
Utility class for MIDI over Bluetooth LE
Dependencies: BLE_API mbed nRF51822
BLEMIDI Class Reference
A class to communicate a BLE MIDI device. More...
#include <BLEMIDI.h>
Public Member Functions | |
BLEMIDI (BLEDevice *device) | |
Constructor. | |
BLEMIDI (BLEDevice *dev, char *deviceName) | |
Constructor with device name. | |
bool | connected () |
Check if a BLE MIDI device is connected. | |
void | attachTuneRequest (void(*fn)()) |
Attach a callback called when the `Tune Request` event is received. | |
void | attachTimingClock (void(*fn)()) |
Attach a callback called when the `Timing Clock` event is received. | |
void | attachStart (void(*fn)()) |
Attach a callback called when the `Start` event is received. | |
void | attachContinue (void(*fn)()) |
Attach a callback called when the `Continue` event is received. | |
void | attachStop (void(*fn)()) |
Attach a callback called when the `Stop` event is received. | |
void | attachActiveSensing (void(*fn)()) |
Attach a callback called when the `Active Sensing` event is received. | |
void | attachReset (void(*fn)()) |
Attach a callback called when the `Reset` event is received. | |
void | attachnProgramChange (void(*fn)(uint8_t, uint8_t)) |
Attach a callback called when the `Program Change` event is received. | |
void | attachChannelAftertouch (void(*fn)(uint8_t, uint8_t)) |
Attach a callback called when the `Channel Aftertouch` event is received. | |
void | attachTimeCodeQuarterFrame (void(*fn)(uint8_t)) |
Attach a callback called when the `Time Code Quarter Frame` event is received. | |
void | attachSongSelect (void(*fn)(uint8_t)) |
Attach a callback called when the `Song Select` event is received. | |
void | attachNoteOff (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when the `Note Off` event is received. | |
void | attachNoteOn (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when the `Note On` event is received. | |
void | attachPolyphonicAftertouch (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when the `Polyphonic Aftertouch` event is received. | |
void | attachControlChange (void(*fn)(uint8_t, uint8_t, uint8_t)) |
Attach a callback called when the `Control Change` event is received. | |
void | attachPitchWheel (void(*fn)(uint8_t, uint16_t)) |
Attach a callback called when the `Pitch Wheel` event is received. | |
void | attachSongPositionPointer (void(*fn)(uint16_t)) |
Attach a callback called when the `Song Position Pointer` event is received. | |
void | attachSystemExclusive (void(*fn)(uint8_t *, uint16_t, bool)) |
Attach a callback called when the `System Exclusive` event is received. | |
void | sendTuneRequest () |
Send a `Tune Request` event. | |
void | sendTimingClock () |
Send a `Timing Clock` event. | |
void | sendStart () |
Send a `Start` event. | |
void | sendContinue () |
Send a `Continue` event. | |
void | sendStop () |
Send a `Stop` event. | |
void | sendActiveSensing () |
Send a `Active Sensing` event. | |
void | sendReset () |
Send a `Reset` event. | |
void | sendProgramChange (uint8_t channel, uint8_t program) |
Send a `Program Change` event. | |
void | sendChannelAftertouch (uint8_t channel, uint8_t pressure) |
Send a `Channel Aftertouch` event. | |
void | sendTimeCodeQuarterFrame (uint8_t timing) |
Send a `Time Code Quarter Frame` event. | |
void | sendSongSelect (uint8_t song) |
Send a `Song Select` event. | |
void | sendNoteOff (uint8_t channel, uint8_t note, uint8_t velocity) |
Send a `Note Off` event. | |
void | sendNoteOn (uint8_t channel, uint8_t note, uint8_t velocity) |
Send a `Note On` event. | |
void | sendPolyphonicAftertouch (uint8_t channel, uint8_t note, uint8_t pressure) |
Send a `Polyphonic Aftertouch` event. | |
void | sendControlChange (uint8_t channel, uint8_t function, uint8_t value) |
Send a `Control Change` event. | |
void | sendPitchWheel (uint8_t channel, uint16_t amount) |
Send a `Pitch Wheel` event. | |
void | sendSongPositionPointer (uint16_t position) |
Send a `Song Position Pointer` event. | |
void | sendSystemExclusive (uint8_t *sysex, uint16_t length) |
Send a `System Exclusive` event. | |
void | onBleDisconnection (Gap::Handle_t handle, Gap::DisconnectionReason_t reason) |
Notifies BLE disconnection to this BLE MIDI instance. | |
void | onBleConnection (Gap::Handle_t handle, Gap::addr_type_t type, const Gap::address_t addr, const Gap::ConnectionParams_t *params) |
Notifies BLE connection to this BLE MIDI instance. |
Detailed Description
A class to communicate a BLE MIDI device.
Definition at line 27 of file BLEMIDI.h.
Constructor & Destructor Documentation
BLEMIDI | ( | BLEDevice * | device ) |
Constructor.
Definition at line 267 of file BLEMIDI.cpp.
BLEMIDI | ( | BLEDevice * | dev, |
char * | deviceName | ||
) |
Constructor with device name.
Definition at line 271 of file BLEMIDI.cpp.
Member Function Documentation
void attachActiveSensing | ( | void(*)() | fn ) |
void attachChannelAftertouch | ( | void(*)(uint8_t, uint8_t) | fn ) |
void attachContinue | ( | void(*)() | fn ) |
void attachControlChange | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
void attachNoteOff | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
void attachNoteOn | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
void attachnProgramChange | ( | void(*)(uint8_t, uint8_t) | fn ) |
void attachPitchWheel | ( | void(*)(uint8_t, uint16_t) | fn ) |
void attachPolyphonicAftertouch | ( | void(*)(uint8_t, uint8_t, uint8_t) | fn ) |
void attachReset | ( | void(*)() | fn ) |
void attachSongPositionPointer | ( | void(*)(uint16_t) | fn ) |
void attachSongSelect | ( | void(*)(uint8_t) | fn ) |
void attachStart | ( | void(*)() | fn ) |
void attachStop | ( | void(*)() | fn ) |
void attachSystemExclusive | ( | void(*)(uint8_t *, uint16_t, bool) | fn ) |
void attachTimeCodeQuarterFrame | ( | void(*)(uint8_t) | fn ) |
void attachTimingClock | ( | void(*)() | fn ) |
void attachTuneRequest | ( | void(*)() | fn ) |
bool connected | ( | ) |
Check if a BLE MIDI device is connected.
- Returns:
- true if a midi device is connected
Definition at line 322 of file BLEMIDI.cpp.
void onBleConnection | ( | Gap::Handle_t | handle, |
Gap::addr_type_t | type, | ||
const Gap::address_t | addr, | ||
const Gap::ConnectionParams_t * | params | ||
) |
Notifies BLE connection to this BLE MIDI instance.
Definition at line 27 of file BLEMIDI.cpp.
void onBleDisconnection | ( | Gap::Handle_t | handle, |
Gap::DisconnectionReason_t | reason | ||
) |
Notifies BLE disconnection to this BLE MIDI instance.
Definition at line 22 of file BLEMIDI.cpp.
void sendActiveSensing | ( | ) |
Send a `Active Sensing` event.
Definition at line 377 of file BLEMIDI.cpp.
void sendChannelAftertouch | ( | uint8_t | channel, |
uint8_t | pressure | ||
) |
Send a `Channel Aftertouch` event.
- Parameters:
-
channel 0-15 pressure 0-127
Definition at line 386 of file BLEMIDI.cpp.
void sendContinue | ( | ) |
Send a `Continue` event.
Definition at line 371 of file BLEMIDI.cpp.
void sendControlChange | ( | uint8_t | channel, |
uint8_t | function, | ||
uint8_t | value | ||
) |
Send a `Control Change` event.
- Parameters:
-
channel 0-15 function 0-127 value 0-127
Definition at line 404 of file BLEMIDI.cpp.
void sendNoteOff | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | velocity | ||
) |
Send a `Note Off` event.
- Parameters:
-
channel 0-15 note 0-127 velocity 0-127
Definition at line 395 of file BLEMIDI.cpp.
void sendNoteOn | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | velocity | ||
) |
Send a `Note On` event.
- Parameters:
-
channel 0-15 note 0-127 velocity 0-127
Definition at line 398 of file BLEMIDI.cpp.
void sendPitchWheel | ( | uint8_t | channel, |
uint16_t | amount | ||
) |
Send a `Pitch Wheel` event.
- Parameters:
-
channel 0-15 amount 0-8192(center)-16383
Definition at line 407 of file BLEMIDI.cpp.
void sendPolyphonicAftertouch | ( | uint8_t | channel, |
uint8_t | note, | ||
uint8_t | pressure | ||
) |
Send a `Polyphonic Aftertouch` event.
- Parameters:
-
channel 0-15 note 0-127 pressure 0-127
Definition at line 401 of file BLEMIDI.cpp.
void sendProgramChange | ( | uint8_t | channel, |
uint8_t | program | ||
) |
Send a `Program Change` event.
- Parameters:
-
channel 0-15 program 0-127
Definition at line 383 of file BLEMIDI.cpp.
void sendReset | ( | ) |
Send a `Reset` event.
Definition at line 380 of file BLEMIDI.cpp.
void sendSongPositionPointer | ( | uint16_t | position ) |
Send a `Song Position Pointer` event.
- Parameters:
-
position 0-16383
Definition at line 410 of file BLEMIDI.cpp.
void sendSongSelect | ( | uint8_t | song ) |
void sendStart | ( | ) |
Send a `Start` event.
Definition at line 368 of file BLEMIDI.cpp.
void sendStop | ( | ) |
Send a `Stop` event.
Definition at line 374 of file BLEMIDI.cpp.
void sendSystemExclusive | ( | uint8_t * | sysex, |
uint16_t | length | ||
) |
Send a `System Exclusive` event.
- Parameters:
-
sysex the data starts with `0xf0` and ends with `0xf7` length
Definition at line 413 of file BLEMIDI.cpp.
void sendTimeCodeQuarterFrame | ( | uint8_t | timing ) |
Send a `Time Code Quarter Frame` event.
- Parameters:
-
timing 0-127
Definition at line 389 of file BLEMIDI.cpp.
void sendTimingClock | ( | ) |
Send a `Timing Clock` event.
Definition at line 365 of file BLEMIDI.cpp.
void sendTuneRequest | ( | ) |
Send a `Tune Request` event.
Definition at line 362 of file BLEMIDI.cpp.
Generated on Tue Jul 12 2022 14:18:15 by
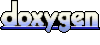