Small, versatile 9-axis sensor module by Bosch Sensortec 3D Accelerometer + 3D Gyroscope + 3D Magnetometer
Dependents: Pruebas_Flex_IMU_copy BMX055_Madgwick
BMX055 Class Reference
BMX055 Small, versatile 9-axis sensor module by Bosch Sensortec. More...
#include <BMX055.h>
Public Member Functions | |
BMX055 (PinName p_sda, PinName p_scl) | |
Configure data pin. | |
BMX055 (I2C &p_i2c) | |
Configure data pin (with other devices on I2C line) | |
void | get_accel (BMX055_ACCEL_TypeDef *acc) |
Get accel data. | |
void | get_gyro (BMX055_GYRO_TypeDef *gyr) |
Get gyroscope data. | |
void | get_magnet (BMX055_MAGNET_TypeDef *mag) |
Get magnet data. | |
float | get_chip_temperature (void) |
Get Chip temperature data both Acc & Gyro. | |
void | read_id_inf (BMX055_ID_INF_TypeDef *id) |
Read BMX055 ID information. | |
bool | chip_ready (void) |
Check chip is avairable or not. | |
void | set_parameter (const BMX055_TypeDef *bmx055_parameter) |
Check chip is avairable or not. | |
void | frequency (int hz) |
Set I2C clock frequency. | |
uint8_t | read_reg (uint8_t addr) |
Read register. | |
uint8_t | write_reg (uint8_t addr, uint8_t data) |
Write register. |
Detailed Description
BMX055 Small, versatile 9-axis sensor module by Bosch Sensortec.
#include "mbed.h" #include "BMX055.h" Serial pc(USBTX,USBRX); I2C (I2C_SDA, I2C_SCL); BMX055 imu(i2c); const BMX055_TypeDef bmx055_my_parameters = { // ACC ACC_2G, ACC_BW250Hz, // GYR GYR_125DPS, GYR_200Hz23Hz, // MAG MAG_ODR10Hz }; int main() { BMX055_ACCEL_TypeDef acc; BMX055_GYRO_TypeDef gyr; BMX055_MAGNET_TypeDef mag; if (imu.imu.chip_ready() == 0){ pc.printf("Bosch BMX055 is NOT avirable!!\r\n"); } imu.set_parameter(&bmx055_my_parameters); while(1) { imu.get_accel(&acc); pc.printf("//ACC: x=%+3.2f y=%+3.2f z=%+3.2f //", acc.x, acc.y, acc.z); imu.get_gyro(&gyr); pc.printf("GYR: x=%+3.2f y=%+3.2f z=%+3.2f //", gyr.x, gyr.y, gyr.z); imu.get_magnet(&mag); pc.printf("MAG: x=%+3.2f y=%+3.2f z=%+3.2f , passed %u sec\r\n", mag.x, mag.y, mag.z, n++); wait(0.5f); } }
Definition at line 201 of file BMX055.h.
Constructor & Destructor Documentation
BMX055 | ( | PinName | p_sda, |
PinName | p_scl | ||
) |
Configure data pin.
- Parameters:
-
data SDA and SCL pins Other parameters are set automatically
Definition at line 26 of file BMX055.cpp.
BMX055 | ( | I2C & | p_i2c ) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition Other parameters are set automatically
Definition at line 33 of file BMX055.cpp.
Member Function Documentation
bool chip_ready | ( | void | ) |
Check chip is avairable or not.
- Parameters:
-
none
- Returns:
- OK = true, NG = false;
Definition at line 433 of file BMX055.cpp.
void frequency | ( | int | hz ) |
void get_accel | ( | BMX055_ACCEL_TypeDef * | acc ) |
float get_chip_temperature | ( | void | ) |
Get Chip temperature data both Acc & Gyro.
- Parameters:
-
none
- Returns:
- temperature data
Definition at line 182 of file BMX055.cpp.
void get_gyro | ( | BMX055_GYRO_TypeDef * | gyr ) |
Get gyroscope data.
- Parameters:
-
float type of 3D data address
Definition at line 89 of file BMX055.cpp.
void get_magnet | ( | BMX055_MAGNET_TypeDef * | mag ) |
Get magnet data.
- Parameters:
-
float type of 3D data address
Definition at line 159 of file BMX055.cpp.
void read_id_inf | ( | BMX055_ID_INF_TypeDef * | id ) |
Read BMX055 ID information.
- Parameters:
-
ID information address
- Returns:
- none
Definition at line 425 of file BMX055.cpp.
uint8_t read_reg | ( | uint8_t | addr ) |
Read register.
- Parameters:
-
register's address
- Returns:
- register data
Definition at line 448 of file BMX055.cpp.
void set_parameter | ( | const BMX055_TypeDef * | bmx055_parameter ) |
Check chip is avairable or not.
- Parameters:
-
configration parameter
- Returns:
- none
Definition at line 40 of file BMX055.cpp.
uint8_t write_reg | ( | uint8_t | addr, |
uint8_t | data | ||
) |
Write register.
- Parameters:
-
register's address data
- Returns:
- register data
Definition at line 456 of file BMX055.cpp.
Generated on Tue Jul 12 2022 20:19:11 by
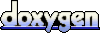