Just a simple library for VLSI's mp3/midi codec chip
Dependents: IsuProject_LPC1768
VS1053.h
00001 // ==================================================== Dec 21 2013, kayeks == 00002 // VS1053.h 00003 // =========================================================================== 00004 // Just a simple library for VLSI's mp3/midi codec chip 00005 // - Minimal and simple implementation (and dirty too) 00006 00007 #ifndef KAYX_VS1053_H_ 00008 #define KAYX_VS1053_H_ 00009 00010 /** Class VS1053. Drives VLSI's mp3/midi codec chip. */ 00011 class VS1053 { 00012 private: 00013 SPI spi; 00014 DigitalOut cs; 00015 DigitalOut bsync; 00016 DigitalIn dreq; 00017 DigitalOut rst; 00018 00019 public: 00020 static const uint8_t SCI_MODE = 0x00; 00021 static const uint8_t SCI_STATUS = 0x01; 00022 static const uint8_t SCI_BASS = 0x02; 00023 static const uint8_t SCI_CLOCKF = 0x03; 00024 static const uint8_t SCI_DECODE_TIME = 0x04; 00025 static const uint8_t SCI_AUDATA = 0x05; 00026 static const uint8_t SCI_WRAM = 0x06; 00027 static const uint8_t SCI_WRAMADDR = 0x07; 00028 static const uint8_t SCI_HDAT0 = 0x08; 00029 static const uint8_t SCI_HDAT1 = 0x09; 00030 static const uint8_t SCI_AIADDR = 0x0a; 00031 static const uint8_t SCI_VOL = 0x0b; 00032 static const uint8_t SCI_AICTRL0 = 0x0c; 00033 static const uint8_t SCI_AICTRL1 = 0x0d; 00034 static const uint8_t SCI_AICTRL2 = 0x0e; 00035 static const uint8_t SCI_AICTRL3 = 0x0f; 00036 00037 VS1053(PinName mosiPin, PinName misoPin, PinName sckPin, 00038 PinName csPin, PinName bsyncPin, PinName dreqPin, PinName rstPin, 00039 uint32_t spiFrequency=1000000); 00040 ~VS1053(); 00041 void hardwareReset(); 00042 void sendDataByte(uint8_t data); 00043 size_t sendDataBlock(uint8_t* data, size_t length); 00044 void clockUp(); 00045 bool sendCancel(); 00046 bool stop(); 00047 00048 private: 00049 void writeReg(uint8_t, uint16_t); 00050 uint16_t readReg(uint8_t); 00051 }; 00052 00053 #endif
Generated on Tue Jul 12 2022 21:40:39 by
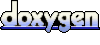