Change buffer sizes to support GR-PEACH
Dependents: GR-PEACH_Azure_Speech
Fork of HTTPClient-SSL by
HTTPFile.h
00001 #ifndef HTTPFILE_H 00002 #define HTTPFILE_H 00003 00004 #include <mbed.h> 00005 #include "../IHTTPData.h" 00006 00007 class HTTPFile : public IHTTPDataIn { 00008 00009 public: 00010 HTTPFile(char* filename); 00011 00012 /** Closes the file, should be called once the http connection is closed. 00013 */ 00014 void close(); 00015 00016 protected: 00017 00018 friend class HTTPClient; 00019 00020 /** Reset stream to its beginning 00021 * Called by the HTTPClient on each new request 00022 */ 00023 virtual void writeReset(); 00024 00025 /** Write a piece of data transmitted by the server 00026 * @param buf Pointer to the buffer from which to copy the data 00027 * @param len Length of the buffer 00028 */ 00029 virtual int write(const char* buf, size_t len); 00030 00031 /** Set MIME type 00032 * @param type Internet media type from Content-Type header 00033 */ 00034 virtual void setDataType(const char* type); 00035 00036 /** Determine whether the data is chunked 00037 * Recovered from Transfer-Encoding header 00038 */ 00039 virtual void setIsChunked(bool chunked); 00040 00041 /** If the data is not chunked, set its size 00042 * From Content-Length header 00043 */ 00044 virtual void setDataLen(size_t len); 00045 private: 00046 FILE *file; 00047 size_t m_len; 00048 bool m_chunked; 00049 }; 00050 #endif
Generated on Wed Jul 13 2022 02:33:44 by
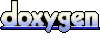