
test1
Dependencies: BLE_API mbed nRF51822
Fork of I2C_Demo by
wire.h
00001 00002 /* 00003 00004 Copyright (c) 2014 RedBearLab, All rights reserved. 00005 00006 This library is free software; you can redistribute it and/or 00007 modify it under the terms of the GNU Lesser General Public 00008 License as published by the Free Software Foundation; either 00009 version 2.1 of the License, or (at your option) any later version. 00010 00011 This library is distributed in the hope that it will be useful, 00012 but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. 00014 See the GNU Lesser General Public License for more details. 00015 00016 You should have received a copy of the GNU Lesser General Public 00017 License along with this library; if not, write to the Free Software 00018 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00019 00020 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00021 EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00022 MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00023 IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY 00024 CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, 00025 TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE 00026 SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00027 00028 */ 00029 00030 #ifndef _WIRE_H_ 00031 #define _WIRE_H_ 00032 00033 #include "mbed.h" 00034 00035 #define TWI_DELAY(x) wait_us(x); 00036 00037 #define BUFF_MAX_LENGTH 128 00038 00039 #define MAX_TIMEOUT_LOOPS (20000UL) 00040 00041 #define TWI_FREQUENCY_100K 0 00042 #define TWI_FREQUENCY_250K 1 00043 #define TWI_FREQUENCY_400K 2 00044 00045 #define TWI_SCL 28 00046 #define TWI_SDA 29 00047 00048 00049 class TwoWire 00050 { 00051 public : 00052 TwoWire(NRF_TWI_Type *twi_use); 00053 void begin(); 00054 void begin(uint32_t scl_pin, uint32_t sda_pin, uint8_t speed); 00055 void beginTransmission(uint8_t); 00056 void beginTransmission(int); 00057 uint8_t endTransmission(void); 00058 uint8_t endTransmission(uint8_t); 00059 uint8_t requestFrom(uint8_t, uint8_t); 00060 uint8_t requestFrom(uint8_t, uint8_t, uint8_t); 00061 uint8_t requestFrom(int, int); 00062 uint8_t requestFrom(int, int, int); 00063 int write(uint8_t); 00064 int write(const uint8_t *, size_t); 00065 int available(void); 00066 int read(void); 00067 00068 private : 00069 uint8_t RX_Buffer[BUFF_MAX_LENGTH]; 00070 uint8_t RX_BufferIndex; 00071 uint8_t RX_BufferLength; 00072 00073 uint8_t TX_Buffer[BUFF_MAX_LENGTH]; 00074 uint8_t TX_BufferIndex; 00075 uint8_t TX_BufferLength; 00076 00077 NRF_TWI_Type *twi; 00078 00079 uint8_t PPI_channel; 00080 uint8_t Transform_Addr; 00081 00082 uint32_t SDA_Pin; 00083 uint32_t SCL_Pin; 00084 00085 uint32_t twi_frequency; 00086 00087 enum TwoWireStatus { 00088 UNINITIALIZED, 00089 MASTER_IDLE, 00090 MASTER_SEND, 00091 MASTER_RECV, 00092 SLAVE_IDLE, 00093 SLAVE_RECV, 00094 SLAVE_SEND 00095 }; 00096 TwoWireStatus twi_status; 00097 00098 bool twi_master_clear_bus(void); 00099 bool twi_master_init(void); 00100 uint8_t twi_master_read(uint8_t *data, uint8_t data_length, uint8_t issue_stop_condition); 00101 uint8_t twi_master_write(uint8_t *data, uint8_t data_length, uint8_t issue_stop_condition); 00102 }; 00103 00104 #endif
Generated on Thu Jul 28 2022 06:39:21 by
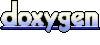