Analog Devices 3-axis accelerometer. I2C interface
ADXL345 Class Reference
Interface for Analog Devices : 3-axis accelerometer Chip: ADXL345. More...
#include <ADXL345.h>
Public Member Functions | |
ADXL345 (PinName p_sda, PinName p_scl, uint8_t addr, uint8_t data_rate, uint8_t fullscale) | |
Configure data pin (with other devices on I2C line) | |
ADXL345 (PinName p_sda, PinName p_scl, uint8_t addr) | |
Configure data pin (with other devices on I2C line) | |
ADXL345 (PinName p_sda, PinName p_scl) | |
Configure data pin (with other devices on I2C line) | |
ADXL345 (I2C &p_i2c, uint8_t addr, uint8_t data_rate, uint8_t fullscale) | |
Configure data pin (with other devices on I2C line) | |
ADXL345 (I2C &p_i2c, uint8_t addr) | |
Configure data pin (with other devices on I2C line) | |
ADXL345 (I2C &p_i2c) | |
Configure data pin (with other devices on I2C line) | |
void | read_data (float *dt_usr) |
Read a float type data from acc. | |
void | read_mg_data (float *dt_usr) |
Read a float type data from acc. | |
void | read_g_data (float *dt_usr) |
Read a float type data from acc. | |
uint8_t | read_id () |
Read a acc ID number. | |
bool | data_ready () |
Read Data Ready flag. | |
void | frequency (int hz) |
Set I2C clock frequency. | |
uint8_t | read_reg (uint8_t addr) |
Read register (general purpose) | |
void | write_reg (uint8_t addr, uint8_t data) |
Write register (general purpose) | |
void | debug_print (void) |
data print for debug | |
void | self_test (void) |
Self-Test Feature. |
Detailed Description
Interface for Analog Devices : 3-axis accelerometer Chip: ADXL345.
#include "mbed.h" // I2C Communication I2C i2c(D14,D15); // SDA, SCL ADXL345 acc(i2c); int main() { float f[3]; while(1){ acc.read_data(f); } }
Definition at line 118 of file ADXL345.h.
Constructor & Destructor Documentation
ADXL345 | ( | PinName | p_sda, |
PinName | p_scl, | ||
uint8_t | addr, | ||
uint8_t | data_rate, | ||
uint8_t | fullscale | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C PinName SDA &SDL device address output data rate selection, power down mode, 0.1Hz to 3.2KHz full scale selection, +/-2g to +/-16g
Definition at line 34 of file ADXL345.cpp.
ADXL345 | ( | PinName | p_sda, |
PinName | p_scl, | ||
uint8_t | addr | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition device address
Definition at line 42 of file ADXL345.cpp.
ADXL345 | ( | PinName | p_sda, |
PinName | p_scl | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition
Definition at line 49 of file ADXL345.cpp.
ADXL345 | ( | I2C & | p_i2c, |
uint8_t | addr, | ||
uint8_t | data_rate, | ||
uint8_t | fullscale | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition device address output data rate selection, power down mode, 0.1Hz to 3.2KHz full scale selection, +/-2g to +/-16g
Definition at line 59 of file ADXL345.cpp.
ADXL345 | ( | I2C & | p_i2c, |
uint8_t | addr | ||
) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition output data rate selection = 100Hz full scale selection = +/-2g
Definition at line 66 of file ADXL345.cpp.
ADXL345 | ( | I2C & | p_i2c ) |
Configure data pin (with other devices on I2C line)
- Parameters:
-
I2C previous definition address check both G & V output data rate selection = 100Hz full scale selection = +/-2g
Definition at line 72 of file ADXL345.cpp.
Member Function Documentation
bool data_ready | ( | ) |
Read Data Ready flag.
- Parameters:
-
none
- Returns:
- true = Ready
Definition at line 202 of file ADXL345.cpp.
void debug_print | ( | void | ) |
void frequency | ( | int | hz ) |
void read_data | ( | float * | dt_usr ) |
Read a float type data from acc.
- Parameters:
-
float type of three arry's address, e.g. float dt_usr[3];
- Returns:
- acc motion data unit: m/s/s(m/s2)
- dt_usr[0]->x, dt_usr[1]->y, dt_usr[2]->z
Definition at line 164 of file ADXL345.cpp.
void read_g_data | ( | float * | dt_usr ) |
Read a float type data from acc.
- Parameters:
-
float type of three arry's address, e.g. float dt_usr[3];
- Returns:
- acc motion data unit: g
- dt_usr[0]->x, dt_usr[1]->y, dt_usr[2]->z
Definition at line 159 of file ADXL345.cpp.
uint8_t read_id | ( | ) |
Read a acc ID number.
- Parameters:
-
none
- Returns:
- ID is okay (I_AM_ ADXL345(0x33)) or not
Definition at line 194 of file ADXL345.cpp.
void read_mg_data | ( | float * | dt_usr ) |
Read a float type data from acc.
- Parameters:
-
float type of three arry's address, e.g. float dt_usr[3];
- Returns:
- acc motion data unit: mg
- dt_usr[0]->x, dt_usr[1]->y, dt_usr[2]->z
Definition at line 154 of file ADXL345.cpp.
uint8_t read_reg | ( | uint8_t | addr ) |
Read register (general purpose)
- Parameters:
-
register's address
- Returns:
- register data
Definition at line 222 of file ADXL345.cpp.
void self_test | ( | void | ) |
void write_reg | ( | uint8_t | addr, |
uint8_t | data | ||
) |
Write register (general purpose)
- Parameters:
-
register's address data
- Returns:
- none
Definition at line 234 of file ADXL345.cpp.
Generated on Wed Jul 27 2022 17:10:48 by
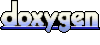