Extended library from C12832 Lib. by Peter Drescher, Chris Styles & Mihail Stoyanov. LCD in the market such as AQM1248A (Akizuki), AD-12864-SPI (antendo), NHD-C12832 (Newhaven), ST7565 (adafruit) and so on
Dependents: CW_Decoder_using_FFT_on_F446 LPC1114_SPI_LCD_ST7565family_test
Fork of C12832 by
TextDisplay.h
00001 /* mbed TextDisplay Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A common base class for Text displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), character (put a character 00008 * at a location), rows and columns (number of rows/cols) functions. 00009 * Everything else (locate, printf, putc, cls) will come for free 00010 * 00011 * The model is the display will wrap at the right and bottom, so you can 00012 * keep writing and will always get valid characters. The location is 00013 * maintained internally to the class to make this easy 00014 */ 00015 00016 #ifndef MBED_TEXTDISPLAY_H 00017 #define MBED_TEXTDISPLAY_H 00018 00019 #include "mbed.h" 00020 #include "Stream.h" // need on mbed-os-6.2.0, Aug. 5th, 2020 by JH1PJL 00021 00022 /** 00023 * TextDisplay interface 00024 */ 00025 class TextDisplay : public Stream { 00026 public: 00027 00028 /** 00029 * Create a TextDisplay interface 00030 * 00031 * @param name The name used in the path to access the strean through the filesystem 00032 */ 00033 TextDisplay(const char *name = NULL); 00034 00035 /** 00036 * Output a character at the given position 00037 * 00038 * @param column column where charater must be written 00039 * @param row where character must be written 00040 * @param c the character to be written to the TextDisplay 00041 */ 00042 virtual void character(int column, int row, int c) = 0; 00043 00044 /** 00045 * Return number of rows on TextDisplay 00046 * 00047 * @results number of rows 00048 */ 00049 virtual int rows() = 0; 00050 00051 /** 00052 * Return number if columns on TextDisplay\ 00053 * 00054 * @results number of rows 00055 */ 00056 virtual int columns() = 0; 00057 00058 // functions that come for free, but can be overwritten 00059 00060 /** 00061 * Redirect output from a stream (stoud, sterr) to display 00062 * 00063 * @param stream stream that shall be redirected to the TextDisplay 00064 */ 00065 virtual bool claim (FILE *stream); 00066 00067 /** 00068 * Clear screen 00069 */ 00070 virtual void cls(); 00071 00072 /** 00073 * Change the cursor position to column, row (in pixels) 00074 */ 00075 virtual void locate(int column, int row); 00076 00077 virtual void foreground(uint16_t colour); 00078 virtual void background(uint16_t colour); 00079 // putc (from Stream) 00080 // printf (from Stream) 00081 00082 protected: 00083 00084 virtual int _putc(int value); 00085 virtual int _getc(); 00086 00087 // character location 00088 uint16_t _column; 00089 uint16_t _row; 00090 00091 // colours 00092 uint16_t _foreground; 00093 uint16_t _background; 00094 char *_path; 00095 }; 00096 00097 #endif
Generated on Tue Jul 12 2022 20:40:34 by
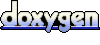