Extended library from C12832 Lib. by Peter Drescher, Chris Styles & Mihail Stoyanov. LCD in the market such as AQM1248A (Akizuki), AD-12864-SPI (antendo), NHD-C12832 (Newhaven), ST7565 (adafruit) and so on
Dependents: CW_Decoder_using_FFT_on_F446 LPC1114_SPI_LCD_ST7565family_test
Fork of C12832 by
ST7565_SPI_LCD.h
00001 /* 00002 EXTEND for SPI interface LCD which is using ST7565 controller 00003 Modified by Kenji Arai / JH1PJL 00004 http://www7b.biglobe.ne.jp/~kenjia/ 00005 https://os.mbed.com/users/kenjiArai/ 00006 Started: September 20th, 2014 00007 Revised: November 29th, 2014 00008 Revised: August 5th, 2020 00009 00010 original file: C12832.h 00011 original Library name: C12832 00012 */ 00013 00014 //---------- ORIGINAL Header --------------------------------------------------- 00015 /* mbed library for the mbed Lab Board 128*32 pixel LCD 00016 * use C12832 controller 00017 * Copyright (c) 2012 Peter Drescher - DC2PD 00018 * Released under the MIT License: http://mbed.org/license/mit 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00021 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00022 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00023 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00024 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00025 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00026 * THE SOFTWARE. 00027 */ 00028 00029 #ifndef ST7565_SPI_LCD_H 00030 #define ST7565_SPI_LCD_H 00031 00032 #include "mbed.h" 00033 #include "GraphicsDisplay.h" 00034 00035 /** Bitmap 00036 */ 00037 struct Bitmap { 00038 int xSize; 00039 int ySize; 00040 int Byte_in_Line; 00041 char* data; 00042 }; 00043 00044 /** SPI LCD control library for ST7565 Controller 00045 * http://www.ladyada.net/learn/lcd/st7565.html 00046 * 00047 * AD-12864-SPI http://www.aitendo.com/product/1622 00048 * AQM12848A http://akizukidenshi.com/catalog/g/gK-07007/ 00049 * 00050 * @code 00051 * #include "mbed.h" 00052 * 00053 * #if 1 00054 * // mosi, sck, reset, a0, ncs 00055 * ST7565 lcd(dp2, dp6, dp10, dp4, dp9, ST7565::AD12864SPI); 00056 * #else 00057 * SPI spi_lcd(dp2, dp1, dp6); // mosi, miso, sck 00058 * // spi, reset, a0, ncs 00059 * ST7565 lcd(spi_lcd, dp10, dp4, dp9, ST7565::AD12864SPI); 00060 * #endif 00061 * 00062 * int main() { 00063 * lcd.cls(); 00064 * lcd.set_contrast(0x06); 00065 * lcd.printf("123456789012345678901234567890\r\n"); 00066 * lcd.rect(10,10,100,50,BLACK); 00067 * lcd.circle(10,10,10,BLACK); 00068 * lcd.fillcircle(50,40,10,BLACK); 00069 * lcd.line(0,0,110,60,BLACK); 00070 * while(1){;} 00071 * } 00072 * @endcode 00073 */ 00074 00075 class ST7565 : public GraphicsDisplay 00076 { 00077 public: 00078 00079 /** LCD panel format */ 00080 enum LCDType { 00081 LCD128x32, 00082 LCD128x48, 00083 LCD128x64, 00084 AQM1248A = LCD128x48, 00085 AD12864SPI = LCD128x64, 00086 ST7565LCD = LCD128x64 00087 }; 00088 00089 /** 00090 * Create a ST7565 object connected to SPI1 00091 */ 00092 ST7565(PinName mosi, PinName sck, 00093 PinName reset, 00094 PinName a0, 00095 PinName ncs, 00096 LCDType type, 00097 const char* name = "LCD" 00098 ); 00099 ST7565(SPI& _spi, 00100 PinName reset, 00101 PinName a0, 00102 PinName ncs, 00103 LCDType type, 00104 const char* name = "LCD" 00105 ); 00106 00107 /** 00108 * Get the width of the screen in pixel 00109 * 00110 * @returns width of screen in pixel 00111 * 00112 */ 00113 virtual int width(); 00114 00115 /** 00116 * Get the height of the screen in pixel 00117 * 00118 * @returns height of screen in pixel 00119 */ 00120 virtual int height(); 00121 00122 /** 00123 * Draw a pixel at x,y black or white 00124 * 00125 * @param x horizontal position 00126 * @param y vertical position 00127 * @param color - 1 set pixel, 0 erase pixel 00128 */ 00129 virtual void pixel(int x, int y,int colour); 00130 00131 /** 00132 * Draw a circle 00133 * 00134 * @param x0,y0 center 00135 * @param r radius 00136 * @param color - 1 set pixel, 0 erase pixel 00137 */ 00138 void circle(int x, int y, int r, int colour); 00139 00140 /** 00141 * Draw a filled circle 00142 * 00143 * @param x0,y0 center 00144 * @param r radius 00145 * @param color - 1 set pixel, 0 erase pixel 00146 * 00147 * Use circle with different radius, 00148 * Can miss some pixels 00149 */ 00150 void fillcircle(int x, int y, int r, int colour); 00151 00152 /** 00153 * Draw a 1 pixel line 00154 * 00155 * @param x0,y0 start point 00156 * @param x1,y1 stop point 00157 * @param color - 1 set pixel, 0 erase pixel 00158 */ 00159 void line(int x0, int y0, int x1, int y1, int colour); 00160 00161 /** 00162 * Draw a rect 00163 * 00164 * @param x0,y0 top left corner 00165 * @param x1,y1 down right corner 00166 * @param color - 1 set pixel, 0 erase pixel 00167 */ 00168 void rect(int x0, int y0, int x1, int y1, int colour); 00169 00170 /** 00171 * Draw a filled rect 00172 * 00173 * @param x0,y0 top left corner 00174 * @param x1,y1 down right corner 00175 * @param color - 1 set pixel, 0 erase pixel 00176 */ 00177 void fillrect(int x0, int y0, int x1, int y1, int colour); 00178 00179 /** 00180 * set the contrast level 00181 */ 00182 void set_contrast(unsigned int o); 00183 00184 /** 00185 * read the contrast level 00186 */ 00187 unsigned int get_contrast(void); 00188 00189 /** 00190 * Invert the screen 00191 * 00192 * @param o = 0 normal, 1 invert 00193 */ 00194 void invert(unsigned int o); 00195 00196 /** 00197 * Clear the screen 00198 */ 00199 virtual void cls(void); 00200 00201 /** 00202 * Set the drawing mode 00203 * 00204 * @param mode NORMAl or XOR 00205 */ 00206 void setmode(int mode); 00207 00208 /** 00209 * Calculate the max number of columns. 00210 * Depends on actual font size 00211 * 00212 * @returns max column 00213 */ 00214 virtual int columns(void); 00215 00216 /** 00217 * calculate the max number of rows 00218 * 00219 * @returns max rows 00220 * depends on actual font size 00221 * 00222 */ 00223 virtual int rows(void); 00224 00225 /** 00226 * Draw a character on given position out of the active font to the LCD 00227 * 00228 * @param x x-position of char (top left) 00229 * @param y y-position 00230 * @param c char to print 00231 */ 00232 virtual void character(int x, int y, int c); 00233 00234 /** 00235 * Setup cursor position 00236 * 00237 * @param x x-position (top left) 00238 * @param y y-position 00239 */ 00240 virtual void locate(int x, int y); 00241 00242 /** 00243 * Setup auto update of screen 00244 * 00245 * @param up 1 = on , 0 = off 00246 * 00247 * if switched off the program has to call copy_to_lcd() 00248 * to update screen from framebuffer 00249 */ 00250 void set_auto_up(unsigned int up); 00251 00252 /** 00253 * Get status of the auto update function 00254 * 00255 * @returns if auto update is on 00256 */ 00257 unsigned int get_auto_up(void); 00258 00259 /** 00260 * Select the font to use 00261 * 00262 * @param f pointer to font array 00263 * 00264 * font array can created with GLCD Font Creator from http://www.mikroe.com 00265 * you have to add 4 parameter at the beginning of the font array to use: 00266 * - the number of byte / char 00267 * - the vertial size in pixel 00268 * - the horizontal size in pixel 00269 * - the number of byte per vertical line 00270 * you also have to change the array to char[] 00271 */ 00272 void set_font(unsigned char* f); 00273 00274 /** 00275 * Print bitmap to buffer 00276 * 00277 * @param bm Bitmap in flash 00278 * @param x x start 00279 * @param y y start 00280 */ 00281 void print_bm(Bitmap bm, int x, int y); 00282 00283 #if DOXYGEN_ONLY 00284 /** 00285 * Write a character to the LCD 00286 * 00287 * @param c The character to write to the display 00288 */ 00289 int putc(int c); 00290 00291 /** 00292 * Write a formated string to the LCD 00293 * 00294 * @param format A printf-style format string, followed by the 00295 * variables to use in formating the string. 00296 */ 00297 int printf(const char* format, ...); 00298 #endif 00299 00300 unsigned char* font; 00301 unsigned int draw_mode; 00302 00303 protected: 00304 /** Draw color 00305 */ 00306 enum {WHITE = 0,BLACK}; 00307 00308 /** Draw mode 00309 * NORMAl 00310 * XOR set pixel by xor the screen 00311 */ 00312 enum {NORMAL,XOR}; 00313 00314 /** Vars */ 00315 SPI *_spi_p; 00316 SPI &_spi; 00317 DigitalOut _reset; 00318 DigitalOut _A0; 00319 DigitalOut _CS; 00320 00321 void hline(int x0, int x1, int y, int colour); 00322 void vline(int y0, int y1, int x, int colour); 00323 void lcd_reset(); 00324 void wr_dat(unsigned char value); 00325 void wr_cmd(unsigned char value); 00326 void wr_cnt(unsigned char cmd); 00327 void line_clear(int y); 00328 void copy_to_lcd(void); 00329 void initialize(LCDType type); 00330 00331 virtual int _putc(int value); 00332 00333 unsigned int lcd_width; 00334 unsigned int lcd_height; 00335 unsigned int char_x; 00336 unsigned int char_y; 00337 unsigned char buffer[1024]; 00338 unsigned int contrast; 00339 unsigned int auto_up; 00340 }; 00341 00342 #endif
Generated on Tue Jul 12 2022 20:40:34 by
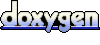