Basic library for SHARP LCD LS027B4DH01/LS027B7DH01
Dependents: AkiSpiLcd_demo AkiSpiLcd_demo2 LCDRAM AkiSpiLcd_example
AkiSpiLcd Class Reference
mbed library for SHARP LCD LS027B4DH01 More...
#include <AkiSpiLcd.h>
Public Types | |
enum | BASE_ADDR |
base address list for 23K256 More... | |
Public Member Functions | |
AkiSpiLcd (PinName mosi, PinName miso, PinName sck, PinName csl, PinName csr) | |
Constructor. | |
void | cls () |
Clear screen. | |
void | cls_ram (int screen) |
Clear screen of SRAM. | |
void | directUpdateSingle (int line, uint8_t *data) |
place a dot pixel | |
void | directUpdateMulti (int startline, int length, uint8_t *data) |
Writes multi lines(400 x N bits = 50 x N bytes) | |
void | cominvert () |
Inverting internal COM signal. | |
void | ramReadSingleLine (int line, uint8_t *buffer, int screen) |
Reads single line (400 bits = 50 bytes) from a screen and writes into buffer. | |
void | ramReadMultiLine (int startline, int length, uint8_t *buffer, int screen) |
Reads multi lines(400 x N bits = 50 x N bytes) from a screen. | |
void | ramWriteSingleLine (int line, uint8_t *data, int screen) |
Writes single line (400 bits = 50 bytes) into a screen. | |
void | ramWriteMultiLine (int startline, int length, uint8_t *data, int screen) |
Writes multi lines(400 x N bits = 50 x N bytes) into a screen. | |
void | ram2lcd (int startline, int length, int screen) |
copies whole data in screen into LCD | |
void | ram2lcd (int screen) |
copies whole data in screen into LCD | |
uint8_t | ram_read (int address) |
read a byte from SRAM (copied from Ser23K256) | |
void | ram_read (int address, uint8_t *buffer, int count) |
read multiple bytes from SRAM into a buffer (copied from Ser23K256) | |
void | ram_write (int address, uint8_t byte) |
write a byte to SRAM (copied from Ser23K256) | |
void | ram_write (int address, uint8_t *buffer, int count) |
write multiple bytes to SRAM from a buffer (copied from Ser23K256) |
Detailed Description
mbed library for SHARP LCD LS027B4DH01
Example:
#include "mbed.h" #include "AkiSpiLcd.h" AkiSpiLcd LCD(MOSI_, MISO_, SCK_, D2, D5); extern const uint8_t hogepic[]; int main() { wait_ms(1); LCD.cls(); LCD.directUpdateSingle(10,(uint8_t*)(hogepic+2000)); LCD.directUpdateMulti(100,(240-100),(uint8_t*)(hogepic)); while(1) { for(int i=0; i<240; i++) { LCD.directUpdateMulti(i,(240-i),(uint8_t*)(hogepic)); LCD.directUpdateMulti(0,(i),(uint8_t*)(hogepic+50*(240-i))); } } }
Definition at line 92 of file AkiSpiLcd.h.
Member Enumeration Documentation
enum BASE_ADDR |
base address list for 23K256
- Parameters:
-
SCREEN0_BASE = 0x0000, SCREEN1_BASE = 0x3000, RAMLINE_BASE = 0x6000,
Definition at line 100 of file AkiSpiLcd.h.
Constructor & Destructor Documentation
AkiSpiLcd | ( | PinName | mosi, |
PinName | miso, | ||
PinName | sck, | ||
PinName | csl, | ||
PinName | csr | ||
) |
Constructor.
- Parameters:
-
mosi SPI data output from mbed mosi SPI data input from slave sck SPI clock output from mbed csl HIGH-active chip select input for LCD csr LOW-active chip select input for SRAM
Definition at line 28 of file AkiSpiLcd.cpp.
Member Function Documentation
void cls | ( | ) |
Clear screen.
Definition at line 38 of file AkiSpiLcd.cpp.
void cls_ram | ( | int | screen ) |
Clear screen of SRAM.
- Parameters:
-
screen screen number (0 or 1)
Definition at line 49 of file AkiSpiLcd.cpp.
void cominvert | ( | ) |
Inverting internal COM signal.
Definition at line 98 of file AkiSpiLcd.cpp.
void directUpdateMulti | ( | int | startline, |
int | length, | ||
uint8_t * | data | ||
) |
Writes multi lines(400 x N bits = 50 x N bytes)
- Parameters:
-
line line number(1-240) length number of line to write *data pointer to data
Definition at line 76 of file AkiSpiLcd.cpp.
void directUpdateSingle | ( | int | line, |
uint8_t * | data | ||
) |
place a dot pixel
- Parameters:
-
x x position y y position Writes single line(400 bits = 50 bytes) line line number(1-240) *data pointer to data
Definition at line 58 of file AkiSpiLcd.cpp.
void ram2lcd | ( | int | startline, |
int | length, | ||
int | screen | ||
) |
copies whole data in screen into LCD
- Parameters:
-
startline starting line number(1-240) length number of line to read screen screen to copy (0 or 1)
Definition at line 189 of file AkiSpiLcd.cpp.
void ram2lcd | ( | int | screen ) |
copies whole data in screen into LCD
- Parameters:
-
screen screen to copy (0 or 1)
Definition at line 234 of file AkiSpiLcd.cpp.
void ram_read | ( | int | address, |
uint8_t * | buffer, | ||
int | count | ||
) |
read multiple bytes from SRAM into a buffer (copied from Ser23K256)
- Parameters:
-
address The SRAM address to read from buffer The buffer to read into (must be big enough!) count The number of bytes to read
Definition at line 248 of file AkiSpiLcd.cpp.
uint8_t ram_read | ( | int | address ) |
read a byte from SRAM (copied from Ser23K256)
- Parameters:
-
address The address to read from
- Returns:
- the character at that address
Definition at line 241 of file AkiSpiLcd.cpp.
void ram_write | ( | int | address, |
uint8_t * | buffer, | ||
int | count | ||
) |
write multiple bytes to SRAM from a buffer (copied from Ser23K256)
- Parameters:
-
address The SRAM address write to buffer The buffer to write from count The number of bytes to write
Definition at line 264 of file AkiSpiLcd.cpp.
void ram_write | ( | int | address, |
uint8_t | byte | ||
) |
write a byte to SRAM (copied from Ser23K256)
- Parameters:
-
address The SRAM address to write to byte The byte to write there
Definition at line 258 of file AkiSpiLcd.cpp.
void ramReadMultiLine | ( | int | startline, |
int | length, | ||
uint8_t * | buffer, | ||
int | screen | ||
) |
Reads multi lines(400 x N bits = 50 x N bytes) from a screen.
Reads multi lines( (16 + 400) x N bits = 52 x N bytes) from a screen.
- Parameters:
-
startline starting line number(1-240) length number of line to read *buffer pointer to buffer screen screen to read from(0 or 1)
Definition at line 129 of file AkiSpiLcd.cpp.
void ramReadSingleLine | ( | int | line, |
uint8_t * | buffer, | ||
int | screen | ||
) |
Reads single line (400 bits = 50 bytes) from a screen and writes into buffer.
Reads single line (16 + 400 bits = 52 bytes) from a screen.
- Parameters:
-
line line number(1-240) buffer pointer to buffer(50 bytes) screen screen to read from(0 or 1)
Definition at line 114 of file AkiSpiLcd.cpp.
void ramWriteMultiLine | ( | int | startline, |
int | length, | ||
uint8_t * | data, | ||
int | screen | ||
) |
Writes multi lines(400 x N bits = 50 x N bytes) into a screen.
- Parameters:
-
startline starting line number(1-240) length number of line to read *data pointer to data to write screen screen to read from(0 or 1)
Definition at line 159 of file AkiSpiLcd.cpp.
void ramWriteSingleLine | ( | int | line, |
uint8_t * | data, | ||
int | screen | ||
) |
Writes single line (400 bits = 50 bytes) into a screen.
- Parameters:
-
line line number(1-240) *data pointer to data to write(50 bytes) screen screen to read from(0 or 1)
Definition at line 144 of file AkiSpiLcd.cpp.
Generated on Thu Jul 14 2022 22:22:59 by
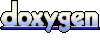