
11u24 Eeprom utility.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 00002 00003 #include "mbed.h" 00004 #include "IAP.h" 00005 #include "TextLCD.h" 00006 #include "stdlib.h" 00007 #include <stdio.h> 00008 00009 #define FLOAT_SIZE 4 00010 #define TARGET_ADDRESS 64 00011 #define NEXT_PLACE 1 00012 #define PLACE 0 00013 00014 IAP iap; 00015 float getEepromFloat(int place); 00016 void teletype(char* text, int row); 00017 00018 typedef char * string; 00019 00020 // ====== Pins ========= 00021 00022 DigitalOut gLED(P1_18); 00023 DigitalOut rLED(P1_24); 00024 00025 00026 TextLCD lcd(P1_22, P1_14, P0_9, P0_8, P1_21, P1_2); // rs, e, d4-d7 00027 00028 00029 //Tie unused analogue pins LOW 00030 00031 DigitalOut a1(P0_15); 00032 DigitalOut a2(P0_16); 00033 DigitalOut a3(P0_22); 00034 DigitalOut a4(P0_23); 00035 00036 // ====== Variables ========= 00037 00038 float test_float = 12.5; 00039 float display_float = 0.0; 00040 00041 int twodp =0; 00042 00043 float _ON; 00044 float _OFF; 00045 00046 float calib; 00047 float voltage; 00048 float displayVoltage; 00049 float displaySet; 00050 00051 float difference = 0; 00052 float calibrate=0.0; 00053 00054 00055 00056 00057 // ====== CODE BEGINS========= 00058 00059 typedef union _data { 00060 float f; 00061 char s[4]; 00062 } myData; 00063 00064 00065 float getEepromFloat(int place) 00066 { 00067 myData returnedFloat; 00068 char someBytes[4]; 00069 00070 iap.read_eeprom( (char*)(TARGET_ADDRESS+(place*4)), someBytes, FLOAT_SIZE ); 00071 00072 returnedFloat.s[0] = someBytes[0]; 00073 returnedFloat.s[1] = someBytes[1]; 00074 returnedFloat.s[2] = someBytes[2]; 00075 returnedFloat.s[3] = someBytes[3]; 00076 return returnedFloat.f; 00077 00078 } 00079 00080 void setEepromFloat(int place, float incomingFloat) 00081 { 00082 char theBytes[4]; 00083 myData aFloat; 00084 00085 aFloat.f = incomingFloat; 00086 00087 theBytes[0] = aFloat.s[0]; 00088 theBytes[1] = aFloat.s[1]; 00089 theBytes[2] = aFloat.s[2]; 00090 theBytes[3] = aFloat.s[3]; 00091 00092 iap.write_eeprom( theBytes, (char*) (TARGET_ADDRESS+(place*4)), FLOAT_SIZE ); 00093 00094 } 00095 00096 00097 int main() 00098 { 00099 00100 teletype("Hello", 0); 00101 float aValue=0.0; 00102 float theReturned=99.99; 00103 00104 while (1) { 00105 wait(2.0); 00106 00107 aValue++; // increment it 00108 00109 setEepromFloat(PLACE,aValue); //put it in place 0 (Global variable) 00110 00111 theReturned = getEepromFloat(PLACE); //get it back 00112 00113 lcd.cls(); 00114 teletype("Value: %f", theReturned); //output it to the screen 00115 00116 00117 }; 00118 } 00119 00120 00121 00122 00123 char* left(string Source,int NewLen) 00124 { 00125 00126 char* result; 00127 char* temp; 00128 if (NewLen <=0) 00129 NewLen =1; /* Minimum length */ 00130 00131 result = (char*)malloc(NewLen + 1); /* +1 = DFTTZ! */ 00132 *result=' '; /* default for unassigned strings */ 00133 temp=result; 00134 if (Source && *Source) { /* don't copy an empty string */ 00135 while (NewLen-- >0) 00136 *temp++=*Source++; 00137 } else temp++; 00138 *temp='\0'; 00139 return result; 00140 } 00141 00142 void teletype(char* text, int row) 00143 { 00144 lcd.locate(0,row); 00145 lcd.printf(" "); 00146 00147 int whole=strlen(text); 00148 00149 for (int i=1; i<=whole; i++) { 00150 lcd.locate(0,row); 00151 lcd.printf(left(text,i)); 00152 wait(0.15); 00153 } 00154 } 00155 00156
Generated on Thu Jul 14 2022 03:49:24 by
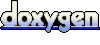