false, socket operations will terminated immediately. timeout : a timeout value in milliseconds for use in blocking operations.
Definition at line 31 of file Socket.cpp.
int set_option | ( | int | level, |
int | optname, | ||
const void * | optval, | ||
int | socklen | ||
) | [inherited] |
Function set_option is not working as expected, because there this functionality is not supported by the WifiPlusClick module.
- Note:
- This functionality is just kept for compatibility reasons.
Generated on Tue Jul 12 2022 23:18:35 by
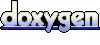