change float to double
Fork of PID by
Embed:
(wiki syntax)
Show/hide line numbers
PID.h
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * A PID controller is a widely used feedback controller commonly found in 00029 * industry. 00030 * 00031 * This library is a port of Brett Beauregard's Arduino PID library: 00032 * 00033 * http://www.arduino.cc/playground/Code/PIDLibrary 00034 * 00035 * The wikipedia article on PID controllers is a good place to start on 00036 * understanding how they work: 00037 * 00038 * http://en.wikipedia.org/wiki/PID_controller 00039 * 00040 * For a clear and elegant explanation of how to implement and tune a 00041 * controller, the controlguru website by Douglas J. Cooper (who also happened 00042 * to be Brett's controls professor) is an excellent reference: 00043 * 00044 * http://www.controlguru.com/ 00045 */ 00046 00047 // Modified by K.Arai/JH1PJL on June 15th, 2016 00048 00049 #ifndef PID_H 00050 #define PID_H 00051 00052 /** 00053 * Includes 00054 */ 00055 #include "mbed.h" 00056 00057 /** 00058 * Defines 00059 */ 00060 #define MANUAL_MODE 0 00061 #define AUTO_MODE 1 00062 00063 /** 00064 * Proportional-integral-derivative controller. 00065 */ 00066 class PID { 00067 00068 public: 00069 00070 /** 00071 * Constructor. 00072 * 00073 * Sets default limits [0-3.3V], calculates tuning parameters, and sets 00074 * manual mode with no bias. 00075 * 00076 * @param Kc - Tuning parameter 00077 * @param tauI - Tuning parameter 00078 * @param tauD - Tuning parameter 00079 * @param interval PID calculation performed every interval seconds. 00080 */ 00081 PID(double Kc, double tauI, double tauD, double interval); 00082 00083 /** 00084 * Scale from inputs to 0-100%. 00085 * 00086 * @param InMin The real world value corresponding to 0%. 00087 * @param InMax The real world value corresponding to 100%. 00088 */ 00089 void setInputLimits(double inMin , double inMax); 00090 00091 /** 00092 * Scale from outputs to 0-100%. 00093 * 00094 * @param outMin The real world value corresponding to 0%. 00095 * @param outMax The real world value corresponding to 100%. 00096 */ 00097 void setOutputLimits(double outMin, double outMax); 00098 00099 /** 00100 * Calculate PID constants. 00101 * 00102 * Allows parameters to be changed on the fly without ruining calculations. 00103 * 00104 * @param Kc - Tuning parameter 00105 * @param tauI - Tuning parameter 00106 * @param tauD - Tuning parameter 00107 */ 00108 void setTunings(double Kc, double tauI, double tauD); 00109 00110 /** 00111 * Reinitializes controller internals. Automatically 00112 * called on a manual to auto transition. 00113 */ 00114 void reset(void); 00115 00116 /** 00117 * Set PID to manual or auto mode. 00118 * 00119 * @param mode 0 -> Manual 00120 * Non-zero -> Auto 00121 */ 00122 void setMode(int mode); 00123 00124 /** 00125 * Set how fast the PID loop is run. 00126 * 00127 * @param interval PID calculation peformed every interval seconds. 00128 */ 00129 void setInterval(double interval); 00130 00131 /** 00132 * Set the set point. 00133 * 00134 * @param sp The set point as a real world value. 00135 */ 00136 void setSetPoint(double sp); 00137 00138 /** 00139 * Set the process value. 00140 * 00141 * @param pv The process value as a real world value. 00142 */ 00143 void setProcessValue(double pv); 00144 00145 /** 00146 * Set the bias. 00147 * 00148 * @param bias The bias for the controller output. 00149 */ 00150 void setBias(double bias); 00151 00152 /** 00153 * PID calculation. 00154 * 00155 * @return The controller output as a double between outMin and outMax. 00156 */ 00157 double compute(void); 00158 00159 //Getters. 00160 double getInMin(); 00161 double getInMax(); 00162 double getOutMin(); 00163 double getOutMax(); 00164 double getInterval(); 00165 double getPParam(); 00166 double getIParam(); 00167 double getDParam(); 00168 00169 private: 00170 00171 bool usingFeedForward; 00172 bool inAuto; 00173 00174 //Actual tuning parameters used in PID calculation. 00175 double Kc_; 00176 double tauR_; 00177 double tauD_; 00178 00179 //Raw tuning parameters. 00180 double pParam_; 00181 double iParam_; 00182 double dParam_; 00183 00184 //The point we want to reach. 00185 double setPoint_; 00186 //The thing we measure. 00187 double processVariable_; 00188 double prevProcessVariable_; 00189 //The output that affects the process variable. 00190 double controllerOutput_; 00191 double prevControllerOutput_; 00192 00193 //We work in % for calculations so these will scale from 00194 //real world values to 0-100% and back again. 00195 double inMin_; 00196 double inMax_; 00197 double inSpan_; 00198 double outMin_; 00199 double outMax_; 00200 double outSpan_; 00201 00202 //The accumulated error, i.e. integral. 00203 double accError_; 00204 //The controller output bias. 00205 double bias_; 00206 00207 //The interval between samples. 00208 double tSample_; 00209 00210 //Controller output as a real world value. 00211 volatile double realOutput_; 00212 00213 }; 00214 00215 #endif /* PID_H */
Generated on Sun Jul 17 2022 01:38:02 by
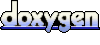