
Tweeting with mbed! (using SuperTweet)
Dependencies: EthernetNetIf NTPClient_NetServices TextLCD mbed
main.cpp
00001 /* 00002 * UDENOKAI #5 SAMPLE PROGRAM: Tweeting with mbed! 00003 * 00004 * Using SuperTweet.Net API Proxy (setup required). 00005 * Based on cookbook/Twitter 00006 */ 00007 #include "mbed.h" 00008 #include "EthernetNetIf.h" 00009 #include "HTTPClient.h" 00010 #include "NTPClient.h" 00011 #include "TextLCD.h" 00012 00013 Serial pc(USBTX, USBRX); // tx, rx 00014 TextLCD lcd(p24, p26, p27, p28, p29, p30); // rs, e, d4-d7 00015 EthernetNetIf eth; 00016 NTPClient ntp; 00017 00018 int main() { 00019 00020 // Tweet informations 00021 char message[64], name[32], twID[32], twPW[32]; 00022 sprintf(message, "hogehogefugafuga"); 00023 sprintf(name, "hoge"); // ex) @kagamikan 00024 sprintf(twID, "fuga"); // ex) udenokai 00025 sprintf(twPW, "piyo"); // ex) hoge 00026 00027 // Setup IP Network 00028 pc.printf("\r\nSetting up...\r\n"); 00029 lcd.printf("Setting up..."); 00030 EthernetErr ethErr = eth.setup(); 00031 if(ethErr) { 00032 printf("Error %d in setup.\n", ethErr); 00033 lcd.printf("\nError %d in setup.", ethErr); 00034 return -1; 00035 } 00036 IpAddr ip = eth.getIp(); 00037 pc.printf("\r\nSetup OK\r\n"); 00038 lcd.cls(); 00039 lcd.printf("IP Address:\n%d.%d.%d.%d", ip[0], ip[1], ip[2], ip[3]); 00040 00041 // Setting time with NTP 00042 Host server(IpAddr(), 123, "ntp.nict.jp"); 00043 ntp.setTime(server); 00044 time_t ctTime; 00045 ctTime = time(NULL); 00046 ctTime += 32400; //set jst time 00047 00048 // Building the tweet 00049 char tweet[128], ts[32]; 00050 strftime(ts, 32, "%I:%M %p\n", localtime(&ctTime)); 00051 sprintf(tweet, "%s (%s's mbed at %s JST) #udenokai", message, name, ts); 00052 pc.printf(tweet); 00053 00054 // Sending the tweet 00055 HTTPClient twitter; 00056 HTTPMap msg; 00057 msg["status"] = tweet; 00058 twitter.basicAuth(twID, twPW); 00059 HTTPResult r = twitter.post("http://api.supertweet.net/1/statuses/update.xml", msg, NULL); 00060 if(r == HTTP_OK) { 00061 pc.printf("Tweet success!\n"); 00062 lcd.cls(); 00063 lcd.printf("\nTweet success!"); 00064 } else { 00065 pc.printf("Tweet failed (Code:%d)\n", r); 00066 lcd.cls(); 00067 lcd.printf("\nTweet failed (%d)", r); 00068 } 00069 00070 return 0; 00071 }
Generated on Sat Jul 16 2022 07:42:35 by
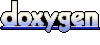