software to control a DC motor, preferably interfacing with a motor driver.
Embed:
(wiki syntax)
Show/hide line numbers
DCMotor.h
00001 #ifndef MBED_DCMOTOR_H 00002 #define MBED_DCMOTOR_H 00003 00004 #include "mbed.h" 00005 00006 /**DC motor control class with PWM control 00007 * 00008 * Example: 00009 * @code 00010 * 00011 * #include "mbed.h" 00012 * #include "DCMotor.h" 00013 * 00014 * DCMotor a(p21,p22,p23); 00015 * DCMotor b(p24,p25,p26); 00016 * int main() { 00017 * a.driveIt(50); 00018 * b.driveIt(50); 00019 * } 00020 * 00021 * @endcode 00022 */ 00023 00024 class DCMotor { 00025 00026 public: 00027 /** create a DCMotor object connected to the pins with speed control 00028 * @param PWMPin PWM pin to control speed of motor 00029 * @param PinA Digital output pin to connect to motor 00030 * @param PinB Digital output pin to connect to motor 00031 */ 00032 DCMotor(PinName PWMPin, PinName PinA, PinName PinB); 00033 00034 /** drive Motor input range (-100 to 100). 00035 * @param perCent PWM pin to control speed of motor 00036 */ 00037 void driveIt(float); 00038 00039 protected: 00040 PwmOut _PWMPin; 00041 DigitalOut _PinA; 00042 DigitalOut _PinB; 00043 }; 00044 00045 #endif
Generated on Tue Jul 12 2022 13:19:22 by
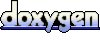