1
Dependencies: QEI2 chair_BNO055 PID Watchdog VL53L1X_Filter ros_lib_kinetic
Dependents: wheelchairControlSumer2019
statistics.cpp
00001 00002 #include "statistics.h" 00003 #include "mbed.h" 00004 00005 /************************************************************************** 00006 * This function initializes the private members of the class Statistics * 00007 **************************************************************************/ 00008 statistics::statistics(int* Input, int dataLengthIn, int firstDataPointIn){ 00009 data = Input; 00010 dataLength = dataLengthIn; 00011 firstDataPoint = firstDataPointIn; 00012 } 00013 00014 /************************************************************************** 00015 * This function returns the mean(average) of an array. * 00016 **************************************************************************/ 00017 double statistics::mean(){ 00018 double sum; 00019 for(int i = firstDataPoint; i < (firstDataPoint + dataLength); ++i) 00020 { 00021 sum += data[i]; 00022 } 00023 00024 double average = sum/dataLength; 00025 return average; 00026 } 00027 00028 /************************************************************************** 00029 * This function returns the standard deviation of an array. This is * 00030 * used to determine whether the data is valid or not. * 00031 **************************************************************************/ 00032 double statistics::stdev(){ 00033 double sum = 0.0, mean, standardDeviation = 0.0; 00034 printf("The length of the array is %d\n", dataLength); 00035 //double num = (pow(2.0,2.0)); 00036 //printf("2^2 is %f\n", num); 00037 //int i; 00038 00039 for(int i = firstDataPoint; i < (firstDataPoint + dataLength); ++i) 00040 { 00041 sum += data[i]; 00042 printf("%d\n", data[i]); 00043 } 00044 00045 mean = sum/dataLength; 00046 00047 for(int i = firstDataPoint; i < (firstDataPoint + dataLength); ++i) { 00048 //standardDeviation += pow(data[i] - mean, 2); 00049 standardDeviation += (data[i] - mean)*(data[i] - mean); 00050 } 00051 00052 return sqrt(standardDeviation / dataLength); 00053 }
Generated on Sun Jul 17 2022 02:37:02 by
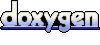